Question
Edit the comments in this Java code so that they would show up when the javadoc index.html is generated. None of my methods and constructors
Edit the comments in this Java code so that they would show up when the javadoc index.html is generated. None of my methods and constructors are showing up.
Also, add code that would allow a student to only be enrolled in a MAX of 5 classes at a time.
//////////////////////////////////////////
public class Homework5 { static Roster roster = new Roster(); public static void main(String[] args) { addStudent(); displayAllStudents(); lookupStudent("11114"); deleteStudent("11113"); addCourses(); changeGrade("11112", "Math161", 80); dropCourse("11111", "Math161"); dropCoursesBelow("Math161", 65); displayCourses("11112"); displayAllStudents(); displayStudents("Math161"); displayCourseAverage("CS146"); }
// add students to the roster static void addStudent() { roster.addStudent(new Student("11111", "Jon", "Benson")); roster.addStudent(new Student("11112", "Erick", "Hooper")); roster.addStudent(new Student("11113", "Sam", "Shultz")); roster.addStudent(new Student("11114", "Trent", "Black")); roster.addStudent(new Student("11115", "Michell", "Waters")); roster.addStudent(new Student("11116", "Kevin", "Johnson")); }
// diaplay all students in the roster static void displayAllStudents() { roster.displayAllStudents(); }
// lookup a student in the roster static void lookupStudent(String id) { Student student = roster.find(id); if (student != null) { System.out.println(id + " found " + student); } else { System.out.println(id + " not found"); } }
// delete a in the roster static void deleteStudent(String id) { roster.deleteStudent(id); }
// add courses to the roster static void addCourses() { roster.addCourse("11111", new Course("CS116", 80)); roster.addCourse("11111", new Course("Math161", 90)); roster.addCourse("11112", new Course("Math161", 70)); roster.addCourse("11112", new Course("CS146", 90)); roster.addCourse("11112", new Course("CS105", 85)); roster.addCourse("11113", new Course("CS216", 90)); roster.addCourse("11114", new Course("CIS255", 75)); roster.addCourse("11114", new Course("CS216", 80)); roster.addCourse("11114", new Course("Math161", 60)); roster.addCourse("11114", new Course("COMM105", 90)); }
// display students enrolled in a given course id static void displayStudents(String courseId) { roster.displayStudents(courseId); }
// display courses taken by a student static void displayCourses(String id) { roster.displayCourses(id); }
// display the average grade for a student static void displayCourseAverage(String courseId) { roster.displayCourseAverage(courseId); }
// display the average grade for a student static void dropCoursesBelow(String id, int grade) { roster.dropCoursesBelow(id, grade); }
// drop a course from a student static void dropCourse(String id, String courseId) { roster.dropCourse(id, courseId); }
// change the grade for a student static void changeGrade(String id, String courseId, int grade) { roster.changeGrade(id, courseId, grade); } }
import java.util.ArrayList; import java.util.List;
/** storing the student details */ class Student implements Comparable {
String id; String firstName; String lastName; /** making an arraylist object of clas course for each of the student */ List courses = new ArrayList<>();
/** constructor for storing student information */ Student(String id, String fName, String lName) { this.id = id; this.firstName = fName; this.lastName = lName; }
@Override /** used for output the object details */ public String toString() { return "Student: " + id + " " + firstName + " " + lastName; }
/** comparing the student id */ public int compareTo(Student st) { int val = this.id.compareTo(st.id); return val < 0 ? -1 : val > 0 ? 1 : (this.firstName + this.lastName).compareTo(st.firstName + st.lastName); }
/** returning id of student */ public String getId() { return id; }
/** returning first name of student */ public String getFirstName() { return firstName; }
/** returning last name of student */ public String getLastName() { return lastName; }
/** adding course to the student */ public void addCourse(Course course) { courses.add(course); }
/** this function taking course id as an input and return curse object */ public Course getCourse(String courseid) { Course course = null; for (int i = 0; i < courses.size(); i++) { if (courses.get(i).getId().equals(courseid)) {//comparing course id course = courses.get(i); break; } } return course; }
/** this function taking course id as an input and droping course from the particular student object */ public void dropCourse(String courseId) { Course course = getCourse(courseId); if (course != null) { courses.remove(course); System.out.println("Course dropped for student: " + id + " " + course); } }
