Equals/hashCode/compareTo for HashSet and TreeSet elements. Consider the following object classes in Section 1.2:
-
Point2D, page 77, a point is identified by its coordinates (x, y)
-
Date, page 103, a Date is identified by (month, day, year)
-
String, page 80, a String is identified by its string contents
-
Counter, page 85, a Counter is identified by its name
-
Examine these object classes for their equals, hashCode, and compareTo, and determine if Point2D can be used for HashSet and separately if it can be used for TreeSet and then if Date can be used for its HashSet or TreeSet, and so on. Briefly explain your reasoning. Note that the same analysis holds for HashMap and TreeMap using the object as a key (values in Maps need no equals, etc.).
-
If the class doesn't have both an equals method and a hashCode method, for example Date.java on page 103, write equals and/or hashCode for it based on the identifying fields listed above, so that it ends up with both methods. After this, HashSet should be usable for all four object classes. ===================================================================================================================================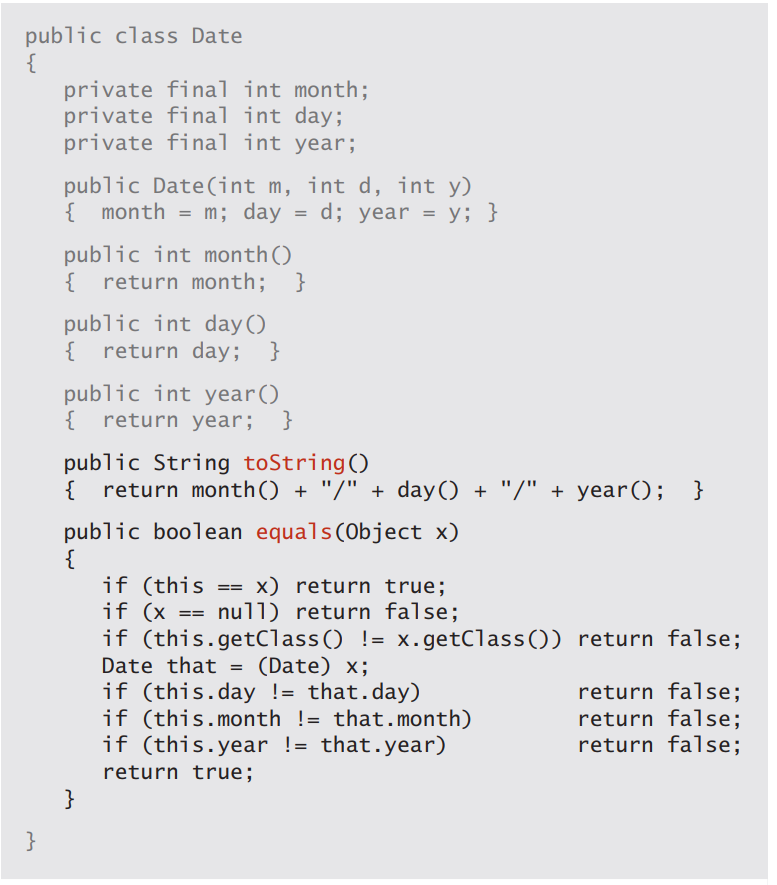
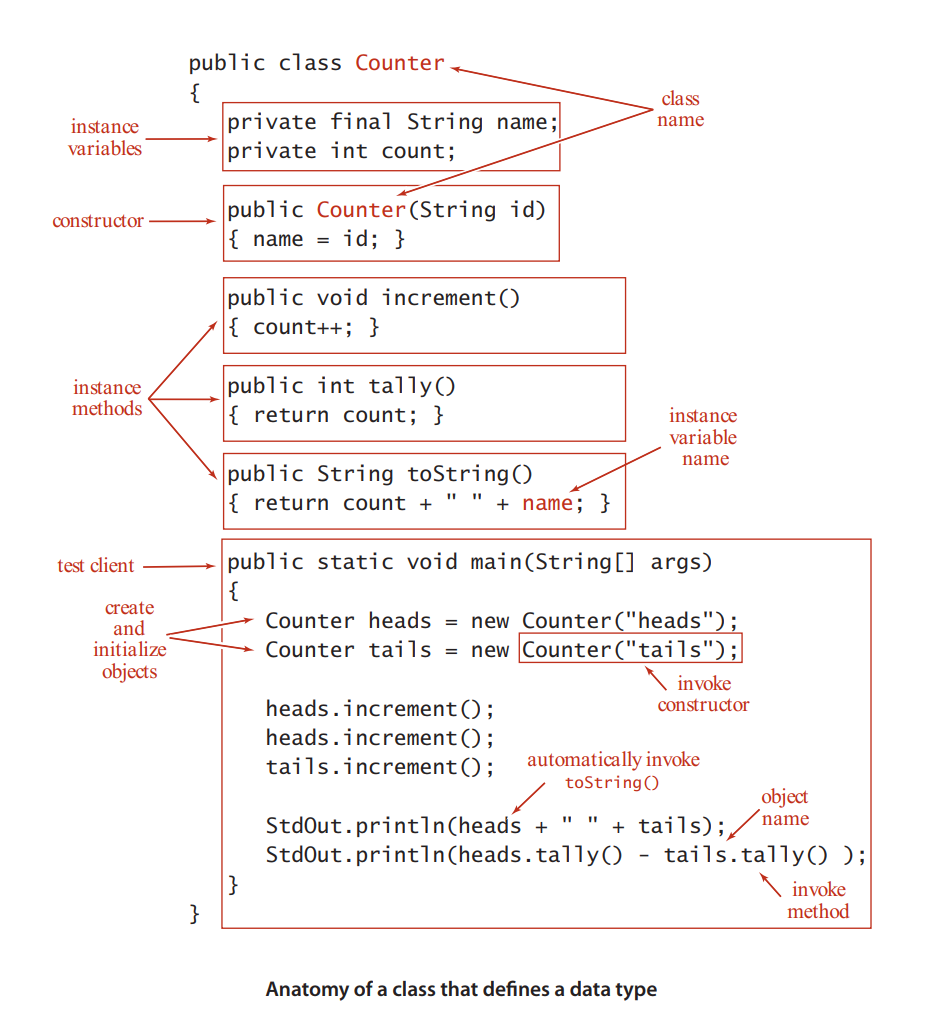
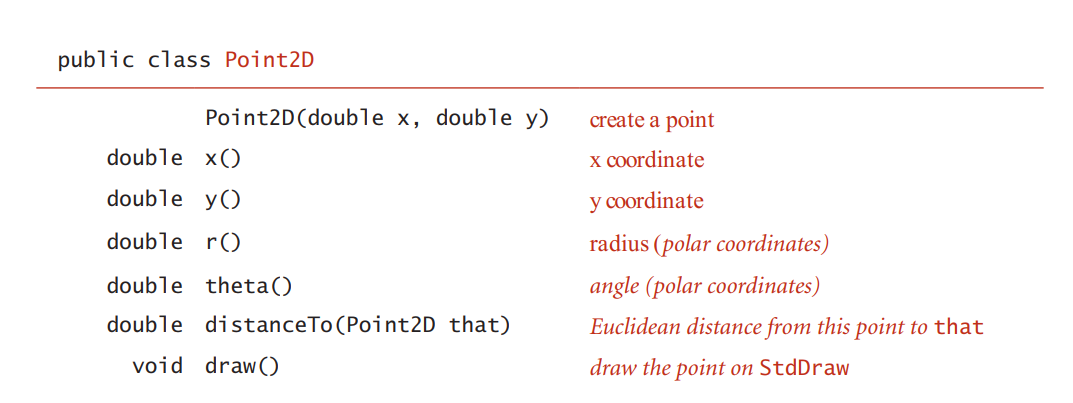
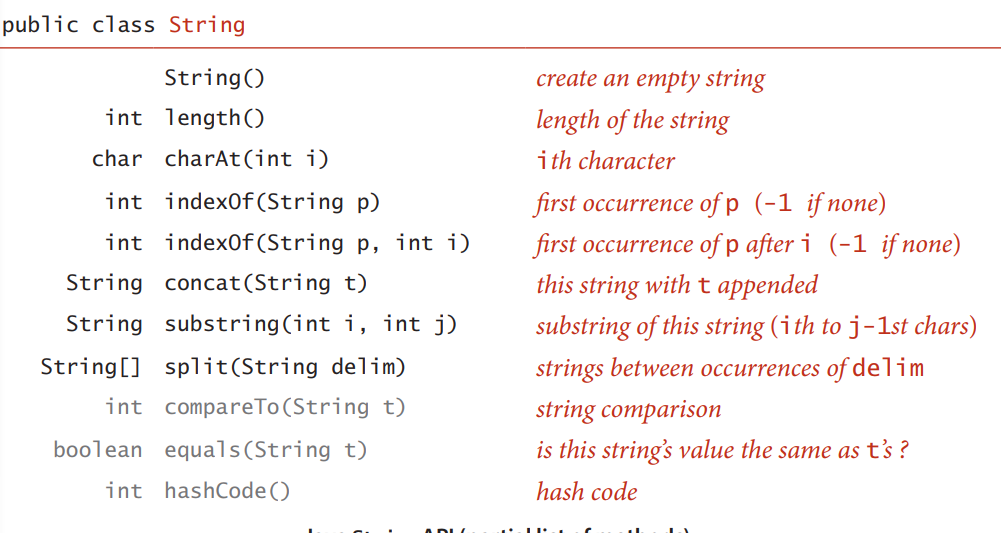
public class Date { private final int month; private final int day; private final int year; public Date(int m, int d, int y) { month = m; day = d; year = y; } public int month() { return month; } public int day () { return day; } public int year() { return year; } public String toString() { return month() + "/" + day() + "/" + year(); } public boolean equals(Object x) { if (this == x) return true; if (x == null) return false; if (this.getClass() != X.getClass()) return