Question
Exercise 1.1: Write a function named largest_even() that takes in 3 arguments: x, y and z. The function's purpose is to find the largest EVEN
Exercise 1.1:
Write a function named largest_even() that takes in 3 arguments: x, y and z. The function's purpose is to find the largest EVEN number among the input and return it as the function output.
If none of them is even, return -1.
Note: The use of the max() function is not allowed! Please use the if, elif, and else statements!
Hint: You might want to take a look at your solution or the instructor's solution for Ex 3.1 in Lab 02 before you attempt this exercise!
In [ ]:
# define the function
def largest_even(x, y, z):
Use the following test cases to test your code (note that the test cases used for grading might be similar to the ones listed here, but might not be exactly the same):
In [ ]:
largest_even(1, 2, 3) #expected output: 2
In [ ]:
largest_even(1, 5, 3) #expected output: -1
In [ ]:
largest_even(9, 9, 4) #expected output: 4
In [ ]:
largest_even(4, 1, 8) #expected output: 8
In [ ]:
largest_even(4, 14, 8) #expected output: 14
2. List
If you have not watched the Python List video [YouTube] [Media Space], please do so before start this section!
2.1 List indexing
Like strings, list has index and elements of a list can be accessed through indexing. For example,
In [1]:
a_list = [1, 4, 15, 19]
a_list
Out[1]:
[1, 4, 15, 19]
In [2]:
a_list[0]
Out[2]:
1
In [3]:
a_list[len(a_list)-1]
Out[3]:
19
Exercise 2.1
Return the 3rd element of the list a_list.
In [ ]:
# your code here
Exercise 2.2
Return the second to last element of the list a_list.
In [7]:
# your code here
2.2 List slicing
Not only can you access a single element of the list, you can also access a block of elements at the same time using slicing!
In [4]:
a_list[0:2]
Out[4]:
[1, 4]
Just like slicing with strings, the syntax is [start:end]. The returned list contains elements starting at index start and ending at index end - 1.
Exercise 2.3
Return all elements of the list except the last one.
In [ ]:
# Write your code here
2.3 Adding elements to a list
To add a new element to the end of a list, use append() function.
In [5]:
a_list.append(43)
a_list
Out[5]:
[1, 4, 15, 19, 43]
To insert a new element at a specific position in the list, use insert() function.
In [6]:
# add an element at the beginning
a_list.insert(0, 23)
a_list
Out[6]:
[23, 1, 4, 15, 19, 43]
In [7]:
# add an element between the 2nd and 3rd elements
a_list.insert(2, 69)
a_list
Out[7]:
[23, 1, 69, 4, 15, 19, 43]
Exercise 2.4
Use two different methods to add number 107 to the end of the list a_list.
Hint: One method is to use the append() function. The other method is to use the insert() function.
Notes: Please run the following cells exactly once (to get the correct output)! If you accidentally run a cell more than once, please re-run all the cells from section 2.1 forwards OR click Kernel menu tab, select Restart & Run All.
In [ ]:
# Method 1
In [ ]:
# Method 2
In [ ]:
# Check the result (by printing out the resulting list)
a_list
Step by Step Solution
There are 3 Steps involved in it
Step: 1
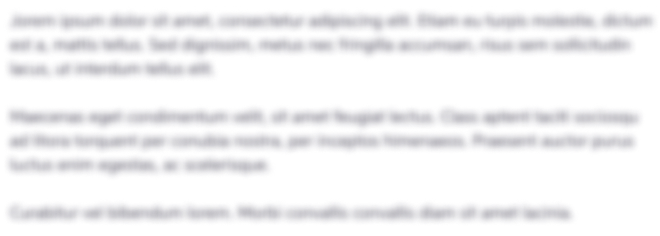
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started