/** this function taking course id as an input and droping course from the particular student object which grade is lower then requier grade */ public void dropCourseBelow(String courseId, int grade) { Course course = getCourse(courseId); if (course != null) { if (course.grade < grade) { courses.remove(course); System.out.println(" Course dropped for student (low grade): " + id + " " + course); } } }
/** Display the all courses taken by a student given by an id */ public void displayCourses() { System.out.println("Courses for student " + id + ": "); for (int i = 0; i < courses.size(); i++) { System.out.println(" " + courses.get(i)); } }
/** display details of course by giving vourseid */ public void displayIfHaveCourse(String courseId) { Course course = getCourse(courseId); if (course != null) { System.out.println(" " + toString()); } } }
/** The details of coure and operation on course is done in class course */ class Course { String id; int grade; /** constructer to initialize course id and grade */ Course(String id, int grade) { this.id = id; this.grade = grade; }
@Override /** constructer to display course id and grade */ public String toString() { return "Course: " + id + " grade = " + grade; }
/** used to get course id */ public String getId() { return id; }
/** used to get course grade */ public int getGrade() { return grade; } }
import java.util.ArrayList; import java.util.List;
/** Class is used for creating student object act like as an driver class */ class Roster { Student root; int numStudents; List students = new ArrayList<>(); //array for students /** constucter for initialization */ public Roster() { root = null; numStudents = 0; }
/** for adding student */ public void addStudent(Student st) { students.add(st); }
/** for displaying all student information in the class */ public void displayAllStudents() { System.out.println("Displaying all students:"); for (int i = 0; i < students.size(); i++) { System.out.println(students.get(i)); } }
/** for finding a student given by his id */ public Student find(String id) { Student student = null; for (int i = 0; i < students.size(); i++) { if (students.get(i).getId().equals(id)) { //this line will check the id of a student student = students.get(i); break; } } return student; }
/** adding course to a student */ public void addCourse(String id, Course course) { Student student = find(id); if (student != null) { System.out.println("Course added for student: " + id + " " + course); student.addCourse(course);//this line will add course to given student id } }
/** display all the student having the course given by course id */ public void displayStudents(String courseId) { System.out.println("Displaying students with course: " + courseId); for (int i = 0; i < students.size(); i++) { students.get(i).displayIfHaveCourse(courseId); //this line will call to display } }
/** display all courses */ public void displayCourses(String id) { Student student = find(id); if (student != null) { student.displayCourses(); } }
/** for displaying course average student enrolled */ public void displayCourseAverage(String courseId) { int total = 0, count = 0; //variable for counting for (int i = 0; i < students.size(); i++) { Course course = students.get(i).getCourse(courseId); //select course if having course id if (course != null) { total += course.grade; count++; } } if (count == 0) { System.out.println("Average of course: " + courseId + " no student enrolled in this."); } else { System.out.println("Average of course: " + courseId + " " + (total / count)); } }
/** dropping a course from the courses */ public void dropCoursesBelow(String courseId, int grade) { System.out.println("Dropping course: " + courseId + " with grade below " + grade); for (int i = 0; i < students.size(); i++) { students.get(i).dropCourseBelow(courseId, grade); //this line will drop course } }
/** dropping all courses */ public void dropCourse(String id, String courseId) { Student student = find(id); if (student != null) { student.dropCourse(courseId); } }
/** change the grade of a perticular student */ public void changeGrade(String id, String courseid, int grade) { Student student = find(id); if (student != null) { Course course = student.getCourse(courseid); if (course != null) { course.grade = grade; //changing grade System.out.println("Grade changed for student: " + id + " " + course); } } }
/** delete the all information of a perticular student */ public void deleteStudent(String id) { Student student = find(id); String msg = "Deleting student: " + id + " => "; if (student == null) { System.out.println(msg + "Student doesn't exist"); } else { students.remove(student); //function call for removing System.out.println(msg + "Deleted"); } } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
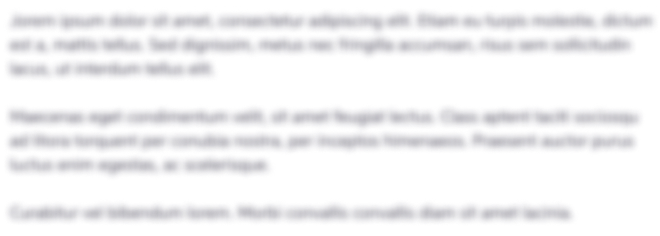
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started