Answered step by step
Verified Expert Solution
Question
1 Approved Answer
Even - Odd Sort: This is the compare and swap function. Pass = 0 = = > even pass. Pass = 1 = = >
EvenOdd Sort:
This is the compare and swap function. Pass even pass. Pass odd pass.
int Sortint Pass, int x int n
int i temp, Swapped FALSE;
for i Pass; i n i
if xi xi
temp xi;
xi xi;
xi temp;
Swapped TRUE;
return Swapped;
This is the evenodd sorting function
#define Even
#define Odd
int Swapped, i n;
Swapped TRUE;
while Swapped
Swapped SortEven x n;
Swapped Swapped SortOdd x n;
The number of comparisons in each iteration is n The total number of comparisons to sort the array is On
Making the EvenOdd Sort Concurrent:
The EvenOdd sort can easily be converted to a concurrent version. All of the n comparisons in each pass are independent of each other, and can be executed concurrently. For each even or odd pass, create n threads, each compares two adjacent entries of the array and swaps them if needed. Once a pass completes, either kill all threads and create another n new threads to perform the next pass or use the original n threads to sort a different pair of adjacent elements. Be very careful so that race conditions do not occur.
The Algorithm:
Suppose the input array x has n numbers. Use a while loop that iterates at most n times as shown earlier. In each iteration, do the following until no swaps occur.
Even Pass:
Create at most n threads: T T T etc. Thread Tk compares xk and xk If xk and xk are out of order, thread Tk swaps them and sets a flag to indicate a swap has occurred. Wait until all threads finish their work. This completes an even pass of this iteration.
Odd Pass:
Create at most n threads: T T T etc. Thread Tk compares xk and xk If xk and xk are out of order, thread Tk swaps them and sets a flag to indicate a swap has occurred. Wait until all threads finish their work. This completes an odd pass of this iteration.
Final Test: Break this while loop if no swaps occurred because the array has been sorted.
In this concurrent version, if we ignore the cost of all thread creation and termination, we need at most n even passes and n odd passes ie On passes In each even or odd pass, all comparisons are done concurrently, so each pass only takes the time of comparing one pair of numbers ie O time
Program Logic
Write a program ie the main to read in n integers into the array x and iterate at most n times. In each iteration, the main creates at most n threads to execute an even pass and then another n threads to execute an odd pass. The main must wait until all created threads of this pass complete before going for the next pass. If none of the even pass and odd pass swapped any numbers, the main prints out the sorted array.
The input array should be read in from stdin. The input array x and the flag that indicated if any numbers have been swapped are global variables shared by all threads. You can only use the above program structure and the indicated thread creation and thread join. No other thread andor process functions can be used for this program.
The input to your program should be taken from stdin. Your executable must be named as prog The command line looks like the following, where inputfilename is a file from which prog reads in the input values:
prog inputfilename
The input file has the following format, where n is a positive integer, and x x xn are n distinct integers. Assume all input values being correct small numbers ie in the range of and
n
x x x xn
Suppose the command line is
prog intxt
and the file intxt has the following lines:
View the picture
All programs must be written in C
The following approach will be used to compile and test programs:
make noVisual make your program wo visualization
prog intxt test your program
This procedure may be repeated a number of times with different input files to see if your program works correctly.
Your implementation should fulfill the program specifications as stated.
README.txt is required to answer the following questions:
Are there any race conditions in this evenodd sort?
In each iteration, main does the creation and join for the completion of n threads twice, one for even pass and one for odd pass. It requires a significant amount of time in creating and joining threads. If you use extra variablesarrays and busy waiting, can you create n threads and let them do even pass and odd pass in the same iteration without race conditions and still deliver correct results?
Can you create n threads at the very beginning and let them do all the even pass and odd pass comparisons? This saves time on creating and joining threads. Suggest a solution and discuss its correctness.
Elaborate each answer and provide details.
