Question
due is today.can you just complete following code only line Lines 140, 151, 158. THANK YOU SO MUCH. A* In this assignment we are going
due is today.can you just complete following code only line Lines 140, 151, 158. THANK YOU SO MUCH.
A*
In this assignment we are going to implement A*. Remember, A* is exactly the same as dijkstra with the only difference that when we add the cost to the queue, we add the heuristic value to the cost of the node.
We will use Manhattan distance heuristic for this assignment, I have included the function for you so you only need to call it.
The algorithm is mostly implemented for you with only few lines for you to fill, I have provided comments above the lines.
You need to fill the following three lines
Lines 140, 151, 158
#code
class PriorityQueue: def __init__(self): self.heap = [] def get_parent(self, node_idx): return (node_idx-1) // 2 def get_left_child(self, node_idx): return node_idx * 2 + 1 def get_right_child(self, node_idx): return node_idx * 2 + 2
def sift_down(self, node_idx): left_idx = self.get_left_child(node_idx) right_idx = self.get_right_child(node_idx) smallest_idx = node_idx if left_idx < len(self.heap) and self.heap[left_idx] < self.heap[smallest_idx]: smallest_idx = left_idx if right_idx < len(self.heap) and self.heap[right_idx] < self.heap[smallest_idx]: smallest_idx = right_idx if smallest_idx != node_idx: self.heap[node_idx], self.heap[smallest_idx] = self.heap[smallest_idx], self.heap[node_idx] return self.sift_down(smallest_idx) def sift_up(self, node_idx): if node_idx == 0: return root_idx = self.get_parent(node_idx) if self.heap[node_idx] < self.heap[root_idx]: self.heap[node_idx], self.heap[root_idx] = self.heap[root_idx], self.heap[node_idx] return self.sift_up(root_idx) """ Extracts the minimum value from the priority queue """ def extract_min(self): last = len(self.heap)-1 self.heap[0], self.heap[last] = self.heap[last], self.heap[0] extract = self.heap.pop() self.sift_down(0) return extract """ Adds a new value to the priority queue """ def append(self, value): self.heap.append(value) self.sift_up(len(self.heap) - 1) def is_empty(self): return len(self.heap) == 0
""" This function returns a tuple of (neighbor_cost, neighbor_cell) """ def get_neighbors(graph, node): def is_valid(node): return ( node[0] >= 0 and node[1] >= 0 and \ node[1] < len(graph) and node[0] < len(graph[0]) and \ graph[node[1]][node[0]] != -1 ) neighbors = [] for direction in [(0, 1), (0, -1), (1, 0), (-1, 0)]: neighbor_x, neighbor_y = [node[i] + direction[i] for i in range(2)] potential_neighbor = (neighbor_x, neighbor_y) if is_valid(potential_neighbor): neighbors.append((graph[neighbor_y][neighbor_x], potential_neighbor)) return neighbors
def manhattan_distance(node1, node2): return abs(node1[0] - node2[0]) + abs(node1[1] - node2[1])
def a_star(graph, start, goal): # initialize variables parent = {} # holds mapping between every node and it's parent in the path current_node = start closed_set = set() cost_dict = {} # initialize open queue open_queue = PriorityQueue() open_queue.append((manhattan_distance(start, goal), start)) # while loop setting current node while not open_queue.is_empty(): current_node_cost, current_node = open_queue.extract_min() """ The cost on the queue includes the heuristic value so we need to subtract it to get the real cost """ current_node_cost -= manhattan_distance(current_node, goal) """ Check if the node is on the closed set, if it's then do nothing """ if current_node in closed_set: continue
if current_node == goal: """ Return the cost """ path = [goal] node = goal while node != start: node = parent[node] path.append(node) path.reverse() return (cost_dict[goal], path) # iterate over neighbors of current node # and add to open queue if not already there or in closed for neighbor in get_neighbors(graph, current_node): neighbor_cost, neighbor_node = neighbor neighbor_heuristic_value = manhattan_distance(neighbor_node, goal) """ Now we need to calculate the cost to reach this neighbor via current_node remember, the cost is the cost to the current_node + the cost of the edge (neighbor cost) + heuristic value """ # fill the following line new_cost = # your code here if neighbor_node in closed_set: continue if neighbor_node in cost_dict and cost_dict[neighbor_node] <= new_cost: continue cost_dict[neighbor_node] = new_cost """ Store parent info """ # fill this line """ Now we need to add the new cost and the neighbor node to the queue, make a tuple of the new cost and the neighbor node """ # Fill the following line open_queue.append(()) closed_set.add(current_node) return None
Step by Step Solution
There are 3 Steps involved in it
Step: 1
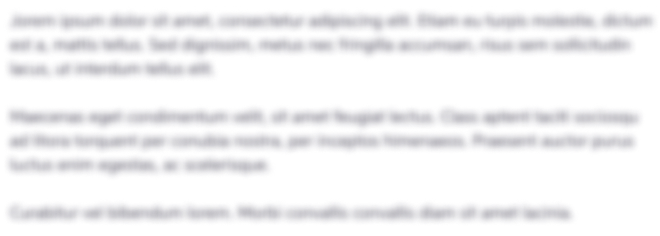
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started