Question
Example Program 1: Arrays of Objects #include #include using namespace std; class Vector3D{ private:static int NumberOfVectors;double X;double Y;double Z;double Length(); public:Vector3D(); // parameterless construcVector3D(double x,
Example Program 1: Arrays of Objects #include
Vector3D::Vector3D(){ // parameterless constructorX = 0.;Y = 0.;Z = 0.;NumberOfVectors++;} Vector3D::Vector3D(double x, double y, double z){ // 3 parameter constructorX = x;Y = y;Z = z;NumberOfVectors++;} double Vector3D::Length(){ double length = X*X + Y*Y + Z*Z; length = sqrt(length); return length;} void Vector3D::Print(){ cout
double SumLength(Vector3D v[]){// Note: This is not a member function of the Vector3D class cout
Output from above Program : NumberOfVectors = 3 Total Length = 10.1962
2. Following examples from Week8 (pg. 34) and Week9 (pg. 6) lecture notes create your own Vector3D class. Reproduce the behavior shown on pg. 8 of Week9 notes. a) Add a new member variable to the class called "Name". Name will be a string type which holds a user assigned name of the vector for display purposes. (See Lab 8 for C++ string type). The default object Name should be "Vec3D". b) Create one parameterless constructor which assigns the default values [0.,0.,0.]forthe components. Create an overridden version of the constructor which allows one to assign all three (zero or non-zero) components to the vector along with the Name of the vector. c) Create a Print function which will print the components of the vector along with the Name. For example: Vec3D =[1.0,2.9,5.0]. d) Create a new member function in the Vector3D class called Cross. The Cross member function with take in two existing vectors, v1 and v2, and compute the cross product ( v1x v2), and produce the vector v 3 where v 3 is a Vector 3D object and v3 will contain the result of the cross product. The use of the function will be v3. Cross (v1,v2) so that v3 is the calling object. See Week9 (pg 24) for some hints, the Cross function will be similar to the Add function shown there. e) Exercise the above program by computing the cross product of v1=[1,1,1] and v2=[2,3,4]. Assign the name " v3 " to the object that calls the Cross function for this example. 3. Create an array of 10 Vector3D objects. Use a for loop to print out (using your Print function) each of the Vector3D objects in the array. (Note: Without any assignments, these should all be vectors with components 0.,0.,0. and have the common name Vec3D. 2. Following examples from Week8 (pg. 34) and Week9 (pg. 6) lecture notes create your own Vector3D class. Reproduce the behavior shown on pg. 8 of Week9 notes. a) Add a new member variable to the class called "Name". Name will be a string type which holds a user assigned name of the vector for display purposes. (See Lab 8 for C++ string type). The default object Name should be "Vec3D". b) Create one parameterless constructor which assigns the default values [0.,0.,0.]forthe components. Create an overridden version of the constructor which allows one to assign all three (zero or non-zero) components to the vector along with the Name of the vector. c) Create a Print function which will print the components of the vector along with the Name. For example: Vec3D =[1.0,2.9,5.0]. d) Create a new member function in the Vector3D class called Cross. The Cross member function with take in two existing vectors, v1 and v2, and compute the cross product ( v1x v2), and produce the vector v 3 where v 3 is a Vector 3D object and v3 will contain the result of the cross product. The use of the function will be v3. Cross (v1,v2) so that v3 is the calling object. See Week9 (pg 24) for some hints, the Cross function will be similar to the Add function shown there. e) Exercise the above program by computing the cross product of v1=[1,1,1] and v2=[2,3,4]. Assign the name " v3 " to the object that calls the Cross function for this example. 3. Create an array of 10 Vector3D objects. Use a for loop to print out (using your Print function) each of the Vector3D objects in the array. (Note: Without any assignments, these should all be vectors with components 0.,0.,0. and have the common name Vec3DStep by Step Solution
There are 3 Steps involved in it
Step: 1
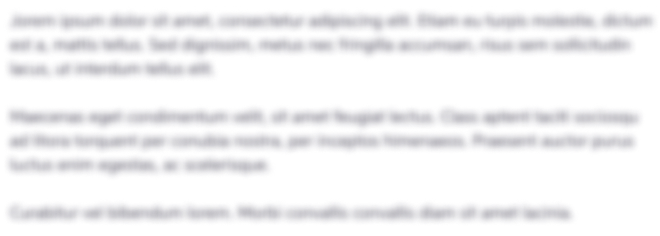
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started