Question
Essentially, you must design a Python program that prompts a user for a year, a day of the year (an integer between 1 and either
Essentially, you must design a Python program that prompts a user for a year, a day of the year (an integer between 1 and either 365 or 366 to account for leap year), and output the month, day and year. For example, give the year 2018, and the day 335, your Python program would return December 1, 2018.
To help to check the accuracy of your program, use online sources such as Day Number of the Year Calculator (Links to an external site.) to compute the day from the date. Your program must account for leap year, so use your is_leap_year() function from Lab 4.
This assignment requires the following three deliverables (in addition to Lab 4):
- A simple text file that outlines the logical steps you'd follow to accomplish the primary programming assignment (e.g. prompt for input, validate input)
- A first version of your program that uses no functions to solve the problem, following your outline
- A final version of your program that adds functions to solve the same problem using refactoring techniques (e.g. encapsulation, generalization)
Note: To solve this assignment, you MUST use Python coding elements we've learned (e.g. loops, conditionals), and not a solution potentially found online.
Day Of Year to Date Algorithm
In order to convert the day of the year to a month, day and year, you will need to calculate the month and day, accounting for leap year. Below is a tip to help you develop an algorithm to solve the problem.
Loop through the number of months, and for each month determine the number of days in the month, and see if the day of the year is
Step 1: Outline
Use a text editor such as Brackets, Atom, Sublime, Notepad++, but not a word processor, to create a file called cis122-assign04-outline.txt. On separate lines, outline the logical steps you'd follow to solve the problem. Steps might include prompt for year, prompt for day of year, validate year, calculate month, print month, day, year, etc.
You will use these outline steps in your Python program.
Step 2: Initial Solution
Use IDLE Editor to create a file called cis122-assign04-yearday-v1.py. This program must
- Include your outline steps as comments
- NOT use any user-defined function definitions other than is_leap_year() from Lab 4
- Prompt the user for a year with the prompt, "Enter year: " (e.g. 2018)
- Prompt the user for a day of the year with the prompt, "Enter day of year: " (e.g. 335)
- Return the day of the year as a string in the format of Month Day, Year (e.g. December 1, 2018)
- Not display any results from testing your code, but simply accept input, and print a result
- Validate input (see Validating Input below)
Testing Your Initial Solution
You will of course need to test your program to ensure the program works fine. You can run the program and use the input, but you'll find it easier to initially code year and day of year values. You may even consider using a loop to run through all days of the year. Remember, any testing should be commented out before submitting the initial solution.
Validating Input
Your initial solution must include code that validates the input. Below are the initial rules you'll need:
- Validate the year is > 0, if not, print the error, "Year must be > 0"
- Validate the day of the year is > 0, if not, print the error, "Day of year must be > 0"
- Validate the day of the year is less than the day of the year, depending on whether the year is a leap year, and if not, print either the error "Day must be
Step 3 - Refactored Solution
Use IDLE Editor to create a file called cis122-assign04-yearday-v2.py. Copy your code from cis122-assign04-yearday-v2.py into this file. Below are required refactoring steps. When creating functions, move the code to the functions, refactoring the code, and replace the code with the function calls. You will need to use variables or use conditional statements, including relational and logical operators.
Remember to work incrementally: make a few changes, save, and test.
Tip: Remember you can Run your Python file, and any functions in your file are now available directly from the IDLE Shell, enabling you to test your functions directly from the IDLE Shell.
- Add a void function start() that encapsulates all of your code, and then call the start() function.
- Add a fruitful function valid_year(year) that replaces year validity checks, printing out any error, and returning True if the year is valid, or False if the year is invalid. When using this function you must test for True or False.
- Add a fruitful function valid_day_of_year(year, day_of_year) that replaces day of year validity checks, printing out any error, and returning True if the day of year is valid, or False if the day of year is invalid. When using this function you must test for True or False.
- Add a fruitful function input_year() that encapsulates the prompting for the year, and returns either 0 if the year is invalid, or the year as an integer. When using this function you must test for 0 (invalid year).
- Add a fruitful function input_day_of_year(year) that encapsulates the prompting for the day of the year, and returns either 0 if the day of the year is invalid, or the day of the year as an integer. Note that the function includes a year parameter, so your function should also call both valid_day_of_year() and valid_year() functions. When using this function you must test for 0 (invalid day of year).
- Add a fruitful function get_days_in_year(year) that returns 0 for an invalid year, or the number of days in a year as an integer, and use this function in your code.
- Add a fruitful function valid_month(month) that returns True if a month is valid (e.g. 0 0" and "Month must be
- Add a fruitful function translate_month(month) that returns an empty string for an invalid month (call valid_month()), or the month as a full month string (e.g. January, December)
- Add a fruitful function get_days_in_month(year, month) that returns the number of days in a month as an integer, accounting for leap year, or returns 0 if the year or month is invalid. You should be able to use this function in your code, but even if you can't use this function, create the function. If you can use the function, then use it.
- Add a fruitful function valid_day(year, month, day) that returns False if the year, month or day are invalid, or True if the arguments are valid.
- Add a fruitful function get_date_string(year, month, day) that returns an empty string if the year, month or day are invalid, or a string formatted as Month Day, Year (e.g. December 1, 2018).
After completing these steps, you should have 12 user-defined functions in your Python code (the 11 above plus is_leap_year() from Lab 4).
Not Quite Finished
Make sure both of your Python files contain the authorship docstring at the top of each file. Also, add each function docstring.
Note: My completed refactored Python file, without any docstrings, comments or test code but with blank lines between functions, is 174 lines.
Sample Run
Each of the three sample runs below represent prompting the user for input and the resulting output.
Submissions
For this assignment you will be uploading your work from Lab 4 as well as your work from this assignment.
Create a single compressed file that contains the Lab 4 Python file and the files from Assignment 4. To create the compressed file, select the deliverable Python files, right-mouse click on the selected files, and select Compress (Mac) or Send To > Compressed (zipped) Folder (Windows). A new file will be created that contains all of the deliverable Python files.
Rename the compressed file cis122-assign04.zip. Use the Assignment 4 Canvas site to submit/upload the compressed/ZIP file.
The compressed file will contain the following deliverables (point values in parentheses).
- (5) cicis122-lab04-calendar.py
- (5) cis122-assign04-outline.txt
- (15) cis122-assign04-yearday-v1.py
- (25) cis122-assign04-yearday-v2.py
Once uploaded, revisit the Assignment 4 page in Canvas and confirm the file was uploaded.
Remember, you are responsible to submit your assignments before the deadline. Late submissions will not be accepted.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
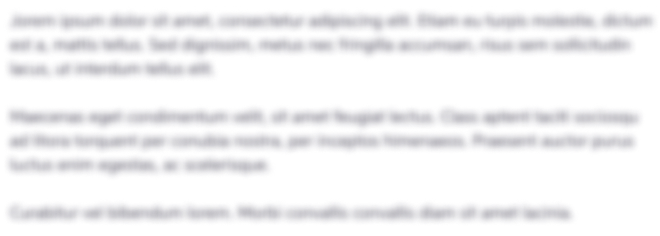
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started