Question
Edit this calculator program by altering the file format from ASCII to binary. You should save all the contents of the structure array containing the
Edit this calculator program by altering the file format from ASCII to binary. You should save all the contents of the structure array containing the calculations. Once user enters N to end the program, your program should then ask (with a Y/N) if the user wants to see the previous calculations. If user enters Y then retrieve all the calculations from the binary file and display all the calculations on display console. The display should have a number for each calculation in a separate line. For example:
- 10 8 = 2
- 24 / 5 = 4.8
Must use getline for calculation inputs.
Here is the code:
#include
#include
#include
using namespace std;
//Calculation struct with three variables, two operands and one operator
struct Calculation{
double f;
double r;
char o;
};
//calculate function, this function will be used for calculation
double calculate(double oprnd1, double oprnd2, char oprtr){
double result;
switch(oprtr){
case '+':
result = oprnd1+oprnd2;
break;
case '-':
result = oprnd1-oprnd2;
break;
case '*':
result = oprnd1*oprnd2;
break;
case '/':
result = oprnd1/oprnd2;
break;
}
return result;
}
double handleSign(double val,char ch){
switch (ch)
{
case '+':
return 1 * val;
case '-':
return (-1) * val;
default:
return val;
}
}
//takeUserChoice function, this function ask user if he/she wants to continue or exit
char takeUserChoice(){
char choice;
cout"do>
cin>>choice;
return choice;
}
//save calculation function, this function will store the three calculation in the file.
void saveCalculations(Calculation *arr){
ofstream MyFile;
MyFile.open("CalculatorOutput.txt",ios::app);
if(!MyFile){
cout"can't>
}else{
for(int i=0;i
int result = calculate(arr[i].f,arr[i].r,arr[i].o);//find the result of the calculation using calculate function
MyFile">">
}
}
}
//takeinput function, this function take calculation input from the user and store them in the passed array
void takeInput(Calculation *myCalculationArray){
fflush(stdin);//need to clear buffer when taking input using getline
int q = 0;
while(q
string inp=" ",="" first,="">
bool is_valid = true;
char symbol,num1Sign = '+',num2Sign = '+';
double c,num1 = 0,num2 = 0,decimal1 = 0, decimal2 = 0;
int decimalFound = 0,operatorfound = 0,dividend = 10;
cout"enter>
getline(cin,inp);//taking input
//here we filter the input string and extract both operands and operator
for(int i = 0; i();>
char ch = inp[i];
if(ch != '.' && (ch '9') && !(ch =='+'||ch =='-'||ch =='*'||ch =='/' || ch == ' ')){
is_valid=false;
}
if(decimalFound == 1 && (ch == '.'|| ch == ' ')){
is_valid=false;
}else if(decimalFound == 0 && ch == '.'){
decimalFound = 1;
}
else if(ch =='+'||ch =='-'||ch =='*'||ch =='/'){
if(i==0){
if(ch =='*'||ch =='/'){
is_valid=false;
}else{
num1Sign = ch;
}
}else if(operatorfound==1){
if(ch =='*'||ch =='/'||ch=='+'){
is_valid=false;
}else{
num2Sign = ch;
}
}else{
operatorfound=1;
decimalFound=0;
dividend = 10;
symbol = ch;
}
}else if(ch!=' '){
if(operatorfound==0){
if(decimalFound==0){
num1 = num1 * 10 + ch - '0';
}else{
float temp = ch-'0';
decimal1 = decimal1 + temp/dividend;
dividend*=10;
}
}else{
if(decimalFound==0){
num2 = num2 * 10 + ch - '0';
}else{
float temp = ch-'0';
decimal2 = decimal2 + temp/dividend;
dividend*=10;
}
}
}
if(operatorfound == 0 && decimal1){
decimalFound=0;
}else if(num2&&decimalFound==0&&ch==' '){
is_valid=true;
}
}
if(is_valid){
num1 = num1 + decimal1;
num2 = num2 + decimal2;
num1 = handleSign(num1,num1Sign);
num2 = handleSign(num2,num2Sign);
c = calculate(num1,num2,symbol);
cout">">">" ";>
myCalculationArray[q] = (Calculation){num1,num2,symbol};
q++;
}else{
cout"input>
}
fflush(stdin);//need to clear buffer when taking input using getline
}
}
int main(){
//declaring Claculation array of size 3;
Calculation myCalculationArray[3];
char userChoice;
takeInput(myCalculationArray);
saveCalculations(myCalculationArray);
userChoice = takeUserChoice();
//we will keep user in loop until he/she wants to exit.
while(true){
if(userChoice=='Y' || userChoice == 'y'){
takeInput(myCalculationArray);
saveCalculations(myCalculationArray);
}else if(userChoice=='N' || userChoice == 'n'){
cout"thank>
break;
}else{
cout"enter>
}
userChoice = takeUserChoice();
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
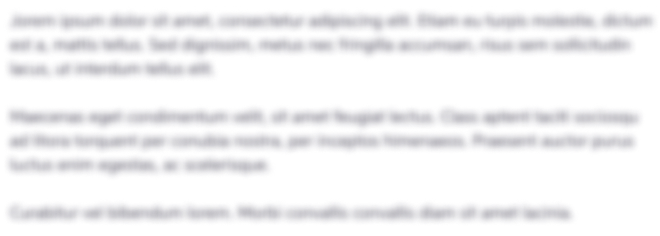
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started