Question
ETT Show what happens in terms of D-V messages exchanged by node 7, if the edge (1, 7) breaks, and then later, when edge (1,
ETT
Show what happens in terms of D-V messages exchanged by node 7, if the edge (1, 7) breaks, and then later, when edge (1, 7) is repaired. (b) Suppose that your college has 1000 members; and that Cambridge has a population of 100,000; and that the Earth's total population is ten thousand million., what is the average degree and average path length? 12 Security II (a) Write out the Needham-Schroder protocol, explaining the notation you use. (b) Describe the "bug" in the protocol, stating why some people consider it to be a bug and other people consider it not to be. [5 marks] (c) Provide an amended protocol that does not have the "bug" but which (unlike Kerberos) uses random nonces rather than timestamps. [10 marks] 9 13 Optimising Compilers (a) Define what is meant by a variable being live at a program point, carefully distinguishing versions based on program structure and I/O behaviour. Discuss these alternatives and their suitability for compilation using words like "decidable" and "safety" (b) Explain the difference between dead code and unreachable code, mentioning any analysis necessary to remove such code. (c) Let q be an expression (whose only free variable is a) which always evaluates to true although deducing this is beyond the power of a given compiler optimiser. Consider the function int p(int a, int b, int c, int d, int e) { int x = a+b, y = a+c, z=0, r; if (a<5) { r = a+x; while (1) continue; z = a+d; } else if (q) { r = y; } else { r = a+e; } return r+z; } (i) Give the flowgraph for p (assuming it to have been translated as navely as possible). [3 marks] (ii) Using your definition of liveness suitable for a compiler from part (a), give the set of variables live on entry to p, comparing this with liveness required by I/O behaviour. [4 marks] (iii) Expressing your answer in source code similar to the definition of p, give the code you might expect a good optimiser to achieve. State the optimisations used, where and in what order they were applied, and the set of variables live (under both forms of liveness) on entry to the optimised version of p
The following is one of the examples from the Foundations of Computer Science course: exception Change; fun change (till, 0) = [] | change ([], amt) = raise Change | change (c::till, amt) = if amt<0 then raise change else (c :: change(c::till, amt-c)) handle> change(till, amt); (a) Define a Java class of your own (i.e. do not use any library class that you may be aware of) to represent linked lists of integers. Provide it with methods that can be used to reverse a list and to append two lists. Comment on whether your design has led you to make the methods for append and reverse static. [7 marks] (b) Prepare a method called change that works in the same way as the ML code shown above. Provide the class that you define it in with a main method that uses it to try to make change for 73p using 2p, 5p and 20p coins, printing the result neatly. [7 marks] (c) If you have lists of non-zero integers such that all the values in them are less than 256 and you have at most 8 items in any list you can pack eight 8-bit fields into a single 64-bit "long". That gives a representation that some people might expect to be faster than using lists represented by chained up instances of a class. Re-work your change-giving code based on the above idea, and modelling the use of exceptions by making the change function return -1L in the exceptional case. (a) What is a binary search tree rotation, and how are rotations useful in the creation of efficient search tree algorithms? [2 marks] (b) Write pseudocode for a recursive function select(x, i) which, given a binary search tree with root node x, executes a sequence of rotations to move the i th largest node to the root of the tree and returns a pointer to the new root node. [6 marks] (c) Making use of select to deal with tricky cases, write pseudocode for a recursive function delete(x, k) which deletes the node containing key value k from the tree and returns a pointer to the new root node. [5 marks] (d) Write a more efficient version of delete which does not use recursion or rotation to perform its work. How does its time complexity compare with your answer to part (c)? [7 marks] 12 Algorithms (a) Carefully describe the purpose and structure of a skip list. Provide a pseudocode definition for a skip-list node. [7 marks] (b) Write pseudocode to find an integer in a skip list.
