Question
Error in Java Payroll Project!! I need help with payroll project. I have allt he code using JAVA. Please help me with this error. These
Error in Java Payroll Project!! I need help with payroll project. I have allt he code using JAVA. Please help me with this error.
These are the three files im using:
firefile:
Jean Brennan F
Ricky Mofsen M
hirefile:
Barry Allen M 0 H 6.75
Nina Pinella F 0 W 425.00
Lane Wagger M 0 W 725.00
payfile:
Howard Starr M 8 H 30.00
Joan Jacobus F 9 W 925.00
David Renn M 3 H 4.75
Albert Cahana M 3 H 18.75
Douglas Sheer M 5 W 250.00
Shari Buchman F 9 W 325.00
Sara Jones F 1 H 7.50
Ricky Mofsen M 6 H 12.50
Jean Brennan F 6 H 5.40
Deborah Starr F 3 W 1000.00
Jamie Michaels F 8 W 150.00
Here is my code:
/* * File: Driver.java */ import java.io.IOException; public class Driver { public static void main(String[] args) { Payroll pr = new Payroll(); try { // Read each line of data from payfile.txt, place the data into an // Employee object, and insert the Employee object onto the end of // an ObjectList. pr.readAndInsertPayfile(); // Traverse the list and output the contents of the info field of // each ObjectListNode into an easily read table format with each // field appropriately labeled. System.out.println("Details of all employees in a table format with each field appropriatley labeled..."); System.out.println("--------------------------------------------------------"); System.out.printf("%-11s%-11s%8s%8s%6s%11s ", "FirstName", "LastName", "Gender", "Tenure", "Rate", "Salary"); System.out.println("--------------------------------------------------------"); pr.printEmployeesAllDetails(); System.out.println("--------------------------------------------------------"); // Traverse the list and output the contents of the info field of // each ObjectListNode into an easily read table format with each // field appropriately. System.out.println(" Details of all employees in a table format with each field appropriatley..."); pr.printEmployeesAllDetails(); // Traverse the list and output the number of employees. pr.printNumberOfEmployees();
// Traverse the list and output the first name of all women on // payroll pr.printWomenEmployeesOnPayroll(); // Traverse the list and output the first and last names and salary // of all weekly employees who make more than $35,000 per year and // who have been with the company for at least five years. pr.printWomenEmployeesWhoMakeMoreThan35000();
// Traverse the list and give a raise of $0.75 per hour to all // employees who are paid on an hourly basis and make less than // $10.00 per hour; and give a raise of $50.00 per week to all // employees who are paid on a weekly basis and make less than // $350.00 per week. Be sure to output the first and last names and // new salaries for each employees on the payroll who has received a // raise. pr.updateAndPrintUpdatedEmployees(); // Sort the nodes of the linked list into alphabetical order // according to last name and print the first and last names and // salaries for each employee on the payroll. pr.sortEmployeesByLastName(); System.out.println(" After sorting, the first and last names for each employee on the payroll..."); pr.printEmployeesFirstAndLastNames(); // The file hirefile.txt contains data for three employees to be // hired by the company. Insert each of the new employees into the // correct location in the sorted linear linked list and print the // first and last names for each employee on the payroll. pr.readAndInsertHirefile(); System.out.println(" After hiring, the first and last names for each employee on the payroll..."); pr.printEmployeesFirstAndLastNames(); // The firefile.txt contains data for two employees to be fired by // the company. Delete the corresponding nodes in the sorted linear // linked list for each of the employees to be fired and print the // first and last names for each employee on the payroll. pr.readAndInsertFirefile(); System.out.println(" After firing, the first and last names for each employee on the payroll..."); pr.printEmployeesFirstAndLastNames(); } catch(IOException e) { System.out.println(e.getMessage()); } } }
/* * File: Payroll.java */ import java.io.File; import java.io.FileNotFoundException; import java.util.Scanner; public class Payroll { private ObjectList olist; public Payroll() { olist = new ObjectList(); } public void readAndInsertPayfile() throws FileNotFoundException { String f; String l; String g; int t; String r; double s; File file = new File("payfile.txt"); Scanner sc = new Scanner(file); Employee emp; while(sc.hasNextLine()) { f = sc.next(); l = sc.next(); g = sc.next(); t = sc.nextInt(); r = sc.next(); s = sc.nextDouble();
