Question
Exercise 16-4 Throw and catch custom exceptions In this exercise, youll modify the Customer Manager application so it handles exceptions differently. This application is similar
Exercise 16-4 Throw and catch custom exceptions
In this exercise, youll modify the Customer Manager application so it handles exceptions differently. This application is similar to the Product Manager application described in chapter 17.
Review the application
1. Open the project named ch16_ex4_CustomerManager in the extra_ex_starts directory.
2. Open the CustomerTextFile class and the Main class and review the code. Note that the CustomerTextFile class handles all I/O exceptions, and the Main class doesnt catch any exceptions.
3. Run the application to make sure it works correctly.
Cause an exception to be thrown
4. In the CustomerTextFile class, add the following code to the beginning of the try block thats in the getCustomers method:
if (true) {
throw new IOException("Test");
}
5.Run the application and test the exception handling. At this point, you should be able to test the exception handling for the list, add, and del commands. For these commands, the application should throw a NullPointerException and display a message like this:
java.io.IOException: Test
Create a custom exception and throw it
6. In the murach.io package, add a class that defines a custom exception named DBException. This exception should allow for exception chaining.
7. In the CustomerTextFile class, modify the getCustomers method so that the catch block creates a DBException object that stores the IOException object and throws the DBException object.
8. Add a throws clause that throws a DBException to all of the methods in the CustomerTextFile that need it.
Catch the custom exception
9. In the Main class, add a try/catch statement that catches any DBExceptions that are thrown by the CustomerTextFile class. In the catch clauses, display a user-friendly message.
10. Run the application and enter the list command. When you do, it should display a message like this:
Error! Unable to read customer file.
11.Use the add command to attempt to add a customer named John Doe. When you do, the application should display a message like this:
Error! Unable to add John Doe.
12.Use the del command to try to delete a customer for an email that exists in the file, the application should display a message like this:
Error! Unable to delete customer with email of johndoe @gmai.com.
Remove the code that causes an exception to be thrown
13.In the CustomerTextFile class, comment out the statement that throws the IOException.
14.Run the application to make sure it works correctly.
THE FOLLOWING CODE HAS ALREADY BEEN GIVEN
package murach.business;
public class Customer {
private String firstName; private String lastName; private String email;
public Customer() { this("", "", ""); }
public Customer(String firstName, String lastName, String email) { this.firstName = firstName; this.lastName = lastName; this.email = email; }
public void setFirstName(String firstName) { this.firstName = firstName; }
public String getFirstName() { return firstName; }
public void setLastName(String lastName) { this.lastName = lastName; }
public String getLastName() { return lastName; }
public void setEmail(String email) { this.email = email; }
public String getEmail() { return email; }
public String getName() { return firstName + " " + lastName; } }
AND
package murach.io;
import java.util.*; import java.io.*; import java.nio.file.*; import murach.business.Customer;
public class CustomerTextFile { private static final String FIELD_SEP = "\t"; private static final Path customersPath = Paths.get("customers.txt"); private static final File customersFile = customersPath.toFile(); private static List
// prevent instantiation of the class private CustomerTextFile() {}
public static List
try (BufferedReader in = new BufferedReader( new FileReader(customersFile))) { // read all customers stored in the file // into the array list customers = new ArrayList<>(); String line; while ((line = in.readLine()) != null) { String[] columns = line.split(FIELD_SEP); String firstName = columns[0]; String lastName = columns[1]; String email = columns[2];
Customer p = new Customer( firstName, lastName, email);
customers.add(p); } return customers; } catch (IOException e) { System.out.println(e); return null; } }
public static Customer getCustomer(String email) { customers = getCustomers(); for (Customer c : customers) { if (c.getEmail().equals(email)) { return c; } } return null; }
public static boolean addCustomer(Customer c) { customers = getCustomers(); customers.add(c); return saveCustomers(); }
public static boolean deleteCustomer(Customer c) { customers = getCustomers(); customers.remove(c); return saveCustomers(); }
