Question
Each class should include: The fields listed above A parameterized constructor, no default constructors Getters/setters for each field An appropriate toString() method Planner Create a
Each class should include:
The fields listed above
A parameterized constructor, no default constructors
Getters/setters for each field
An appropriate toString() method
Planner
Create a program that stores six Contact objects (three PersonalContact, three BusinessContact). Initialize your array like so:
//create my contacts contacts[0] = new PersonalContact(...); contacts[1] = new BusinessContact(...); ...
Use the following data for your contact objects:
Type | Data |
Personal | Joe Smith, 33, 100 Evergreen Ave, Seattle, WA, 98999 |
Personal | Lawrence Williams, 45, 2000 1st St, Tacoma, WA, 98100 |
Personal | Rachel Garcia, 12, 12 Forest Drive, Los Angelos, CA, 99301 |
Business | Gregory Smith, 67, 360-888-7777, 360-555-6666 |
Business | Jerome Bradley, 18, 216-111-2222, 253-444-7777 |
Business | Susie Adams, 23, 253-333-7777, 425-666-9999 |
Planner Menu
Add the following menu that lets you interact with your contacts array:
Welcome to my planner! 1. Print planner contacts. 2. Print planner statistics. 3. Exit.
Option 1
When printing the planner contacts, you should loop over your array of contacts and print the toString() method of each Contact object:
Personal Contact: Joe Smith (33), 100 Evergreen Ave Seattle, WA, 98999 Business Contact: Susie Adams (23), business - 253-333-7777, cell - 425-666-9999 ...
Option 2
Print the following planner statistics. Your statistics should accurately reflect the data in your array (ie. if I change your contact array or any contact details, your planner statistics should still be accurate).
Number of contacts: 6 Number of personal contacts: 3 Number of business contacts: 3 Average contact age: 44
Note: Use the instanceof operator to identify which contacts are personal and which are business.
Option 3
After each user choice, the user should be presented with the menu repeatedly. If the user chooses option 3, the program will end.
Coding Guidelines
The output of your program should directly follow the instructions above
Make sure to use constants
Make sure to break your solution into several methods
Step by Step Solution
There are 3 Steps involved in it
Step: 1
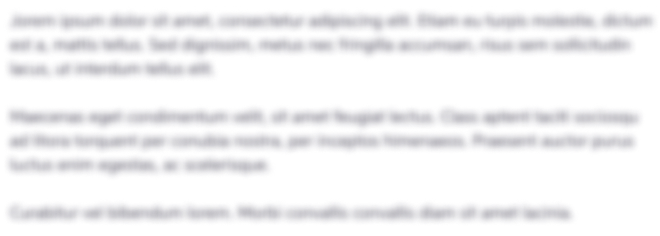
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started