Answered step by step
Verified Expert Solution
Question
1 Approved Answer
example _ main.cpp . #include #include Juice.hpp #include Tea.hpp #include Coffee.hpp using namespace std; int main ( ) { / / Default
examplemain.cpp #include
#include "Juice.hpp
#include "Tea.hpp
#include "Coffee.hpp
using namespace std;
int main
Default beverages
Juice defaultjuice;
Tea defaulttea;
Coffee defaultcoffee;
cout defaultjuice.info endl;
cout defaulttea.info endl;
cout defaultcoffee.info endl;
Test drink
cout "Drank servings of OJ: jdrink "calories" endl;
return ;
Base class Beverage
This class should be implemented in files Beverage.hpp and Beverage.cpp the starter code already provides declarations in Beverage.hpp The class should include the following:
a Protected data members:
i name string
ii caloriesperserving integer
b Public methods:
i Constructor that initializes both data members Beveragestring name, int caloriesperserving;
ii Copy Constructor that initializes both data members using a reference to another Beverage object
Beverageconst Beverage& beverage;
iii. Default Constructor that takes no arguments and initializes namedefaultbeverage and
caloriesperserving
Beverage;
iv drink that returns an integer and takes one integer parameter servings. The return value is the
product of caloriesperserving and servings.
int drinkint servings;
v info that returns a string and takes no parameters. The return value is a string in the following
format: name,caloriesperserving For example: water,
string info;
Derived class Juice
This class should be implemented in files Juice.hpp and Juice.cpp Class Juice should be a public child of class Beverage. The class should include the following:
a Private data members: i fruit string
b Public methods:
i Constructor that initializes data member and parent class
Juicestring name, int caloriesperserving, string fruit;
ii Copy Constructor that initializes data members and parent class using a reference to another
Juice object
Juiceconst Juice& juice;
iii. Default Constructor that takes no arguments and initializes fruitunknown Juice;
iv info that returns a string and takes no parameters. The return value is a string in the following format: name,fruit,caloriesperserving
For example: tropicana,orange, string info;
Derived class CaffeinatedBeverage
This class should be implemented in files CaffeinatedBeverage.hpp and CaffeinatedBeverage.cpp Class CaffeinatedBeverage should be a public child of class Beverage. The class should include the following:
c Protected data members:
i caffeinelevel integer
d Public methods:
i Constructor that initializes data member and parent class
CaffeinatedBeveragestring name, int caloriesperserving, int caffeinelevel;
ii Copy Constructor that initializes data members and parent class using a reference to another
CaffeinatedBeverage object
CaffeinatedBeverageconst CaffeinatedBeverage& caffeinatedbeverage;
iii. Default Constructor that takes no arguments and initializes caffeinelevel CaffeinatedBeverage;
iv info that returns a string and takes no parameters. The return value is a string in the following format: name,caffeinelevel,caloriesperserving
For example: redbull, string info;
Derived class Coffee
This class should be implemented in files Coffee.hpp and Coffee.cpp Class Coffee should be a public child of class CaffeinatedBeverage. The class should include the following:
e Private data members:
i beantype string
f Public methods:
i Constructor that initializes data member and parent class
Coffeestring name, int caloriesperserving, int caffeinelevel,
string beantype;
ii Copy Constructor that initializes data members and parent class using a reference to another Coffee object
Coffeeconst Coffee& coffee;
iii. Default Constructor that takes no arguments and initializes beantypedefaultbean
Coffee;
iv info that returns a string and takes no parameters. The return value is a string in the following format: name,beantype,caffeinelevel,caloriesperserving
For example: starbucks,arabica, string info;
Derived class Tea
This class should be implemented in files Tea.hpp and Tea.cpp Class Tea should be a public child of class CaffeinatedBeverage. The class should include the following:
g Private data members: i teatype string
h Public methods:
i Constructor that initializes data member and parent class
Teastring name, int caloriesperserving, int caffeinelevel,
string teatype;
ii Copy Constructor that initializes data members and parent class using a reference to another Tea object
Teaconst Tea& tea;
iii. Default Constructor that takes no arguments and initializes teatypedefaulttea
Tea;
iv info that returns a string and takes no parameters. The return value is a string in the following format: name,teatype,caffeinelevel,caloriesperserving
For example: lipton,black, string info;
Testing

Step by Step Solution
There are 3 Steps involved in it
Step: 1
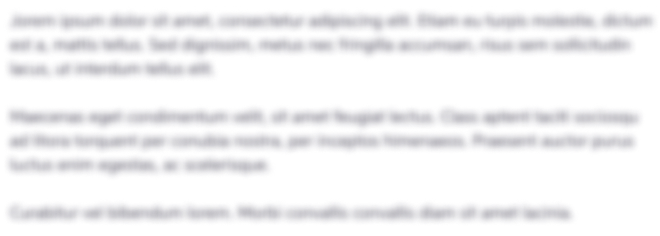
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started