Question
enhance my previous bank account application by changing the application to use Polymorphism. The assignment demonstrates your ability to use both virtual and pure functions
enhance my previous bank account application by changing the application to use Polymorphism. The assignment demonstrates your ability to use both virtual and pure functions from a parent "abstract" bankAccount Class.
All .cpp and .h files needed: testBankAccount.cpp, bankAccount.h, bankAccount.cpp, checkingAccount.h, checkingAccount.cpp ,savingsAccount.h, savingsAccount.cpp
Previous application to build off of:
Bankaccounttest.cpp:
//Test class
#include "savingsAccount.h"
#include "checkingAccount.h"
using namespace std;
int main() {
//Variables
int accountNumber = 27;
//Interest Rate: 0.08%
//Minimum Balance : $25
//Service Charge : $20
//Create 2 savings and checking accounts
checkingAccount bobAccount(accountNumber++, 1000, 0.08, 25, 20);
checkingAccount myraAccount(accountNumber++, 450, 0.08, 25, 20);
savingsAccount samyAccount(accountNumber++, 9300, 0.08);
savingsAccount ricoAccount(accountNumber++, 32, 0.08);
//Deposit values
bobAccount.deposit(1000);
myraAccount.deposit(2300);
samyAccount.deposit(800);
ricoAccount.deposit(500);
//Interset added
bobAccount.postInterest();
myraAccount.postInterest();
samyAccount.postInterest();
ricoAccount.postInterest();
//Display details
cout
bobAccount.print();
myraAccount.print();
samyAccount.print();
ricoAccount.print();
// write check and with draw
cout
bobAccount.writeCheck(250);
myraAccount.writeCheck(350);
samyAccount.withdraw(120);
ricoAccount.withdraw(290);
//Display details
cout
bobAccount.print();
myraAccount.print();
samyAccount.print();
ricoAccount.print();
cout
return 0;
bankaccount.cpp
//Implementation of bank account class
#include "bankAccount.h"
//Constructor
bankAccount::bankAccount(int accNum, double bal) {
setAccountNumber(accNum);
setBalance(bal);
}
//Getters
int bankAccount::getAccountNumber() {
return accountNumber;
}
double bankAccount::getBalance() {
return balance;
}
//Setters
void bankAccount::setAccountNumber(int accNum) {
if (accNum > 0) {
accountNumber = accNum;
}
}
void bankAccount::setBalance(double bal) {
if (bal > 0) {
balance = bal;
}
else
{
balance = 0;
}
}
//Withdraw from account
void bankAccount::withdraw(double amt) {
if (balance - amt >= 0) {
balance -= amt;
}
}
//Deposit into account
void bankAccount::deposit(double amt) {
if (amt >= 0) {
balance += amt;
}
}
//Display details of account
void bankAccount::print() {
cout
bankaccount.h
//Create a class bankAccount as super class
#ifndef BANK_ACCOUNT_H
#define BANK_ACCOUNT_H
#include
#include
#include
using namespace std;
class bankAccount {
//Member varaibles
private:
int accountNumber;
double balance;
//Member functions
public:
//Constructor
bankAccount(int, double);
//Getters
int getAccountNumber();
double getBalance();
//Setters
void setAccountNumber(int);
void setBalance(double);
//Withdraw from account
void withdraw(double);
//Deposit into account
void deposit(double);
//Display details of account
void print();
};
#endif // !BANK_ACCOUNT_H
Checkingaccount.cpp
//Implementation of checking account
#include "checkingAccount.h"
//Constructor
checkingAccount::checkingAccount(int accNum, double bal, double ir, double minBal, double servCharge) :bankAccount(accNum, bal) {
setMinimumBalance(minBal);
setInterestRate(ir);
setServiceCharge(servCharge);
}
//Getters and setters
double checkingAccount::getMinimumBalance() {
return minimumBalance;
}
void checkingAccount::setMinimumBalance(double amt) {
minimumBalance = amt;
}
double checkingAccount::getInterestRate() {
return interestRate;
}
void checkingAccount::setInterestRate(double ir) {
interestRate = ir;
}
double checkingAccount::getServiceCharge() {
return serviceCharge;
}
void checkingAccount::setServiceCharge(double amt) {
serviceCharge = amt;
}
//Calculate interest and add
void checkingAccount::postInterest() {
setBalance(getBalance() + getBalance() * (interestRate / 100));
}
//withdraw amount and check for service charge
void checkingAccount::writeCheck(double amt) {
withdraw(amt);
}
//With draw amount
void checkingAccount::withdraw(double amt) {
if (getBalance()
cout
}
else if (getBalance() - amt
if ((getBalance() - amt) - serviceCharge
cout
}
else {
setBalance(getBalance() - amt - serviceCharge);
}
}
else {
setBalance(getBalance() - amt);
}
}
//Display details
void checkingAccount::print() {
cout
}
Savingsaccount.h
//Create a savings account class
#ifndef SAVINGS_ACCOUNT_H
#define SAVINGS_ACCOUNT_H