(a) Some program state is per-process, and some is per-thread. How many instances of each of the following will a 2-thread process have: virtual address space, executable program, register file, scheduling state (e.g., RUN, SLEEP), and stack? [5 marks] (b) A programmer adds printfs to a concurrent program to debug a race condition, but the symptoms vanish. Explain why this might have happened. [2 marks] (c) thrprint accepts as arguments the current thread's unique ID and a debug message to print. If each thread calls thrprint exactly once on start, how many possible interleavings are there with n threads? [2 marks] void thrprint(int threadid, char *message) { printf("Thread %d: %s ", threadid, message); } (d) ordered thrprint attempts to print debug messages ordered by thread ID. Describe three ways in which the synchronisation in this implementation is incorrect, and provide a corrected pseudocode implementation. [6 marks] int next_thread_id = 0; // Next ID to print pthread_mtx_t ordering_mtx; // Lock protecting next ID pthread_cond_t ordering_cv; // next_thread_id has changed void ordered_thrprint(int thread_id, char *message) { pthread_mtx_lock(ordering_mtx); if (thread_id != next_thread_id) { pthread_cond_wait(ordering_cv, ordering_mtx); } next_thread_id = next_thread_id + 1; pthread_mtx_unlock(ordering_mtx); printf("Thread %d: %s ", thread_id, message); } (e) This approach to implementing ordered thrprint suffers a substantial performance problem: if lower-numbered threads are slow in starting, then higher-numbered threads will also be delayed. Describe an alternative strategy, paying particular attention to synchronisation, that maintains ordered output while allowing greater concurrency.
(a) Explain how to check a large number for primality using a probabilistic method that gives you a bound of the probability of getting an incorrect judgment. [7 marks] (b) Give an asymptotic formula predicting the number of computer operations needed to verify that a number with n bits is prime, supposing that multiplication, division and remaindering are done using O(n 2 ) methods and that you want to achieve a probability of error bounded by 1 in 260. You do not need to prove that the algorithm you describe works, but you should nevertheless explain it carefully and completely. [7 marks] (c) The gap between adjacent primes near the integer N is roughly log(N). Estimate roughly the number of computer operations you would expect to be needed to find a 2000-bit prime that is just slightly larger than some given 2000-bit random number
Explain the mechanisms and computational significance of nerve impulse generation and transmission. Include the following aspects: (a) Equivalent electrical circuit for an excitable nerve cell membrane. (b) How different ion species flow across the membrane, in terms of currents, capacitance, conductances, and voltage-dependence. (Your answer can be qualitative.) (c) Role of positive feedback and voltage-dependent conductances. (d) The respect in which a nerve impulse is a mathematical catastrophe. (e) Approximate time-scale of events, and the speed of nerve impulse propagation. (f ) What happens when a propagating nerve impulse reaches an axonal branch. (g) What would happen if two impulses approached each other from opposite directions along a single nerve fibre and collided. (h) How linear operations like integration in space and time can be combined in dendritic trees with logical or Boolean operations such as AND, OR, NOT, and veto. (i) Whether "processing" can be distinguished from "communications" as it is for artificial computing devices.
(a) Give the typing rules for the polymorphic lambda calculus (PLC). [5 marks] (b) Let prod(1, 2) denote the PLC type ((1 (2 )) ). Explain how it behaves like the ML product type 1 * 2. To do so, you should give PLC expressions pair , fst and snd of types 1(2(1 (2 prod(1, 2)))), 1(2(prod(1, 2) 1)), and 1(2(prod(1, 2) 2)) respectively, corresponding to the ML polymorphic pairing and projection functions fn x1 => fn x2 => (x1, x2) , fn (x1, x2) => x1 , and fn (x1, x2) => x2 . Give proofs for the typing of pair and fst, and explain the beta-conversion properties of fst 1 2(pair 1 2 M1 M2), for any PLC types 1, 2 and terms M1, M2. [10 marks] (c) Is it always the case that a PLC term M of type prod(1, 2) is beta-convertible to pair 1 2(fst 1 2 M)(snd 1 2 M)? Justify your answer. [Hint: consider terms with free variables.]
The Ultimate Dating Agency has a database which contains enough information to respond to requests like: List every computer scientist with any friends who obsess(es) about some reprogrammable device(s). (a) Describe the different queries that result from adding each morphological suffix in brackets. [2 marks] (b) Give formulae of first-order logic which represent these different queries. [6 marks] (c) Describe techniques for morphological analysis, syntactic parsing and compositional semantic interpretation that would output such representations
write program that creates and initialize a four-element double array . calculate and display the average of its values.
write program that calculates and prints payslips. User inputs are the name of employee, numbers of hours worked and hourly rate.
