Question
Edit this code We have 2 motors the problem is when operating a weak electricity arrives it should be a 6v each motor because i
Edit this code
We have 2 motors the problem is when operating a weak electricity arrives
it should be a 6v each motor because i used 12v source
and the decrease and increase in the motors are slow it should be faster
and the motors must be controlled separately
PIC16F887 & MOTOR DRIVER L298N
// 2 Motors control with NEC IR remote control CCS C code // Used MCU: PIC16F887 // Internal oscillator used @ 8MHz // Used remote control: Car MP3 IR remote control // PWM1 and PWM2 modules are used to control motor 1 and motor 2 speeds respectively #include <16F887.h> #fuses NOMCLR NOBROWNOUT NOLVP INTRC_IO #use delay(clock = 8MHz) short nec_ok = 0, repeated = 0, m1_dir = 0, m2_dir = 0; unsigned int8 nec_state = 0, i, duty1 = 0, duty2 = 0; unsigned int32 nec_code; #INT_EXT // External interrupt void ext_isr(void){ unsigned int16 time; if(nec_state != 0){ time = get_timer1(); // Store Timer1 value set_timer1(0); // Reset Timer1 } switch(nec_state){ case 0 : // Start receiving IR data (we're at the beginning of 9ms pulse) setup_timer_1( T1_INTERNAL | T1_DIV_BY_2 ); // Enable Timer1 module with internal clock source and prescaler = 2 set_timer1(0); // Reset Timer1 value nec_state = 1; // Next state: end of 9ms pulse (start of 4.5ms space) i = 0; ext_int_edge( L_TO_H ); // Toggle external interrupt edge break; case 1 : // End of 9ms pulse if((time > 9500) || (time < 8500)){ // Invalid interval ==> stop decoding and reset nec_state = 0; // Reset decoding process setup_timer_1(T1_DISABLED); // Stop Timer1 module } else nec_state = 2; // Next state: end of 4.5ms space (start of 562s pulse) ext_int_edge( H_TO_L ); // Toggle external interrupt edge break; case 2 : // End of 4.5ms space if((time > 5000) || (time < 1500)){ // Invalid interval ==> stop decoding and reset nec_state = 0; // Reset decoding process setup_timer_1(T1_DISABLED); // Stop Timer1 module break; } nec_state = 3; // Next state: end of 562s pulse (start of 562s or 1687s space) if(time < 3000) // Check if previous code is repeated repeated = 1; ext_int_edge( L_TO_H ); // Toggle external interrupt edge break; case 3 : // End of 562s pulse if((time > 700) || (time < 400)){ // Invalid interval ==> stop decoding and reset nec_state = 0; // Reset decoding process setup_timer_1(T1_DISABLED); // Disable Timer1 module } else{ // Check if the repeated code is for buttons 2, 3, 5 or 6 if(repeated && (nec_code == 0x00FF629D || nec_code == 0x00FFE21D || nec_code == 0x00FF02FD || nec_code == 0x00FFC23D)){ repeated = 0; nec_ok = 1; // Decoding process is finished with success disable_interrupts(INT_EXT); // Disable the external interrupt break; } nec_state = 4; // Next state: end of 562s or 1687s space ext_int_edge( H_TO_L ); // Toggle external interrupt edge break; } case 4 : // End of 562s or 1687s space if((time > 1800) || (time < 400)){ // Invalid interval ==> stop decoding and reset nec_state = 0; // Reset decoding process setup_timer_1(T1_DISABLED); // Disable Timer1 module break; } if( time > 1000) // If space width > 1ms (short space) bit_set(nec_code, (31 - i)); // Write 1 to bit (31 - i) else // If space width < 1ms (long space) bit_clear(nec_code, (31 - i)); // Write 0 to bit (31 - i) i++; if(i > 31){ // If all bits are received nec_ok = 1; // Decoding process is finished with success disable_interrupts(INT_EXT); // Disable the external interrupt } nec_state = 3; // Next state: end of 562s pulse (start of 562s or 1687s space) ext_int_edge( L_TO_H ); // Toggle external interrupt edge } } #INT_TIMER1 // Timer1 interrupt (used for time out) void timer1_isr(void){ nec_state = 0; // Reset decoding process ext_int_edge( H_TO_L ); // External interrupt edge from high to low setup_timer_1(T1_DISABLED); // Disable Timer1 module clear_interrupt(INT_TIMER1); // Clear Timer1 interrupt flag bit } void main(){ setup_oscillator(OSC_8MHZ); // Set internal oscillator to 8MHz output_d(0); enable_interrupts(GLOBAL); // Enable global interrupts enable_interrupts(INT_EXT_H2L); // Enable external interrupt clear_interrupt(INT_TIMER1); // Clear Timer1 interrupt flag bit enable_interrupts(INT_TIMER1); // Enable Timer1 interrupt setup_timer_2(T2_DIV_BY_16, 255, 1); // Set PWM frequency to 488Hz setup_ccp1(CCP_PWM); // Configure CCP1 module as PWM setup_ccp2(CCP_PWM); // Configure CCP2 module as PWM set_pwm1_duty(0); // Set PWM1 duty sycle set_pwm2_duty(0); // Set PWM2 duty sycle while(TRUE){ if(nec_ok){ // If the MCU receives a message from the remote control nec_ok = 0; // Reset decoding process nec_state = 0; setup_timer_1(T1_DISABLED); // Disable Timer1 module // Motor 1 if(nec_code == 0x00FFE21D && m1_dir){ // If button 1 is pressed (toggle rotation direction of motor 1) m1_dir = 0; nec_code = 0; output_high(PIN_D0); output_low(PIN_D1); } if(nec_code == 0x00FFE21D && !m1_dir){ // If button 1 is pressed (toggle rotation direction of motor 1) m1_dir = 1; output_low(PIN_D0); output_high(PIN_D1); } if(nec_code == 0x00FFE21D && duty1 < 255){ // If button 3 is pressed (increase motor 1 speed) duty1++; set_pwm1_duty(duty1); } if(nec_code == 0x00FF629D && duty1 > 0){ // If button 2 is pressed (decrease motor 1 speed) duty1--; set_pwm1_duty(duty1); } // Motor 2 if(nec_code == 0x00FF22DD && m2_dir){ // If button 4 is pressed (toggle rotation direction of motor 2) m2_dir = 0; nec_code = 0; output_high(PIN_D2); output_low(PIN_D3); } if(nec_code == 0x00FF22DD && !m2_dir){ // If button 4 is pressed (toggle rotation direction of motor 2) m2_dir = 1; output_low(PIN_D2); output_high(PIN_D3); } if(nec_code == 0x00FFC23D && duty2 < 255){ // If button 6 is pressed (increase motor speed) duty2++; set_pwm2_duty(duty2); } if(nec_code == 0x00FF02FD && duty2 > 0){ // If button 5 is pressed (decrease motor 2 speed) duty2--; set_pwm2_duty(duty2); } enable_interrupts(INT_EXT_H2L); // Enable external interrupt } } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
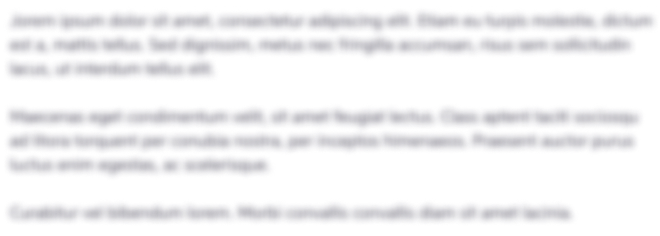
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started