Question
Exercise 1 (10 Points): Define a FixdLenStringList class that encapsulate a list of strings and a string length. All string in a FixdLenStringList have the
Exercise 1 (10 Points): Define a FixdLenStringList class that encapsulate a list of strings and a string length. All string in a FixdLenStringList have the same length. There is not limit on number of strings (dynamic array) that a FixdLenStringList can hold. For example, The FixdLenStringList list1 may contain the strings aa, bb, cc, and dd (all strings of length 2). The FixdLenStringList list2 may contain the strings cat, dog, pig, fox, bat, and eel (all strings of length 3). An incomplete implementation of the FixdLenStringList.java class is provided with this exercise: Here are the required methods in the FixdLenStringList class that you need to implement. i) Public constructor This constructor will have one parameter that will indicate the length of each string in FixdLenStringLists list. The constructor will initialize strLength with this value and will initialize FixdLenStringLists list of strings, possibleStrings, to have 0 entries. ii) You are to implement the found method. This method returns true if its String parameter key is found in the list of strings, false otherwise. iii) You are to implement the addString method. This method will add a string to the FixdLenStringLists list if it is the correct length and not already in the list. If the string is already in the list or if the string is not the correct length, it is not added. HINT: you may call method found that is specified in part ii of this problem. iv) You are to implement the method removeRandomString that will remove and return a random string entry from FixdLenStringLists list of strings. Write a client test class for testing the FixdLenStringList class. Supply, your client class along with screen shots of some test runs.
Exercise 2: [20 Points] In this exercise, we will create a memory game called Mind Game. In this game, even number of tiles are placed face-down in rows on a Mind Game Board. Each tile contains an image. For each tile, there is exactly one other tile with the same image. The player is to pick a tile, see which image it has, and try to find its match from the remaining face-down tiles. If the player fails to turn over its match, both tiles are turned face-down and the player attempts to pick a matching pair again. The game is over when all tiles on the board are turned face-up. In this implementation of a simplified version of Mind Game, Images will be strings chosen from a FixdLenStringList. (Use the FixdLenStringList class from exercise 1). The mind game board will contain even number of Tiles. The board will be implemented as a one-dimensional array of Tiles. For example, if the size of the mind game board is requested to be 4, then the board will have 16 tiles. The one dimension array, gameBoard, can be viewed as a 4-by-4 mind game board as follows: gameBoard[0] gameBoard[1] gameBoard[2] gameBoard[3] gameBoard[4] gameBoard[5] gameBoard[6] gameBoard[7] gameBoard[8] gameBoard[9] gameBoard[10] gameBoard[11] gameBoard[12] gameBoard[13] gameBoard[14] gameBoard[15] An incomplete implementation of the Tile and Board classes are provided with this exercise (Tile.java, Board.java). You should examine these classes and complete them with the following methods. To implement the methods, see the specifications provided in the comments of the methods. a) Write the implementation of the Board method fillBoard, which will randomly fill the mind game board with Tiles whose images (strings) are randomly chosen from the strings contained in the FixdLenStringList. A tile image that appears on the board appears exactly twice on the board. b) Write the implementation for the Board method lookAtTile. This method will call the appropriate method of the Tile class to turn the Tile face-up. c) Write the implementation for the Boards method checkMatch. This method will check whether the tiles in its two integer parameter positions on gameBoard have the same image. If they do, the tiles will remain face-up. If they have different images, then tiles will be turned face-down. (See the comments that were given in the problem specification). d) Complete the implementation for printBoard so that the Mind Game board is printed as described in the comment. For example, you should call the Board method format to right-justify the printing of the Tile image or