#include "bankAccount.h"
class savingsAccount :public bankAccount {
//Member variable
private:
double interestRate;
//Member functions
public:
savingsAccount(int, double, double);
//Getters and setters
double getInterestRate();
void setInterestRate(double);
//Withdraw amount
void withdraw(double);
//Add interest
void postInterest();
//display account details
void print();
};
#endif // !SAVINGS_ACCOUNT_H
Checkingaccount.h
//Create a sub class checkingAccount
#ifndef CHECKING_ACCOUNT_H
#define CHECKING_ACCOUNT_H
#include "bankAccount.h"
class checkingAccount :public bankAccount {
//Member variables
private:
double interestRate, minimumBalance, serviceCharge;
//Member functions
public:
//Constructor
checkingAccount(int, double, double, double, double);
//Getters and setters
double getMinimumBalance();
void setMinimumBalance(double);
double getInterestRate();
void setInterestRate(double);
double getServiceCharge();
void setServiceCharge(double);
//Calculate interest and add
void postInterest();
//withdraw amount and check for service charge
void writeCheck(double);
//With draw amount
void withdraw(double);
//Display details
void print();
};
#endif // !CHECKING_ACCOUNT_H
Savingsaccount.cpp
//Implementation of savings account implementation
#include "savingsAccount.h"
savingsAccount::savingsAccount(int accNum, double bal, double ir) :bankAccount(accNum, bal) {
interestRate = ir;
}
//Getters and setters
double savingsAccount::getInterestRate() {
return interestRate;
}
void savingsAccount::setInterestRate(double ir) {
interestRate = ir;
}
//Withdraw amount
void savingsAccount::withdraw(double amt) {
if (amt > getBalance()) {
cout
}
else {
setBalance(getBalance() - amt);
}
}
//Add interest
void savingsAccount::postInterest() {
setBalance(getBalance() + getBalance() * (interestRate / 100));
}
//display account details
void savingsAccount::print() {
cout
WHAT to do:
bankAccount - The bankAccount class will be abstract in this assignment and will use virtual and pure virtual functions. Constructor: - sets account number - set account holder name - sets account balance Note: Print() - displays the account number and balance Derive class checkingAccount from base class bankAccount. The checkingAccount class should define and implement the following data and methods: checkingAccount \begin{tabular}{l|l} \hline Data & \\ interestRate & Methods \\ minimumBalance & getMinimumBalance \\ serviceCharge & setMinimumBalance \\ fee & getInterestRate \\ & setInterestRate \\ & getServiceCharge \\ & setServiceCharge \\ & postInterest \\ & writeCheck \\ & withdraw \\ & print \\ & createMonthlyStatement \\ \hline \end{tabular} Constructor: - sets account holder name - sets account number - sets account balance - sets minimum balance - set interest rate - sets service charge amount - sets amount of monthly fee NOTES: postInterest takes the interest amount based off of the total balance and adds it to the balance (balance + balance * interestRate) writeCheck calls withdraw withdraw checks to make sure there is enough money in the account first and reports a warning if not. If there is enough money to make the withdraw, it then checks to see if the balance will go below the minimum amount after the withdraw. If so, it checks to see if the balance will go below zero after the withdraw and the service charge is applied. Should this occur an error is reported. If not, the balance is adjust to reflect the withdraw and the service charge applied. createMonthlyStatement - this posts the interest (calls postInterest()) and deducts the monthly fee postInterest takes the interest amount based off of the total balance and adds it to the balance (balance + balance * interestRate) writeCheck calls withdraw withdraw checks to make sure there is enough money in the account first and reports a warning if not. If there is enough money to make the withdraw, it then checks to see if the balance will go below the minimum amount after the withdraw. If so, it checks to see if the balance will go below zero after the withdraw and the service charge is applied. Should this occur an error is reported. If not, the balance is adjust to reflect the withdraw and the service charge applied. createMonthlyStatement - this posts the interest (calls postInterest()) and deducts the monthly fee from the balance. Print uses the base class print () and then also prints the interest rate
Step by Step Solution
There are 3 Steps involved in it
Step: 1
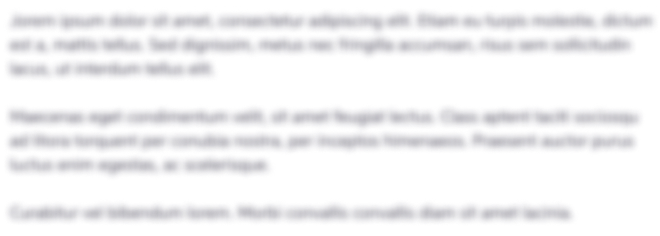
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started