emp = new Employee(f, l, g, t, r, s);
olist.addLast(emp); } sc.close(); }
public void printEmployeesAllDetails() { ObjectListNode obj = olist.getFirstNode(); while(obj != null) { System.out.println(obj.getInfo());
obj = obj.getNext(); } }
public void printNumberOfEmployees() { int count = 0; ObjectListNode obj = olist.getFirstNode(); while(obj != null) { count++; obj = obj.getNext(); } System.out.println(" The number of employees in the list: " + count); }
public void printWomenEmployeesOnPayroll() { System.out.println(" The first name of all women on payroll..."); ObjectListNode obj = olist.getFirstNode(); Employee emp; while(obj != null) { emp = (Employee) obj.getInfo();
if(emp.getGender().equalsIgnoreCase("F")) { System.out.println(emp.getFirstName()); }
obj = obj.getNext(); } }
public void printWomenEmployeesWhoMakeMoreThan35000() { System.out.println(" Employees who make more than $35,000 per year and who have been with the company for at least five years..."); ObjectListNode obj = olist.getFirstNode(); Employee emp; while(obj != null) { emp = (Employee) obj.getInfo();
if(emp.getRate().equalsIgnoreCase("W") && emp.getSalary() * 52 >= 35000 && emp.getTenure() >= 5) { System.out.printf("%-11s%-11s%11.2f ", emp.getFirstName(), emp.getLastName(), emp.getSalary()); }
obj = obj.getNext(); } }
public void updateAndPrintUpdatedEmployees() { System.out.println(" Employees on the payroll who has received a raise..."); ObjectListNode obj = olist.getFirstNode(); Employee emp; while(obj != null) { emp = (Employee) obj.getInfo();
if(emp.getRate().equalsIgnoreCase("H") && emp.getSalary() < 10.0) { emp.setSalary(emp.getSalary() + 0.75); System.out.printf("%-11s%-11s%11.2f ", emp.getFirstName(), emp.getLastName(), emp.getSalary()); } if(emp.getRate().equalsIgnoreCase("W") && emp.getSalary() < 350.0) { emp.setSalary(emp.getSalary() + 50.00); System.out.printf("%-11s%-11s%11.2f ", emp.getFirstName(), emp.getLastName(), emp.getSalary()); }
obj = obj.getNext(); } }
public void sortEmployeesByLastName() { ObjectList sortList = new ObjectList(); while(!olist.isEmpty()) { Object first = olist.removeFirst(); sortList.insert(first); } olist = sortList; }
public void readAndInsertHirefile() throws FileNotFoundException { String f; String l; String g; int t; String r; double s; File file = new File("hirefile.txt"); Scanner sc = new Scanner(file); Employee emp; while(sc.hasNextLine()) { f = sc.next(); l = sc.next(); g = sc.next(); t = sc.nextInt(); r = sc.next(); s = sc.nextDouble();
emp = new Employee(f, l, g, t, r, s);
olist.insert(emp); } sc.close(); }
public void readAndInsertFirefile() throws FileNotFoundException { String f; String l; String g; File file = new File("firefile.txt"); Scanner sc = new Scanner(file); Employee emp; while(sc.hasNextLine()) { f = sc.next(); l = sc.next(); g = sc.next();
emp = new Employee(f, l, g, 0, "", 0.0);
olist.remove(emp); } sc.close(); }
public void printEmployeesFirstAndLastNames() { Employee emp; ObjectListNode obj = olist.getFirstNode(); while(obj != null) { emp = (Employee) obj.getInfo(); System.out.printf("%-11s%-11s ", emp.getFirstName(), emp.getLastName());
obj = obj.getNext(); } } }
/* * File: Employee.java */ public class Employee implements Comparable
public Employee(String firstName, String lastName, String gender, int tenure, String rate, double salary) { this.firstName = firstName; this.lastName = lastName; this.gender = gender; this.tenure = tenure; this.rate = rate; this.salary = salary; }
public String getFirstName() { return firstName; }
public void setFirstName(String firstName) { this.firstName = firstName; }
public String getLastName() { return lastName; }
public void setLastName(String lastName) { this.lastName = lastName; }
public String getGender() { return gender; }
public void setGender(String gender) { this.gender = gender; }
public int getTenure() { return tenure; }
public void setTenure(int tenure) { this.tenure = tenure; }
public String getRate() { return rate; }
public void setRate(String rate) { this.rate = rate; }
public double getSalary() { return salary; }
public void setSalary(double salary) { this.salary = salary; }
public String toString() { return String.format("%-11s%-11s%8s%8d%6s%11.2f", firstName, lastName, gender, tenure, rate, salary); }
public int compareTo(Employee other) { if(lastName.compareTo(other.lastName) < 0) return -1; else if(lastName.compareTo(other.lastName) > 0) return 1; else return 0; } }