false; Date that = (Date) x; if (this.day != that.day) return false; if (this.month != that.month) return false; if (this.year != that.year) return false; return true; } } class public class Counter { private final String name; private int count; name instance variables constructor public Counter(String id) { name = id; } public void increment() { count++; } instance methods public int tally() { return count; } instance variable name public String toString() { return count + + name; } test client create and initialize objects public static void main(String[] args) { Counter heads = new Counter("heads"); Counter tails = new Counter("tails"); invoke heads.increment(); constructor heads.increment(); tails.increment(); automatically invoke toString object Stdout.println(heads + + tails);an name Stdout.println(heads.tally() tails.tally() ); } invoke method } Anatomy of a class that defines a data type public class Point2D Point2D(double x, double y) double x() double y() double r() create a point x coordinate y coordinate radius (polar coordinates) angle (polar coordinates) Euclidean distance from this point to that draw the point on StdDraw double theta () double distanceTo (Point2D that) void draw() public class String String() int length() char charAt(int i) int indexOf(String p) int indexOf(String p, int i) String concat(String t) String substring(int i, int j) String[] split(String delim) int compareTo(String t) boolean equals(String t) int hashCode () create an empty string length of the string ith character first occurrence of p (-1 if none) first occurrence of p after i (-1 if none) this string with t appended substring of this string (ith to j-1st chars) strings between occurrences of delim string comparison is this string's value the same as t's? hash code