private static boolean saveCustomers() { try (PrintWriter out = new PrintWriter( new BufferedWriter( new FileWriter(customersFile)))) {
// write all customers in the array list // to the file for (Customer c : customers) { out.print(c.getFirstName() + FIELD_SEP); out.print(c.getLastName() + FIELD_SEP); out.println(c.getEmail()); } } catch (IOException e) { System.out.println(e); return false; }
return true; } }
AND
package murach.ui;
import java.util.Scanner;
public class Console {
private static Scanner sc = new Scanner(System.in); public static void println() { System.out.println(); }
public static void println(String string) { System.out.println(string); }
public static String getString(String prompt) { System.out.print(prompt); String s = sc.nextLine(); // read the whole line return s; }
public static int getInt(String prompt) { boolean isValid = false; int i = 0; while (isValid == false) { System.out.print(prompt); try { i = Integer.parseInt(sc.nextLine()); isValid = true; } catch (NumberFormatException e) { System.out.println("Error! Invalid integer value. Try again."); } } return i; }
public static int getInt(String prompt, int min, int max) { int i = 0; boolean isValid = false; while (isValid == false) { i = getInt(prompt); if (i <= min) { System.out.println( "Error! Number must be greater than " + min); } else if (i >= max) { System.out.println( "Error! Number must be less than " + max); } else { isValid = true; } } return i; }
public static double getDouble(String prompt) { boolean isValid = false; double d = 0; while (isValid == false) { System.out.print(prompt); try { d = Double.parseDouble(sc.nextLine()); isValid = true; } catch (NumberFormatException e) { System.out.println("Error! Invalid decimal value. Try again."); } } return d; }
public static double getDouble(String prompt, double min, double max) { double d = 0; boolean isValid = false; while (isValid == false) { d = getDouble(prompt); if (d <= min) { System.out.println( "Error! Number must be greater than " + min); } else if (d >= max) { System.out.println( "Error! Number must be less than " + max); } else { isValid = true; } } return d; } }
AND
package murach.ui;
import java.util.List;
import murach.business.Customer; import murach.io.CustomerTextFile;
public class Main {
public static void main(String[] args) { // display a welcome message System.out.println("Welcome to the Customer Manager ");
// display the command menu displayMenu();
// perform 1 or more actions String action; while (true) { // get the input from the user action = Console.getString("Enter a command: "); System.out.println();
if (action.equalsIgnoreCase("list")) { displayAllCustomers(); } else if (action.equalsIgnoreCase("add")) { addCustomer(); } else if (action.equalsIgnoreCase("del") || action.equalsIgnoreCase("delete")) { deleteCustomer(); } else if (action.equalsIgnoreCase("help") || action.equalsIgnoreCase("menu")) { displayMenu(); } else if (action.equalsIgnoreCase("exit") || action.equalsIgnoreCase("quit")) { quit(); } else { System.out.println("Error! Not a valid command. "); } } }
public static void displayMenu() { System.out.println("COMMAND MENU"); System.out.println("list - List all customers"); System.out.println("add - Add a customer"); System.out.println("del - Delete a customer"); System.out.println("help - Show this menu"); System.out.println("exit - Exit this application "); }
public static void displayAllCustomers() { System.out.println("CUSTOMER LIST");
List
public static void addCustomer() { String firstName = Console.getString("Enter first name: "); String lastName = Console.getString("Enter last name: "); String email = Console.getString("Enter customer email: ");
Customer customer = new Customer(); customer.setFirstName(firstName); customer.setLastName(lastName); customer.setEmail(email); CustomerTextFile.addCustomer(customer);
System.out.println(); System.out.println(firstName + " " + lastName + " has been added. "); }
public static void deleteCustomer() { String email = Console.getString("Enter email to delete: ");
Customer c = CustomerTextFile.getCustomer(email);
System.out.println(); if (c != null) { CustomerTextFile.deleteCustomer(c); System.out.println(c.getName() + " has been deleted. "); } else { System.out.println("No customer matches that email. "); } } public static void quit() { System.out.println("Bye. "); System.exit(0); } }
AND
package murach.ui;
public class StringUtils {
public static String padWithSpaces(String s, int length) { if (s.length() < length) { StringBuilder sb = new StringBuilder(s); while (sb.length() < length) { sb.append(" "); } return sb.toString(); } else { return s.substring(0, length); } } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
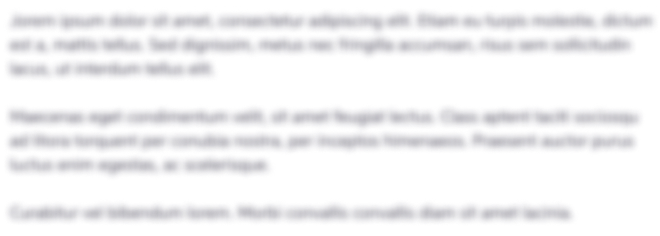
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started