/* * File: ObjectList.java (NOT MODIFIED) */ public class ObjectList implements ObjectListInterface { private ObjectListNode list; private ObjectListNode last;
// Constructs an empty list public ObjectList() { list = null; last = null; }
// Returns the first node in the list public ObjectListNode getFirstNode() { return list; }
// Returns the last node in the list public ObjectListNode getLastNode() { return last; }
// Returns the first object in the list public Object getFirst() { if(list == null) { System.out.println("Runtime Error: getFirst()"); System.exit(1); } return list.getInfo(); }
// Returns the last object in the list public Object getLast() { if(list == null) { System.out.println("Runtime Error: getLast()"); System.exit(1); } return last.getInfo(); }
// Adds an object to the front of a list public void addFirst(Object o) { ObjectListNode p = new ObjectListNode(o, list); if(list == null) last = p; list = p; }
// Adds a node to the front of the list public void addFirst(ObjectListNode p) { if(p == null) { System.out.println("Runtime Error: addFirst()"); System.exit(1); } p.setNext(list); if(list == null) last = p; list = p; }
// Adds an object to the end of the list public void addLast(Object o) { ObjectListNode p = new ObjectListNode(o); if(list == null) list = p; else last.setNext(p); last = p; }
// Adds a node to the end of the list public void addLast(ObjectListNode p) { if(p == null) { System.out.println("Runtime Error: addLast()"); System.exit(1); } p.setNext(null); if(list == null) list = p; else last.setNext(p); last = p; }
// Removes the first object from the list public Object removeFirst() { if(list == null) { System.out.println("Runtime Error: removeFirst()"); System.exit(1); } ObjectListNode p = list; list = p.getNext(); if(list == null) last = null; return p.getInfo(); }
// Removes the last object from the list public Object removeLast() { if(list == null) { System.out.println("Runtime Error: removeLast()"); System.exit(1); } ObjectListNode p = list; ObjectListNode q = null; while(p.getNext() != null) { q = p; p = p.getNext(); } if(q == null) { list = null; last = null; } else { q.setNext(null); last = q; } return p.getInfo(); }
// Inserts an object after the node referenced by p public void insertAfter(ObjectListNode p, Object o) { if(list == null || p == null) { System.out.println("Runtime Error: insertAfter()"); System.exit(1); } ObjectListNode q = new ObjectListNode(o, p.getNext()); p.setNext(q); if(q.getNext() == null) last = q; }
// Inserts a node after the node referenced by p public void insertAfter(ObjectListNode p, ObjectListNode q) { if(list == null || p == null || q == null) { System.out.println("Runtime Error: insertAfter()"); System.exit(1); } q.setNext(p.getNext()); p.setNext(q); if(last.getNext() != null) last = q; }
// Deletes the node after the node referenced by p public Object deleteAfter(ObjectListNode p) { if(list == null || p == null || p.getNext() == null) { System.out.println("Runtime Error: deleteAfter()"); System.exit(1); } ObjectListNode q = p.getNext(); p.setNext(q.getNext()); if(p.getNext() == null) last = p; return q.getInfo(); }
// Inserts an item into its correct location within an ordered list public void insert(Object o) { ObjectListNode p = list; ObjectListNode q = null; while(p != null && ((Comparable) o).compareTo(p.getInfo()) > 0) //Error: Comparable is a raw type. References to generic type Comparable
// Inserts a node into its correct location within an ordered list public void insert(ObjectListNode r) { ObjectListNode p = list; ObjectListNode q = null; while(p != null && ((Comparable) r.getInfo()).compareTo(p.getInfo()) > 0) { q = p; p = p.getNext(); } if(q == null) addFirst(r); else insertAfter(q, r); }
// Removes the first occurrence of an item in a list public Object remove(Object o) { ObjectListNode p = list; ObjectListNode q = null; while(p != null && ((Comparable) o).compareTo(p.getInfo()) != 0) { q = p; p = p.getNext(); } if(p == null) return null; else return q == null ? removeFirst() : deleteAfter(q); }
// Returns true if the item is found in the list public boolean contains(Object o) { ObjectListNode p = list; while(p != null && ((Comparable) o).compareTo(p.getInfo()) != 0) p = p.getNext(); return p != null; }
// Returns a reference to the node with the requested value // Returns null otherwise public ObjectListNode select(Object o) { ObjectListNode p = list; while(p != null) if(((Comparable) o).compareTo(p.getInfo()) == 0) return p; else p = p.getNext(); return null; }
// Determines whether or not a list is empty public boolean isEmpty() { return list == null; }
