Answered step by step
Verified Expert Solution
Question
1 Approved Answer
Edit the code below to make it into the snake game import pygame import random import time SCREEN_W, SCREEN_H = 750, 450 clock= pygame.time.Clock() class
Edit the code below to make it into the snake game import pygame import random import time SCREEN_W, SCREEN_H = 750, 450 clock= pygame.time.Clock() class Box: # Class holding info of box "character" def __init__(self, dim=36, color=(16, 132, 194)): self.dim = dim self.x, self.y = (SCREEN_W / 2) - (self.dim / 2), (SCREEN_H / 2) - (self.dim / 2) self.color = color def update_loc(self, x, y): self.x, self.y = x, y def surrounds(self, mouse_loc): # returns True if x and y are within box region, otherwise False mouse_x, mouse_y = mouse_loc return self.x - self.dim < mouse_x < self.x + self.dim and self.y - self.dim < mouse_y < self.y + self.dim def respawn(self): # resets box to center of screen self.x, self.y = (SCREEN_W / 2) - (self.dim / 2), (SCREEN_H / 2) - (self.dim / 2) def get_x(self): return self.x def increment_x(self, dx): self.x += dx def get_y(self): return self.y def increment_y(self, dy): self.y += dy def get_center(self): return self.x + (0.5 * self.dim), self.y + (0.5 * self.dim) def set_center(self, x=None, y=None): if x is not None: self.x = x - (0.5 * self.dim) if y is not None: self.y = y - (0.5 * self.dim) def get_dim(self): return self.dim def check_out_of_bounds(self): # checks if box is outside of screen, based on x and y (top-left corner coordinates of box) if self.x <= 0: self.x = 0 elif (self.x + self.dim) >= SCREEN_W: self.x = SCREEN_W - self.dim if self.y <= 0: self.y = 0 elif (self.y + self.dim) >= SCREEN_H: self.y = SCREEN_H - self.dim def get_rect_params(self): # returns tuple in format pygame often uses for rectangle parameters return self.x, self.y, self.dim, self.dim def get_color(self): return self.color def set_color(self, c): self.color = c def intersects_with(self, other_box): this_box = pygame.Rect(self.x, self.y, self.dim, self.dim) other_box = pygame.Rect(other_box.get_x(), other_box.get_y(), other_box.get_dim(), other_box.get_dim()) return this_box.colliderect(other_box) def game(): dx, dy = 12, 12 # setting speed for box movement length = 1 segments = [] big_box = Box(color=(16, 132, 194)) # creating main box object small_box = Box(dim=18, color=(239, 123, 61)) # creating smaller box for intersection example small_box.set_center(0.75*SCREEN_W, 0.5*SCREEN_H)# putting smaller box in different spot pygame.init() bg = pygame.display.set_mode((SCREEN_W, SCREEN_H), pygame.SRCALPHA, 32) clock = pygame.time.Clock() flags = {'up': False, 'down': False, 'mouse_loc': (), 'right': False, 'left': False, 'left_click': False, 'right_click': False, 'line_start': (), 'line_end': (), 'on_line': False} # creating dictionary of flags for ease of access mouse_loc, line_start, line_end = (), (), () # initalizing location variables text_label, text_val = 'Score', 0 # variables for scoreboard font = pygame.font.Font(None, 38) while True: clock.tick(30) # guarantees up to 30 FPS bg.fill((0, 0, 0)) # reset bg to black # checking for events for event in pygame.event.get(): if event.type == pygame.QUIT: exit() elif event.type == pygame.KEYDOWN: if event.key == pygame.K_w: flags['up'] = True elif event.key == pygame.K_s: flags['down'] = True elif event.key == pygame.K_a: flags['right'] = True elif event.key == pygame.K_d: flags['left'] = True elif event.key == pygame.K_SPACE: big_box.respawn() # SPACE lets the box go back to center, feel free to remove elif event.type == pygame.KEYUP: if event.key == pygame.K_w: flags['up'] = False elif event.key == pygame.K_s: flags['down'] = False elif event.key == pygame.K_a: flags['right'] = False elif event.key == pygame.K_d: flags['left'] = False elif event.type == pygame.MOUSEBUTTONDOWN: # only allows mouse functionality if left-clicking and doing so inside