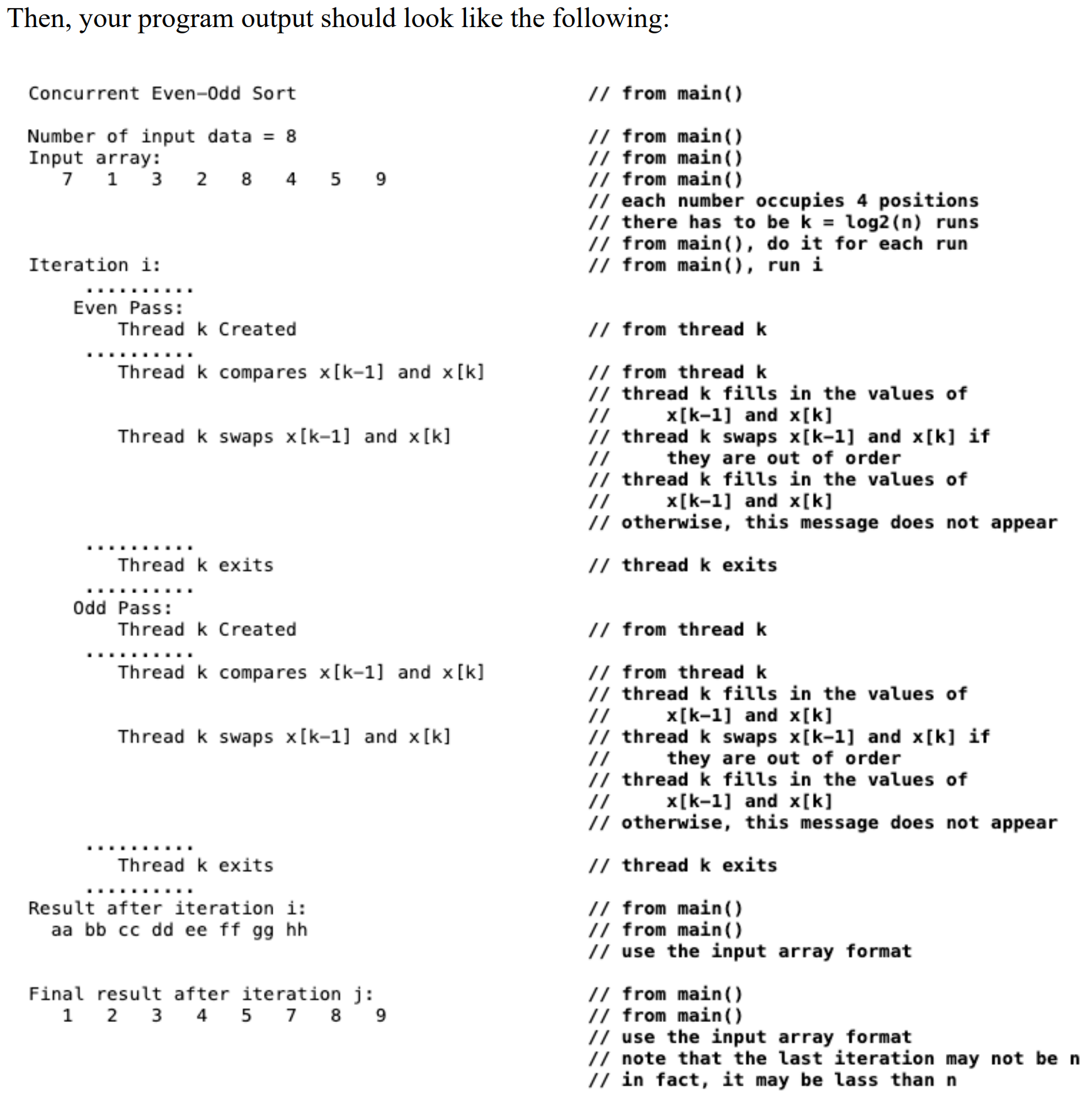
Step by Step Solution
There are 3 Steps involved in it
Step: 1
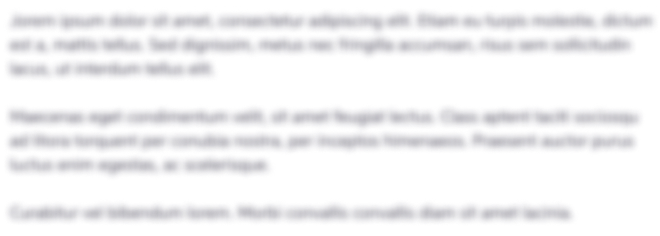
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started