. Write program that insert number from the keyboard, and find out the number is prime or not? Write program that print the even numbers between 0 and 50. (Use loop and branching to solve this problem) by MIPS assembly language
This question assumes familiarity with the process language SPL and its eventbased semantics. Suppose that there are three agents A, B, S which run at most once (so with no replication) according to the scheme: A B : A B A : n (a nonce) A B : {n}Key(A,S) B S : {A, {n}Key(A,S)}Key(B,S) S B : {A, n}Key(B,S) The symmetric key Key(A, S) is shared between A and S, and the symmetric key Key(B, S) between B and S. (a) Express the agents A, B and S as processes in SPL. [3 marks] (b) Describe diagrammatically the reachable events of A, B and S, taking care to specify the pre- and postconditions, and actions of the events (you may, however, omit descriptions of the control conditions). [5 marks] (c) The agents communicate in the presence of an attacker which can decrypt and encrypt messages with available keys, and compose and decompose messages. Draw the four kinds of events (decryption, encryption, composition, decomposition), taking care to specify the nature of their pre- and postconditions. [2 marks] Let P be the Petri net obtained as the union of the events of A, B, S and the attacker. (d) Let Q(M) be the property of net P's markings M which holds when no output message in M has either key Key(A, S) or Key(B, S) as a submessage. Explain why the property Q holds at all reachable markings of P provided it holds at its initial marking. [6 marks] (e) Assume that Q h.
(a) (i) Describe the four classes in Flynn's taxonomy of computing systems. [4 marks] (ii) Describe Amdahl's law and use it to calculate the maximum speedup achievable by running a program, P, on a multicore system, S, where 80% of P is parallel and S contains 16 cores. [4 marks] (b) Consider the following pseudo-code that is run on a SIMD processor with 8 lanes, where i gives the lane number. r1 = load X[i] r2 = load Y[i] if (i%2 == 0) if (i%8 == 0) r1 = r1 * 2 r1 = r1 + r2 endif else r1 = r1 - r2 endif store r1, X[i] (i) Describe how the processor can support branch divergence between the different lanes. [4 marks] (ii) With the aid of a diagram, show the utilisation of the SIMD lanes for each pseudo-code operation, hence calculate the code's efficiency (overall utilisation of the SIMD lanes).
(a) Explain clearly an algorithm to schedule assembler code for a given architecture to increase its efficiency; the algorithm must give exactly the same instructions as in the original code, possibly in a different order. You may assume the hardware is interlocked so that NOPs are not required. Your answer should state the range over which scheduling takes place and, if this is smaller than a procedure, what actions can sensibly be taken at the boundary between separately scheduled sections of code. [10 marks] (b) Consider an architecture which is ARM-likethe first operand is the destination register except in the case of stores. All operations take one cycle, with the exceptions that (i) using the value resulting from an immediately previous load will cause a single-cycle stall, as does (ii) the second of two successive store instructions. Use your algorithm to schedule the following code, noting briefly, for each instruction emitted, why your algorithm has chosen this instruction over other candidates. ldr r3,[r1,#0] str r3,[r2,#0] ldr r4,[r1,#4] str r4,[r2,#4] add r5,r4,#4 [6 marks] (c) Give with brief explanation the number of possible valid schedulings of the following code (not just the one produced by your algorithm): add r3,r1,r2 or r4,r1,r2 sub r5,r3,r4 xor r4,r1,r3
The multiplexer MUX, register REG c (where c is the initial value) and combinational unit COM f (where f is the function computed) are defined to have the behaviour given below. MUX(sw,i1,i2,o) = t. o t = if sw t then i1 t else i2 t REG c (i,o) = (o 0 = c) t. o(t+1) = i t COM f (i,o) = t. o t = f(i t) Using only instances of MUX, REG c and COM f design a device DEV(c,f) that satisfies DEV(c,f)(reset,i,o) = (o 0 = c) t. o(t+1) = if reset(t+1) then c else f(o t) [8 marks] Prove that your design meets this specification.
(a) Consider the "eigenfaces" approach to face recognition in computer vision. (i) What is the role of the database population of example faces upon which this algorithm depends? (ii) What are the features that the algorithm extracts, and how does it compute them? How is any given face represented in terms of the existing population of faces?
Step by Step Solution
There are 3 Steps involved in it
Step: 1
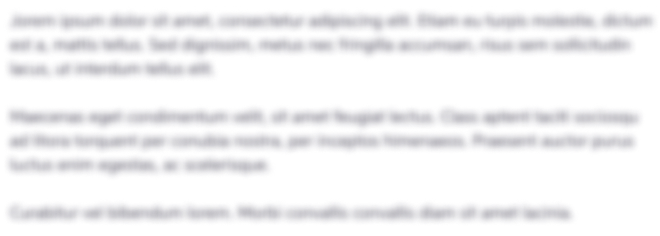
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started