the printing of the Tile position. An example of printBoard for a 4 by 4 mind game board that is partially solved is shown below after 4 matches have been found. The FixdLenStringList passed as a parameter to Board constructor contains strings of length 3. fox fox dog dog cow 5 6 7 cow cat 10 11 12 13 14 15 Write a MindGame.java class that will handle user input and simulate the mind game to the user. An example run of the mind Game is provided below: 0 1 2 3 Choose positions:1 2 pig , cat 0 1 2 3 Choose positions:0 1 cat , pig 0 1 2 3 Choose positions:0 3 cat , pig 0 1 2 3 Choose positions:1 3 pig , pig 0 pig 2 pig Choose positions:0 2 cat , cat cat pig cat pig
import java.util.ArrayList; import java.util.Collections;
/** * The mind game board containing n by n tiles. * * @author musfiqrahman */ public class Board {
private Tile[] gameBoard; // Mind Game board of Tiles private int size; // Number of Tiles on board private int rowLength; // Number of Tiles printed in a row private int numberOfTileFaceUp; // Number of Tiles face-up private FixdLenStringList possibleTileValues; // Possible Tile values
/** * Constructs n by n mind game board of Tiles whose image values are chosen * from the already filled FixdLenStringList. Precondition: n is the length * of a side of the board, n is an even positive integer. FixdLenStringList * contains at least n * n / 2 strings. * * @param n is length of the side of the mind game board (an even number) * @param list the FixdLenStringList from which the values for the tiles are * chosen */ public Board(int n, FixdLenStringList list) {
// your code goes here
}
/** * Randomly fills this Mind Game Board with tiles. The number of distinct * tiles used on the board is size/2. Any one tile image appears exactly * twice. Precondition: number of position on board is even, * FixdLenStringList contains at least size / 2 elements. */ private void fillBoard() {
// Your code goes here // You may find Collections.shuffle() class useful.
}
/** * Precondition: 0 <= p < gameBoard.length. Precondition: Tile in position p * is face-down. Postcondition: After execution, Tile in position p is * face-up @param p the index of the tile to turn face-up */ public void lookAtTile(int p) {
// Your code goes here
}
/** * Checks whether the Tiles in pos1 and pos2 have the same image. If they * do, the Tiles are turned face-up. If not, the Tiles are turned face-down. * Precondition: gameBoard[pos1] is face-up, gameBoard[pos2] is face-up * * @param pos1 index in gameBoard, 0 <= pos1 < gameBoard. length @param pos2 * index in gameBoard, 0 <= pos1 < gameBoard. length */ public void checkMatch(int pos1, int pos2) { // Your code goes here
}
/** * Board is printed for the Player. If the Tile is turned face-up, the image * is printed. If the Tile is turned face-down, the Tile position is * printed. */ public void printBoard() {
final int PADDING = 3; int spacing = possibleTileValues.getStringLength() + PADDING; for (int i = 0; i < size; i++) { // Your code goes here } }
/** * Returns Tile in position pos. Precondition: 0 <= pos < gameBoard.length. * @param pos * * is index in gameBoard * @return tile in position pos, null otherwise */ public Tile pickTile(int pos) { if (pos < 0 || pos >= gameBoard.length) { return null; }
return gameBoard[pos];
}
/** * Right-justifies a number to a given number of places. * * @param number an integer to be formatted * @param p total number of characters in returned string * @return right-justified number with p places as a string */ private String format(int number, int p) { String str = String.format("%1$" + p + "s", (number + "")); return str; }
/** * Right-justifies a string to a given number of places. * * @param word a string to be formatted * @param p total number of characters in returned string * @return right-justified word with p places as a string */ private String format(String word, int p) { String str = String.format("%1$" + p + "s", word); return str; }
/** * Checks whether all tiles are face-up. * * @return true if all tiles are face-up; false otherwise */ public boolean allTilesUp() { // Your code goes here }
}
import java.util.Scanner;
/** * This client class play starts the mind game and controls user input. */
public class MindGameClient {
public static void main(String[] args) {
FixdLenStringList fl = new FixdLenStringList(3); fl.addString("cat"); fl.addString("dog"); fl.addString("pig"); fl.addString("eel"); fl.addString("pet"); fl.addString("net"); fl.addString("ted"); fl.addString("car");
