Answered step by step
Verified Expert Solution
Question
1 Approved Answer
DynamicList.h #pragma once /*-- List.h --------------------------------------------------------------- This header file defines the data type List for processing lists. Basic operations are: Constructor Destructor Copy constructor Assignment

DynamicList.h
#pragma once
/*-- List.h ---------------------------------------------------------------
This header file defines the data type List for processing lists.
Basic operations are:
Constructor
Destructor
Copy constructor
Assignment operator
empty: Check if list is empty
insert: Insert an item
erase: Remove an item
display: Output the list
-------------------------------------------------------------------------*/
#include
using namespace std;
#ifndef LIST
#define LIST
typedef int ElementType;
class List
{
public:
/******** Function Members ********/
/***** Class constructor *****/
List(int maxSize = 1024);
/*----------------------------------------------------------------------
Construct a List object.
Precondition: maxSize is a positive integer with default value 1024.
Postcondition: An empty List object is constructed; myCapacity ==
maxSize (default value 1024); myArrayPtr points to a run-time
array with myCapacity as its capacity; and mySize is 0.
-----------------------------------------------------------------------*/
/***** Class destructor *****/
~List();
/*----------------------------------------------------------------------
Destroys a List object.
Precondition: The life of a List object is over.
Postcondition: The memory dynamically allocated by the constructor
for the array pointed to by myArrayPtr has been returned to
the heap.
------------------------------------------------------------------*/
/***** Copy constructor *****/
List(const List & origList);
/*----------------------------------------------------------------------
Construct a copy of a List object.
Precondition: A copy of origList is needed; origList is a const
reference parameter.
Postcondition: A copy of origList has been constructed.
-----------------------------------------------------------------------*/
/***** Assignment operator *****/
List & operator=(const List & origList);
/*----------------------------------------------------------------------
Assign a copy of a List object to the current object.
Precondition: none
Postcondition: A copy of origList has been assigned to this
object. A reference to this list is returned.
-----------------------------------------------------------------------*/
/***** empty operation *****/
bool empty() const;
/*----------------------------------------------------------------------
Check if a list is empty.
Precondition: None
Postcondition: true is returned if the list is empty,
false if not.
-----------------------------------------------------------------------*/
/***** insert and erase *****/
void insert(ElementType item, int pos);
/*----------------------------------------------------------------------
Insert a value into the list at a given position.
Precondition: item is the value to be inserted; there is room in
the array (mySize
0
Postcondition: item has been inserted into the list at the position
determined by pos (provided there is room and pos is a legal
position).
-----------------------------------------------------------------------*/
void erase(int pos);
/*----------------------------------------------------------------------
Remove a value from the list at a given position.
Precondition: The list is not empty and the position satisfies
0
Postcondition: element at the position determined by pos has been
removed (provided pos is a legal position).
----------------------------------------------------------------------*/
/***** output *****/
void display(ostream & out) const;
/*----------------------------------------------------------------------
Display a list.
Precondition: The ostream out is open.
Postcondition: The list represented by this List object has been
inserted into out.
-----------------------------------------------------------------------*/
int getSize() { return mySize; }
private:
/******** Data Members ********/
int mySize; // current size of list stored in array
int myCapacity; // capacity of array
ElementType * myArrayPtr; // pointer to dynamically-allocated array
}; //--- end of List class
//------ Prototype of output operator
ostream & operator
#endif
DynamicList.cpp
/*-- List.cpp------------------------------------------------------------
This file implements List member functions.
