Answered step by step
Verified Expert Solution
Question
1 Approved Answer
Encoding needs to be UTF-8. Please don't change method names or their types. import java.util.Scanner; import java.util.Random; public class Battleship { /** * This method
Encoding needs to be UTF-8. Please don't change method names or their types.
import java.util.Scanner; import java.util.Random; public class Battleship { /** * This method converts a String representing a base (or radix) 26 number into a decimal (or * base 10) number. The String representation of the base 26 number uses the letters of the * Latin alphabet to represent the 26 digits. That is, A represents 0, B represents 1, C * represents 2, ..., Y represents 24, and Z represents 25. * * A couple of examples: * BAAA = 1 * 26^3 + 0 * 26^2 + 0 * 26^1 + 0 * 26^0 = 17576 * ZERTY = 25 * 26^4 + 4 * 26^3 + 17 * 26^2 + 19 * 26^1 + 24 * 26^0 = 11506714 * "&\"" = -14747 * For this method: * - use Math.pow to calculate the powers of 26. * - don't assume that the input is in any particular case; use toUpperCase(). * - don't check that the input is only 'A' to 'Z'. * - calculate the value of each digit relative to 'A'. * - start from either the first or last character, and calculate the exponent based on the * index of each character. * * @param coord The coordinate value in base 26 as described above. * @return The numeric representation of the coordinate. */ public static int coordAlphaToNum(String coord) { //FIXME return -1; } /** * This method converts an int value into a base (or radix) 26 number, where the digits are * represented by the 26 letters of the Latin alphabet. That is, A represents 0, B represents 1, * C represents 2, ..., Y represents 24, and Z represents 25. * A couple of examples: 17576 is BAAA, 11506714 is ZERTY. * * The algorithm to convert an int to a String representing these base 26 numbers is as follows: * - Initialize res to the input integer * - The next digit is determined by calculating the remainder of res with respect to 26 * - Convert this next digit to a letter based on 'A' * - Set res to the integer division of res and 26 * - Repeat until res is 0 * * @param coord The integer value to covert into an alpha coordinate. * @return The alpha coordinate in base 26 as described above. If coord is negative, an empty * string is returned. */ public static String coordNumToAlpha(int coord) { //FIXME return ""; } /** * Prompts the user for an integer value, displaying the following: * "Enter the valName (min to max): " * Note: There should not be a new line terminating the prompt. valName should contain the * contents of the String referenced by the parameter valName. min and max should be the values * passed in the respective parameters. * * After prompting the user, the method will read an int from the console and consume an entire * line of input. If the value read is between min and max (inclusive), that value is returned. * Otherwise, "Invalid value." terminated by a new line is output and the user is prompted * again. * * @param sc The Scanner instance to read from System.in. * @param valName The name of the value for which the user is prompted. * @param min The minimum acceptable int value (inclusive). * @param min The maximum acceptable int value (inclusive). * @return Returns the value read from the user. */ public static int promptInt(Scanner sc, String valName, int min, int max) { //FIXME return -1; } /** * Prompts the user for an String value, displaying the following: * "Enter the valName (min to max): " * Note: There should not be a new line terminating the prompt. valName should contain the * contents of the String referenced by the parameter valName. min and max should be the values * passed in the respective parameters. * * After prompting the user, the method will read an entire line of input, trimming any trailing * or leading whitespace. If the value read is (lexicographically ignoring case) between min and * max (inclusive), that value is returned. Otherwise, "Invalid value." terminated by a new line * is output and the user is prompted again. * * @param sc The Scanner instance to read from System.in. * @param valName The name of the value for which the user is prompted. * @param min The minimum acceptable String value (inclusive). * @param min The maximum acceptable String value (inclusive). * @return Returns the value read from the user. */ public static String promptStr(Scanner sc, String valName, String min, String max) { //FIXME return ""; }
/** * This is the main method for the Battleship game. It consists of the main game and play again * loops with calls to the various supporting methods. When the program launches (prior to the * play again loop), a message of "Welcome to Battleship!", terminated by a newline, is * displayed. After the play again loop terminiates, a message of "Thanks for playing!", * terminated by a newline, is displayed. * * The Scanner object to read from System.in and the Random object with a seed of Config.SEED * will be created in the main method and used as arguments for the supporting methods as * required. */ public static void main(String[] args) { //FIXME } }
Interleaved input and output:
Welcome to Battleship! Enter the board height (1 to 99): 8 Enter the board width (1 to 675): 5 Would you like to play again? (y/n): Y Enter the board height (1 to 99): 200 Invalid value. Enter the board height (1 to 99): 99 Enter the board width (1 to 675): 675 Would you like to play again? (y/n): y Enter the board height (1 to 99): 3 Enter the board width (1 to 675): 200 Would you like to play again? (y/n): y Enter the board height (1 to 99): 1 Enter the board width (1 to 675): 1 Would you like to play again? (y/n): n Thanks for playing!
Config:
public class Config { /** * Minimum and maximum values used in the program */ public static final int MIN_WIDTH = 1; //Minimum number of columns public static final int MAX_WIDTH = 675; //Maximum number of columns public static final int MIN_HEIGHT = 1; //Minimum number of rows public static final int MAX_HEIGHT = 99; //Maximum number of rows public static final int MAX_COL_WIDTH = 3; //Maximum number of characters per column public static final int MIN_SHIPS = 1; //Minimum number of ships public static final int MAX_SHIPS = 9; //Maximum number of ships public static final int MIN_SHIP_LEN = 1; //Minimum ship length /** * Character values for displaying the different statuses of the game board cells. */ public static final char WATER_CHAR = '~'; // Water character (not yet targeted) public static final char HIT_CHAR = '*'; // Hit character public static final char MISS_CHAR = '@'; // Miss character /** * Constants for the random processes. */ public static final long SEED = 1234; // The random seed public static final int RAND_SHIP_TRIES = 20; // Maximum number of tries to place a ship }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
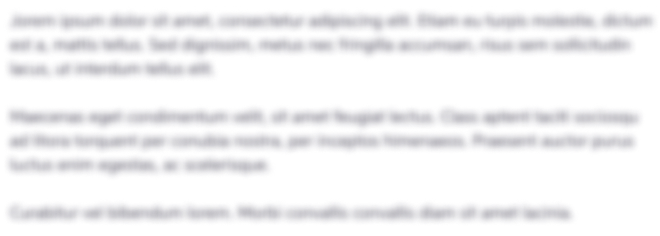
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started