Question
// Enter your name here // State the IDE that you use: Visual Studio or GCC #include #include #pragma warning(disable : 4996) // compiler directive
// Enter your name here // State the IDE that you use: Visual Studio or GCC #include
// Problem 1: initializeStrings (5 points) // Use pointer 'ptr' to traverse the 2D array of characters variable 'strings' (input from user in main()) and set all characters in each // array to a null terminator so that there is a 4 row and 50 column 2D array full of null terminators. // The null terminator '\0' is used to denote the end of a string. void initializeStrings(char strings[NUM_STRINGS][STRING_LENGTH]) { char* ptr = &strings[0][0]; // Enter the code below }
// Problem 2: printStrings (5 points) // Use pointer 'ptr' to traverse the 2D character array 'strings' and print each string.
// See the example outputs provided in the word document. Each string should be printed on a new line. void printStrings(char strings[NUM_STRINGS][STRING_LENGTH]) { char* ptr = &strings[0][0]; // Enter the code below }
// Problem 3: toUppercase (5 points) // Convert the string in the array 's' to an uppercase string. // Traverse the input using pointer 'p' and covert the character to an uppercase letter if it is lowercase. // You need to write down the code to check whether or not the character is lowercase. // HINT: Use a pointer to change the ASCII value of a character. // The ASCII value of 'a'= 97 and the ASCII value of 'A' = 65 so the difference between them is 32. void toUppercase(char strings[NUM_STRINGS][STRING_LENGTH]) { char* p = &strings[0][0]; // enter code here }
// Problem 4: reverseOneString (5 points) // Reverse the string s by using pointer. // Use pointer 'ptr' and 'temp' char to swap 1st char with last, then 2nd char with (last-1) and so on.. // Finally return pointer 'ptr' which points to start of the reversed string. // Hint: You might want to check if your logic works with even as well as odd length string. // You can write test code to print out the reversed string to check if your function works. (Don't include it in final submission) char* reverseOneString(char s[STRING_LENGTH]) { char temp; // not necessary to use this variable char* ptr = &s[0]; // pointer to start of string int len = strlen(s); // Enter the code below } // Problem 5: reverseStrings (5 points) // Reverse all the strings in 'strings[][]' // For each string in 'strings', use the reverseOneString() to reverse it. void reverseStrings(char strings[NUM_STRINGS][STRING_LENGTH]) { // Enter the code below } // Problem 6: wordMatch (5 points) // This function must be working as follows: // 1. Compare the strings in the 2D array with input string // 2. If one of them matches with input string, print "Match found" // 3. Else print "Match not found" // Example: strings array -> ["Hi", "Sun", "Devils"], input -> "Sun" => print "Match found" // Example: strings array -> ["Hi", "Sun", "Devils"], input -> "Hello" => print "Match not found"
void wordMatch(char strings[NUM_STRINGS][STRING_LENGTH], char input[STRING_LENGTH]) { char* p = &strings[0][0]; char* ip = input; int flag = 0; // enter code here } // Problem 7: concatStr (10 points) // This function must be working as follows: // 1. Concatenate the string in the array 'strings' together in a sentence. // 2. Store the string to the array 'result'. // 3. Print the string in the array 'result'. // The sample output is shown in document // NOTE: This is the function partially containing what you have implemented so far. // Initialize the array 'result' first before you store a string to it. // You may declare and use more pointers if needed. // Hint: You'll want to pay special attention '\0' characters void concatStr(char strings[NUM_STRINGS][STRING_LENGTH], char result[NUM_STRINGS * STRING_LENGTH]) { char* p_result = result; char* p_input = &strings[0][0]; // enter code here // 1. Initialize result // 2. Append characters in strings array to result using pointers // 3. Print the string in the variable 'result' using pointer. }
// You should study and understand how main() works. // *** DO NOT modify it in any way *** int main() { char strings[NUM_STRINGS][STRING_LENGTH]; // will store four strings each with a max length of 34 char strings_Copy[NUM_STRINGS][STRING_LENGTH]; int i; char input[STRING_LENGTH]; char result[NUM_STRINGS * STRING_LENGTH]; printf("CSE240 HW4: Pointers "); initializeStrings(strings); for (i = 0; i
//Question 3 printf(" Checking conversion to uppercase. "); toUppercase(strings);
printStrings(strings); //Question 4/5 printf(" Checking Reversing of Strings. "); reverseStrings(strings); printStrings(strings);
//Question 6 printf(" Enter a string to find a match: "); // prompt for string fgets(input, sizeof(input), stdin); // store input string input[strlen(input) - 1] = '\0'; // convert trailing ' ' char to '\0' (null terminator) // Word match function call wordMatch(strings, input);
//Question 7 printf(" The concatenated string is: "); concatStr(strings, result); printf(" "); return 0; }
output:
Step by Step Solution
There are 3 Steps involved in it
Step: 1
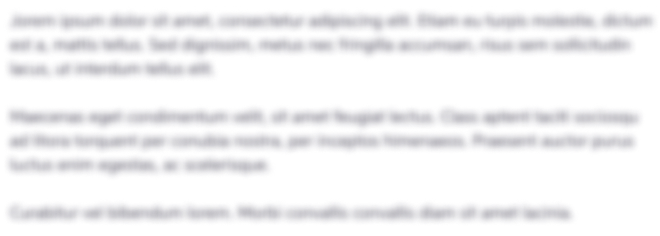
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started