-------------------------------------------------------------------------*/
//#include
#include
using namespace std;
#include "DynamicList.h"
//--- Definition of class constructor
List::List(int maxSize)
: mySize(0), myCapacity(maxSize)
{
myArrayPtr = new ElementType[maxSize];
//assert(myArrayPtr != 0);
}
//--- Definition of class destructor
List::~List()
{
delete[] myArrayPtr;
}
//--- Definition of the copy constructor
List::List(const List & origList)
: mySize(origList.mySize), myCapacity(origList.myCapacity)
{
//--- Get new array for copy
myArrayPtr = new ElementType[myCapacity];
if (myArrayPtr != NULL) // check if memory available
//--- Copy origList's array into this new array
for (int i = 0; i
myArrayPtr[i] = origList.myArrayPtr[i];
else
{
cerr
exit(1);
}
}
//--- Definition of the assignment operator
List & List::operator=(const List & origList)
{
if (this != &origList) // check for list = list
{
//-- Allocate a new array if necessary
if (myCapacity != origList.myCapacity)
{
delete[] myArrayPtr;
myArrayPtr = new ElementType[myCapacity];
if (myArrayPtr == NULL) // check if memory available
{
cerr
exit(1);
}
}
mySize = origList.mySize;
myCapacity = origList.myCapacity;
//--- Copy origList's array into this new array
for (int i = 0; i
myArrayPtr[i] = origList.myArrayPtr[i];
}
return *this;
}
//--- Definition of empty()
bool List::empty() const
{
return mySize == 0;
}
//--- Definition of display()
void List::display(ostream & out) const
{
for (int i = 0; i
out
}
//--- Definition of output operator
ostream & operator
{
aList.display(out);
return out;
}
//--- Definition of insert()
void List::insert(ElementType item, int pos)
{
if (mySize == myCapacity)
{
cerr
"execution *** ";
exit(1);
}
if (pos mySize)
{
cerr
return;
}
// First shift array elements right to make room for item
for (int i = mySize; i > pos; i--)
myArrayPtr[i] = myArrayPtr[i - 1];
// Now insert item at position pos and increase list size
myArrayPtr[pos] = item;
mySize++;
}
//--- Definition of erase()
void List::erase(int pos)
{
if (mySize == 0)
{
cerr
return;
}
if (pos = mySize)
{
cerr
return;
}
// Shift array elements left to close the gap
for (int i = pos; i
//cout
myArrayPtr[i] = myArrayPtr[i + 1];
}
// Decrease list size
mySize--;
}
SourceDynamic.cpp
/* listtester.cpp is a program for testing class List. */
#include
using namespace std;
#include "DynamicList.h"
int main()
{
// Test the class constructor
List intList;
List llist;
cout
// Test empty() and output of empty list
if (intList.empty())
cout
cout
for (int i = 0; i
{
intList.insert(i, i / 2); // -- Insert i at position i/2
} //Test output
cout
//}
//cout
// Test the copy constructor
//List l= intList;
// //Test output
// cout
//
// cout
//
// l.insert(332,2);
// cout
// cout
//
for (int i = 0; i
{
llist.insert(i, i); // -- Insert i at position i/2
} //Test output
cout
cout
//Testing operator =
llist = intList; //cout
cout
cout
//cout
llist.insert(10, 2);
cout
cout
// Test destructor
{
List anotherlist;
for (int i = 0; i
anotherlist.insert(100 * i, i);
cout
cout
}
cout
//// Test erase
int index;
while (!intList.empty())
{
cout
cin >> index;
intList.erase(index);
cout
}
cout
1- (4 pts) A Compare function that returns true if two lists are equal and false otherwise. It compares *this to other. The prototype of the function s bool Compare (List &other). 2- (3 pts) Create a function to copy the elements from other to *this. Your function should be able to downsize or upsized the lists i.e. function should be able to copy a smaller list to a bigger one and vice versa. This function is similar to the copy assignment operator. The prototype is as follows: void CopyResize (List &) l3ps Funeion to rewense the orderofall ementsin 'thia list The prototype is void Reverse0 }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
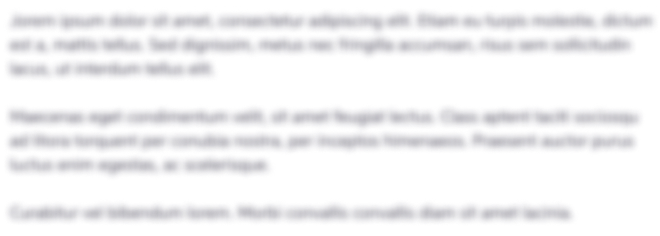
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started