Question
Employee C++ Program changes This project's goal is to take a previously written program and make changes to it. Here is my .cpp File https://pastebin.com/0EFbpx5G
Employee C++ Program changes
This project's goal is to take a previously written program and make changes to it.
Here is my .cpp File
https://pastebin.com/0EFbpx5G
Here is my .h File
https://pastebin.com/73XvRpPk
The code that you wrote for last week's project should only work for a specific set of employee data. Given what we knew last week, it would have been difficult to do any better. It is harder to write a program that can handle any number of employees, in any order. Now that we have studied polymorphism, we can begin to make our payroll program more general. This week's program should handle employee objects in any order. The most important thing we will do is enable polymorphism in our Employee class hierarchy. We will rename Employee to HourlyEmployee, and then create two new classes, Employee and SalariedEmployee, as depicted below.
Note the following changes to the Employee project:
1. The Employee abstract class establishes the interface for all classes that derive from it, and store common data operations that apply to all derived classes.
2. The public Employee constructor has 4 parameters representing the common data. The derived class constructors pass the common data to the Employee class constructor in an initializer list, before storing its own specific data in its own constructor.
3. The functions write, calcPay, printCheck are all pure virtual functions in Employee, because they must be overridden in the derived classes. They also contain code that is common to the derived classes (pure virtual functions do not have to have empty bodies; they constitute a good place to put shared code).
4. readData is a private, pure virtual function called by Employee::read (see below).
5. Base classes at the top of a hierarchy must always declare their destructors to be virtual, and must have a (usually empty) function body. We signify this by writing virtual ~Employee() = default;.
6. The write functions in the derived classes explicitly call Employee::write to write the common output before they write their specific output.
7. In similar fashion, printCheck and readData explicitly call their Employee counterparts to perform the common operations for all employees.
8. calcPay works as follows in the derived classes (Employee::calcPay needs no body):
8 A. Hourly Employees: An hourly employee's gross pay is calculated by multiplying the hours worked by their hourly wage. Be sure to give time-and-a-half for overtime (anything over 40 hours). To compute the net pay, deduct 20% of the gross for Federal income tax, and 7.5% of the gross for state income tax.
8 B. Salaried Employees: A salaried employee's gross pay is just their weekly salary value. To compute the net pay for a salaried employee, deduct 20% of the gross for Federal income tax, 7.5% of the gross for state income tax, and 5.24% for benefits.
9. Employee::read creates an empty Employee object via a private default constructor, for example, Employee* temp = new Employee();. It then reads the common data from the file stream provided and directly assigns data to the common data members. It then calls temp->readData(theFile), which sets the remaining derived data members. Finally, Employee::read returns temp. There is no public default constructor.
The Driver
Your driver will provide the same set of options as in the previous project. However, your driver code should be somewhat simpler because you will now create an array of Employee pointers, and store the pointers to your objects in this array. The code to do this will look something like:
payroll[0] = new Hourly(1, "H. Potter", "Privet Drive", "201-9090", 40, 12.00); payroll[1] = new Salaried(2, "A. Dumbledore", "Hogewarts", "803-1230", 1200); payroll[2] = new Hourly(3, "R. Weasley", "The Burrow", "892-2000", 40, 10.00); payroll[3] = new Salaried(4, "R. Hagrid", "Hogwarts", "910-8765", 1000);
You can then use a simple for loop to save each object's data to the file.
When you run option 2, your program should create a new array of Employee pointers. Your program should be able to read the data from the file without assumming that the objects in the file are in any particular order. To do this, you have to have some code that determines what kind of object is represented by the next set of data in the file. Then
Create an object of the required kind, Hourly or Salaried.
Save the pointer to this object in the new array of Employee pointers.
Have the object read its data from the file.
Repeat this for each set of data in the file.
Employee getEmployeeNumber getName getAddress getPhone Employee read(ifstream&) virtual void write(ofstream&) virtual double calcPay 0 virtual printCheck 0 virtual void readData -0 virtual Employee() Hourly Salaried Employee Employee getHours Worked getWeekly Salary getHourly Wage override write override write override calcPay override calcPay override printCheck override printCheck override readData override readData Employee getEmployeeNumber getName getAddress getPhone Employee read(ifstream&) virtual void write(ofstream&) virtual double calcPay 0 virtual printCheck 0 virtual void readData -0 virtual Employee() Hourly Salaried Employee Employee getHours Worked getWeekly Salary getHourly Wage override write override write override calcPay override calcPay override printCheck override printCheck override readData override readDataStep by Step Solution
There are 3 Steps involved in it
Step: 1
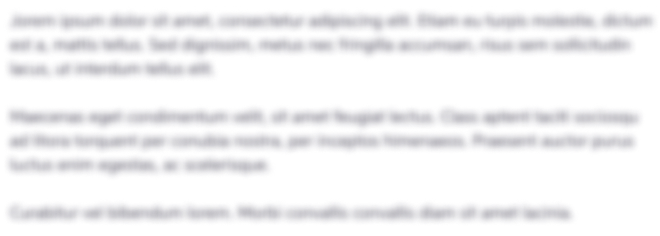
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started