Question
Exercise 1: Create a template class Matrix that has two-dimensional array of any number of rows (with a default of 4 rows) and any number
Exercise 1:
Create a template class Matrix that has two-dimensional array of any number of rows (with a default of 4 rows) and any number of columns (with a default of 4 columns). Matrix should make use of a standard C++, dynamically allocated two-dimensional array to store its elements.
Design, implement and test the Matrix class. Your class should contain at least the following features, namely:
A default constructor and parametrized constructor with two arguments m (the number of rows) and n (the number of columns). It initializes all elements to zeros.
- A destructor.
A copy constructor and an assignment operator =.
A comparison operator ==.
Matrix addition + and subtraction - operators.
Matrix insertion << and extraction >> operators (using row-col format).
- operator () to provide indexing. For example, in a 3-by-5 Matrix called A, the programmer could write A (1,3) to access the element at row 1 and column 3. There should be two versions of operator( ), one that returns int & so an element of a Matrix can be used as an lvalue and one that returns const int & so an element of a Matrix can be used as an rvalue.
- Two versions of operator ++: post and pre increment operators that adds 1 to every element of the matrix.
Note: You must create an array of pointers to implement Matrix.
Class definition and driver is provided in Lab1.cpp file.
Lab1.cpp file:
#include
private: T **arr; int rows; int cols; public: Matrix(int r = 4, int c = 4): rows(r), cols(c) { } Matrix(const Matrix &rhs) {
} Matrix operator=(const Matrix &rhs) {
} bool operator == (const Matrix& rhs) {
}
friend Matrix operator + (const Matrix& m1, const Matrix& m2) { } friend Matrix operator - (const Matrix& m1, const Matrix& m2) { }
friend ostream& operator<<(ostream& outs, const Matrix& rhs) { } friend istream& operator>>(istream& ins, Matrix& rhs) { }
T& operator () (const int r_index, const int c_index) { } const T& operator () (const int r_index, const int c_index) const { } Matrix& operator++(){ // Pre increment. } Matrix operator++(int)//post increment { } ~Matrix() { } };
int main() {
Matrix
//Testing addition + and subtraction - operators. cout << "Testing D + A = : " << d + a; cout << "Testing D - A = : " << d - a; cout << endl;
//Testing comparison operator = cout << "Testing A == A = " << (a == a) << endl; cout << "Testing A == B = " << (a == b) << endl;
//Testing operator () cout << "Testing cout << A[index] "; int index1, index2; cout << "Enter row index : "; cin >> index1; cout << "Enter column index : "; cin >> index2; cout << "A[" << index1 << "," << index2 << "] = " << a(index1, index2) << endl; cout << "Enter new value : "; int value; cin >> value; a(index1, index2) = value; cout << "A[" << index1 << "," << index2 << "] = " << a(index1, index2) << endl; cout << "New values of a : " << a; cout<<"Post increment A:"<
Step by Step Solution
There are 3 Steps involved in it
Step: 1
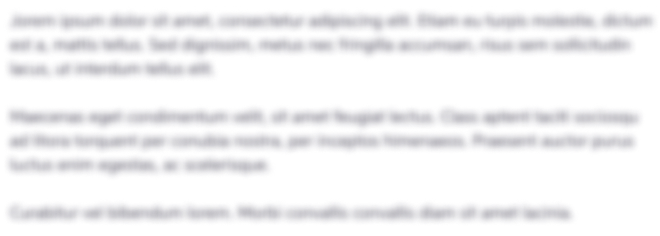
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started