// Removes all elements from a list public void clear() { list = null; last = null; }
// Returns the number of elements in the list public int size() { int count = 0; ObjectListNode p = list; while(p != null) { ++count; p = p.getNext(); } return count; }
// Makes a copy of a list public ObjectList copyList() { ObjectListNode p = null; ObjectListNode q = null; // to satisfy compiler; ObjectListNode r = list;
if(isEmpty()) return null; ObjectList newList = new ObjectList(); while(r != null) { p = new ObjectListNode(r.getInfo()); if(newList.isEmpty()) newList.addFirst(p); else q.setNext(p); q = p; r = r.getNext(); } newList.last = p; return newList; }
// Reverses a list public void reverse() { ObjectListNode p = list; ObjectListNode q = null; ObjectListNode r;
while(p != null) { r = q; q = p; p = p.getNext(); q.setNext(r); } last = list; list = q; } }
/* * File: ObjectListNode.java (NOT MODIFIED) */ public class ObjectListNode { private Object info; private ObjectListNode next;
// Default ctor public ObjectListNode() { info = null; next = null; }
// One-arg ctor public ObjectListNode(Object o) { info = o; next = null; }
// Two-arg ctor public ObjectListNode(Object o, ObjectListNode p) { info = o; next = p; }
// Sets info field public void setInfo(Object o) { info = o; }
// Returns object in info field public Object getInfo() { return info; }
// Sets next field public void setNext(ObjectListNode p) { next = p; }
// Returns object in info field public ObjectListNode getNext() { return next; } }
/* * File: ObjectListInterface.java (NOT MODIFIED) */ public interface ObjectListInterface { // Adds an object to the front of a list public void addFirst(Object o);
// Adds a node to the front of the list public void addFirst(ObjectListNode p);
// Adds an object to the end of the list public void addLast(Object o);
// Adds a node to the end of the list public void addLast(ObjectListNode p);
// Inserts an object after the node referenced by p public void insertAfter(ObjectListNode p, Object o);
// Inserts a node after the node referenced by p public void insertAfter(ObjectListNode p, ObjectListNode q);
// Inserts an item into its correct location within an ordered list public void insert(Object o);
// Inserts a node into its correct location within an ordered list public void insert(ObjectListNode r);
// Removes all elements from a list public void clear();
// Reverses a list public void reverse(); }
THis is the sample output that I should be getting
Sample Output:
Details of all employees in a table format with each field appropriatley labeled... -------------------------------------------------------- FirstName LastName Gender Tenure Rate Salary -------------------------------------------------------- Howard Starr M 8 H 30.00 Joan Jacobus F 9 W 925.00 David Renn M 3 H 4.75 Albert Cahana M 3 H 18.75 Douglas Sheer M 5 W 250.00 Shari Buchman F 9 W 325.00 Sara Jones F 1 H 7.50 Ricky Mofsen M 6 H 12.50 Jean Brennan F 6 H 5.40 Deborah Starr F 3 W 1000.00 Jamie Michaels F 8 W 150.00 --------------------------------------------------------
Details of all employees in a table format with each field appropriatley... Howard Starr M 8 H 30.00 Joan Jacobus F 9 W 925.00 David Renn M 3 H 4.75 Albert Cahana M 3 H 18.75 Douglas Sheer M 5 W 250.00 Shari Buchman F 9 W 325.00 Sara Jones F 1 H 7.50 Ricky Mofsen M 6 H 12.50 Jean Brennan F 6 H 5.40 Deborah Starr F 3 W 1000.00 Jamie Michaels F 8 W 150.00
The number of employees in the list: 11
The first name of all women on payroll... Joan Shari Sara Jean Deborah Jamie
Employees who make more than $35,000 per year and who have been with the company for at least five years... Joan Jacobus 925.00
Employees on the payroll who has received a raise... David Renn 5.50 Douglas Sheer 300.00 Shari Buchman 375.00 Sara Jones 8.25 Jean Brennan 6.15 Jamie Michaels 200.00
After sorting, the first and last names for each employee on the payroll... Jean Brennan Shari Buchman Albert Cahana Joan Jacobus Sara Jones Jamie Michaels Ricky Mofsen David Renn Douglas Sheer Deborah Starr Howard Starr
After hiring, the first and last names for each employee on the payroll... Barry Allen Jean Brennan Shari Buchman Albert Cahana Joan Jacobus Sara Jones Jamie Michaels Ricky Mofsen Nina Pinella David Renn Douglas Sheer Deborah Starr Howard Starr Lane Wagger
After firing, the first and last names for each employee on the payroll... Barry Allen Shari Buchman Albert Cahana Joan Jacobus
But here is my output from my computer:
Details of all employees in a table format with each field appropriatley labeled...