the box if event.button == 1 and big_box.surrounds(event.pos): flags['left_click'] = True # flag that allows for stuff that can only happen when left-clicking mouse_loc = event.pos # store mouse location for line drawing (current end point) line_start = big_box.get_center() # store box center for line drawing (starting point) flags['up'], flags['down'], flags['right'], flags['left'] = False, False, False, False # set flags elif event.button == 2 and big_box.surrounds(event.pos): flags['right_click'] = True flags['up'], flags['down'], flags['right'], flags['left'] = False, False, False, False elif event.type == pygame.MOUSEBUTTONUP: # only care here if left mouse button was clicked (held) previously if event.button == 1 and flags['left_click']: flags['left_click'] = False # no longer left-clicking since mouse button was released flags['on_line'] = True # presumably box is moving "on the line" after releasing mouse button line_end = event.pos # set final endpoint for the box as it moves along the path elif event.button == 1 and flags['right_click']: flags['right_click'] = False # allows for updating the line in real time while still left-clicked elif event.type == pygame.MOUSEMOTION and flags['left_click']: mouse_loc = event.pos # update mouse position due to movement # makes the big (blue) box white while it's intersecting with the smaller (orange) box if big_box.intersects_with(small_box): big_box.set_color((255, 255, 255)) text_val += 1 clock.tick(0) x, y = random.randint(18, SCREEN_W), random.randint(18, SCREEN_H) small_box.set_center(x, y) length += 1 else: big_box.set_color((16, 132, 194)) # If box is "on the line" (user clicked endpt and released), then calculate movement if flags['on_line']: # rate of change of x, y for movement rise, run = line_end[1] - line_start[1], line_end[0] - line_start[0] # the try/except stuff below just makes sure that we can work with 100% vertical/horizontal lines try: rise_sign = rise / abs(rise) except ZeroDivisionError: rise_sign = 0 try: run_sign = run / abs(run) except ZeroDivisionError: run_sign = 0 try: slope = (rise / run) except ZeroDivisionError: slope = 1 big_box.increment_x(dx * run_sign) if run_sign == 0: big_box.increment_y(dy * slope * rise_sign) else: big_box.increment_y(dy * slope * run_sign) # once we reach our goal x value, stop there if run_sign * big_box.get_center()[0] > run_sign * (line_end[0]): big_box.set_center(x=line_end[0]) flags['on_line'] = False # once we reach our goal y value, stop there if rise_sign * big_box.get_center()[1] > rise_sign * (line_end[1]): big_box.set_center(y=line_end[1]) flags['on_line'] = False # allow W/S key to move only if the box isn't moving "on the line" elif not flags['left_click'] : big_box.increment_y(flags['up'] * -dy + flags['down'] * dy) big_box.increment_x(flags['right'] * -dx + flags['left'] * dx) # move box back to 100% in the screen movement caused it to leave bounds if big_box.check_out_of_bounds() == False: bg = pygame.display.set_mode((SCREEN_W, SCREEN_H), pygame.SRCALPHA, 0) text_label= 'GAME OVER' font = pygame.font.Font(None, 40) pygame.display.update() # only draw line while left mouse button is pressed if flags['left_click']: pygame.draw.line(bg, (16, 132, 194), line_start, mouse_loc, width=2) elif flags['right_click']: big_box.dim += 6 score_surf = font.render(f'{text_label}: {text_val}', True, (230, 230, 230)) pygame.draw.rect(bg, big_box.get_color(), big_box.get_rect_params()) # print(big_box.dim) pygame.draw.rect(bg, small_box.get_color(), small_box.get_rect_params()) bg.blit(score_surf, (((SCREEN_W / 2) - (score_surf.get_width() / 2)), 10)) # draws text in top center of screen pygame.display.update() def main(): game() if __name__ == '__main__': main()
Step by Step Solution
There are 3 Steps involved in it
Step: 1
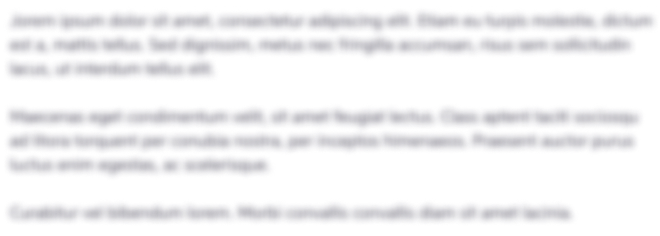
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started