Board b = new Board(4, fl); b.printBoard();
Scanner kb = new Scanner(System.in);
while (!b.allTilesUp()) { System.out.print("Choose positions:");
int n1 = kb.nextInt(); int n2 = kb.nextInt();
if (b.pickTile(n1) != null && b.pickTile(n2) != null) {
System.out.print(b.pickTile(n1).getImage() + " , "); System.out.println(b.pickTile(n2).getImage());
System.out.println("");
b.checkMatch(n1, n2); b.printBoard();
} else { System.out.println("Invalid Selection!"); }
}
}
}
/** * Tile for the Mind Game. A tile has an image and cabe be placed either * face-up or face-down. */
public class Tile {
private String image; private boolean faceUP;
/** * Constructor for the Tile class. initially, face-down. * * @param word a tile is created with the string word */ public Tile(String word) { // Your code goes here }
/** * Shows image on face-up Tile * * @return image if Tile is face-up; otherwise returns the empty string */ public String showFace() { // Your code goes here }
/** * Checks whether Tile is face-up. * * @return true if Tile is face-up; false otherwise */ public boolean isFaceUp() { // Your code goes here }
/** * Getter method for image * * @return the image string. */ public String getImage() { return image; }
/** * Compares images on Tiles * * @param other image to be compared to image on this tile * @return true if the image on other is the same as this image; false * otherwise */ @Override public boolean equals(Object other) { // Your code goes here // self check // null check // type check and cast // field comparison }
/** * Turns Tile face-up Precondition: Tile is turned face-up */ public void turnFaceUp() { // Your code goes here }
/** * Turns Tile face-down Precondition: Tile is turned face-down */ public void turnFaceDown() { // Your code goes here }
}
import java.util.ArrayList; import java.util.Random;
/** * FixdLenStringList defines a list of strings and a string length. All * strings in the FixdLenStringList have the same length. There is no * present number of strings that a FixdLenStringList can hold. */ public class FixdLenStringList {
private final int strLength; private ArrayList availableStrings;
/** * Constructor for FixedLenStringList that initializes strLength ( the * length of the strings in FixdLenStringList's list) and possibleStrings * (FixdLenStringList's list of strings). * * @param len the length of the FixdLenStringList's strings. */ public FixdLenStringList(int len) { strLength = len; availableStrings = new ArrayList(); }
/** * Gets the length of a string in FixdLenStringList * * @return length of the individual the strings in FixdLenStringList */ public int getStringLength() { // Your code goes here }
/** * Returns the string in position x of FixdLenStringList's list * implementation. The first string is in position 0. Precondition: 0 <= x < * availableStrings.length @param x an index * * value in the FixdLenStringList * @return returns the xth entry in FixdLenStringList */ public String getString(int x) { // Your code goes here }
/** * Returns true if the string, key, is found in the FixdLenStringList's list * of strings, false otherwise. * * @param key the string we are searching for * @return true if key is found in FixdLenStringList; false otherwise */ public boolean found(String key) { // Your code goes here }
/** * Adds the string, entry, to FixdLenStringList's list implementation if it * is the correct length and not already in the list. If the string is * already in the list or if the string is not the correct length, it is not * added. * * @param entry string to add to FixdLenStringList */ public void addString(String entry) { // Your code goes here }
/** * Removes and returns a random string entry from the FixdLenStringList's * list of strings. Precondition: the FixdLenStringList's list must not be * empty. * * @return a random string from FixdLenStringList */ public String removeRandomString() { // Your code goes here }
}
fox fox dog dog cow 5 6 7 cow cat 10 11
12 13 14 15
An example run of the mind Game is provided below:
01
23 Choose positions:1 2
pig , cat
01
23 Choose positions:0 1
cat , pig
01
23 Choose positions:0 3
cat , pig
01
23 Choose positions:1 3
pig , pig
0 pig
2 pig Choose positions:0 2
cat , cat
cat pig cat pig
Step by Step Solution
There are 3 Steps involved in it
Step: 1
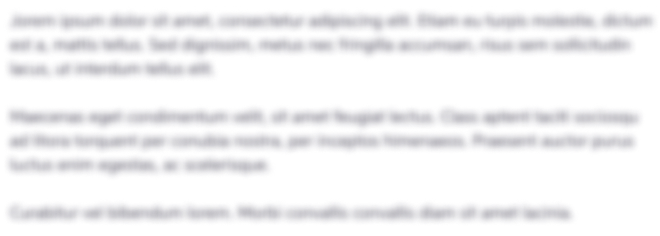
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started