--------------------------------------------------------
FirstName LastName Gender Tenure Rate Salary
--------------------------------------------------------
Howard Starr M 8 H 30.00
Joan Jacobus F 9 W 925.00
David Renn M 3 H 4.75
Albert Cahana M 3 H 18.75
Douglas Sheer M 5 W 250.00
Shari Buchman F 9 W 325.00
Sara Jones F 1 H 7.50
Ricky Mofsen M 6 H 12.50
Jean Brennan F 6 H 5.40
Deborah Starr F 3 W 1000.00
Jamie Michaels F 8 W 150.00
--------------------------------------------------------
Details of all employees in a table format with each field appropriatley...
Howard Starr M 8 H 30.00
Joan Jacobus F 9 W 925.00
David Renn M 3 H 4.75
Albert Cahana M 3 H 18.75
Douglas Sheer M 5 W 250.00
Shari Buchman F 9 W 325.00
Sara Jones F 1 H 7.50
Ricky Mofsen M 6 H 12.50
Jean Brennan F 6 H 5.40
Deborah Starr F 3 W 1000.00
Jamie Michaels F 8 W 150.00
The number of employees in the list: 11
The first name of all women on payroll...
Joan
Shari
Sara
Jean
Deborah
Jamie
Employees who make more than $35,000 per year and
who have been with the company for at least five years...
Joan Jacobus 925.00
Employees on the payroll who has received a raise...
David Renn 5.50
Douglas Sheer 300.00
Shari Buchman 375.00
Sara Jones 8.25
Jean Brennan 6.15
Jamie Michaels 200.00
After sorting, the first and last names for each employee on the payroll...
Howard Starr
Joan Jacobus
David Renn
Albert Cahana
Douglas Sheer
Shari Buchman
Sara Jones
Ricky Mofsen
Jean Brennan
Deborah Starr
Jamie Michaels
After hiring, the first and last names for each employee on the payroll...
Howard Starr
Joan Jacobus
David Renn
Albert Cahana
Douglas Sheer
Shari Buchman
Sara Jones
Ricky Mofsen
Jean Brennan
Deborah Starr
Jamie Michaels
Barry Allen
Nina Pinella
Lane Wagger
Exception in thread "main" java.lang.ClassCastException: Employee cannot be cast to java.lang.Comparable
at ObjectList.remove(ObjectList.java:219)
at Payroll.readAndInsertFirefile(Payroll.java:183)
at Driver.main(Driver.java:73)
IF someone can please help me fix my issue I've been trying for hours and haven't gotten anywwhere please show in bold the corrections you've made. With your output printed out I will appreciate it! Will give review! thanks!
Step by Step Solution
There are 3 Steps involved in it
Step: 1
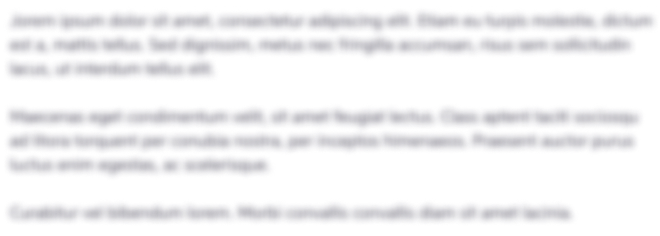
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started