Question
Exercise 1: Adding a Test Harness to the Person class To make it easier to test code that you have written for a Java class
Exercise 1: Adding a Test Harness to the Person class
To make it easier to test code that you have written for a Java class you can add to that class a main method that acts as a "test harness". A test harness is a main method that includes calls to methods that you wish to test. For convention this main method is the last method of the class.
If you have a test harness, you do not need to have a separate application program in order to test your class: the test harness is part of the class you are testing. In this part of the Lab, you will get some experience in writing a test harness.
Open the SocialNetworking project that you created in Lab 1.
We have provided code for a test harness for the Person class, in the file PersonTestHarness.txt. It creates a Person object (this tests the constructor) and prints the result of invoking most of the methods of the class. Add this main method to your Person.java.
Run only Person.java; it will execute the test harness (main method).
Now add code to the test harness to test the equals method: it should print a message like "same friend" if two objects of the class Person are the same according to the equals method documentation (i.e. if they have the same first name and last name), or print "not same friend" if they are not the same. (Hint: you can use friend1 and friend2 to compare two objects of class Person that are not the same, but for thorough testing, you should also create a third Person object who has the same name as one of the first two.)
Run Person.java again to execute the changed test harness.
Make sure you show your results to the TA.
Exercise 2: Adding to the SocialNetwork Class
Add the following two methods to the SocialNetwork class:
getNumFriends(): it will return the number of friends in the list.
clearFriends(): it will remove all friends from the list. (Hint: this can be implemented with just one assignment statement. The SocialNetwork class has an instance variable called numFriends that holds the current number of persons in the list of friends. If you set this to 0 (zero), you do not need to clear out the array numFriends - why not?)
Add a test harness to SocialNetwork.java to test your new methods by doing the following. (Note that this is not a complete test of the class; it will just give you a start in using a test harness in the Lab. You can add more tests later if you wish.)
Create a SocialNetwork object (Hint: see page 40 of the Topic 1 lecture notes)
Add three friends to your social network
Print the contents of your list of friends
Test the getNumFriends method
Test the clearFriends method (how will you show that this worked?)
Make sure you show your results to the TA.
Exercise 3: Using Javadoc comments
Note that the existing comments in Person.java and SocialNetwork.java are Javadoc comments. (You should have read the Introduction to Javadoc document before this Lab.) Add Javadoc comments for the new methods you added to SocialNetwork.java in Exercise 2.
Set up Eclipse to generate documentation for the classes in this Lab, from your Javadoc comments:
Go to Project, Generate Javadoc, ...
For the Javadoc command, click on the Configure button and locate the "javadoc.exe" program. This will be in the "bin" directory of the Java Development Kit installation on the machine. This could for instance be C:\Program Files\Java\jdk1.5.0_03\bin\javadoc.exe
Check the project for which you want to generate the documentation.
Ensure that Public and Use Standard Doclet are selected, and Destination is the default (Z:\{your workspace}\{your project}\doc) and click Finish.
Javadoc generates a tree of html files which comprise the documentation for the program. You should see these in the Package Explorer in Eclipse under the doc directory. You can view them in Eclipse by double clicking (start with index.html).
Make sure you show your results to the TA.
Exercise 4: Using the SocialNetwork Class in an Application
You will now use the SocialNetwork class in an application program. Run the application MyFriends.java that is part of your SocialNetworking project from Lab 1. It is a simple application program that creates and prints a list of friends. (For simplicity, there is no file I/O in this application at present.)
Add statements to MyFriends to do the following:
Call the remove() method to remove one of the friends whose name is already in the list, and report whether or not the remove was successful. Make sure you include the name of the person you removed in the message to the user, for example: "Mickey Mouse was removed successfully."
Call the remove() method for a person whose name is not in the list of friends, and report whether or not the remove was successful.
Print the revised list of contacts.
persontestharness.txt
/** * test harness */ public static void main (String[] args) { // create a friend Person friend1 = new Person("Mickey", "Mouse", ""); friend1.setEmail("mickey@uwo.ca"); System.out.println(friend1); // test accessor methods System.out.println(friend1.getName()); System.out.println(friend1.getEmail()); // create a friend without email Person friend2 = new Person("Minnie", "Mouse", ""); System.out.println(friend2); }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
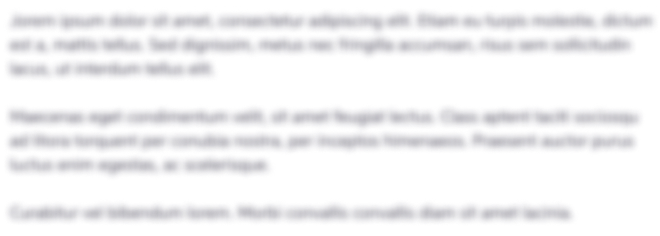
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started