Question
Exercise #2 Complete the following method string compareArea(Planet &p2) : Return the name of the biggest planet , between the target and parameter, based on
Exercise #2
Complete the following method string compareArea(Planet &p2): Return the name of the biggest planet, between the target and parameter, based on the area
NOTE: Remember to constantly verify the Planet class to see if there anything that can help with the solution.
For example:
Answer:(penalty regime: 0 %) | |||||
---|---|---|---|---|---|
Answer:(penalty regime: 0 %)
NOTE: Remember to constantly verify the Planet class to see if there anything that can help with the solution.
/*.......................................................................................................................................................................................................*/
/*Planet.h*/
#include
#include
#include
using namespace std;
class Planet{
private:
string name;
long population;
bool isHabitable;
double diameter;
bool hasWater;
string closestPlanet;
string galaxy;
/*
Area of the Planet
Furmula: area = 4 * pi * r^2
*/
double calculateArea(){
return (2.0 * acos(0.0)) * 4 * pow((getDiameter()/2), 2);
}
public:
//Constructor
Planet(string name, long population, bool isHabitable, double diameter, bool hasWater,
string closestPlanet, string galaxy){
this->name = name;
this->population = population;
this->isHabitable = isHabitable;
this->diameter = diameter;
this->hasWater = hasWater;
this->closestPlanet = closestPlanet;
this->galaxy = galaxy;
}
//Copy constructor
Planet(Planet &p2){
this->name = p2.name;
this->population = p2.population;
this->isHabitable = p2.isHabitable;
this->diameter = p2.diameter;
this->closestPlanet = p2.closestPlanet;
this->hasWater = p2.hasWater;
this->galaxy = p2.galaxy;
}
//Default Constructor
Planet();
//Getters
string getName(){return name;}
long getPopulation(){ return population;}
bool getIsHabitable(){return isHabitable;}
double getDiameter(){return diameter;}
double getArea(){return calculateArea();}
bool getHasWater(){return hasWater;}
string getClosestPlanet(){return closestPlanet;}
string getGalaxy(){return galaxy;}
//Setters
void setName(string name){this->name = name;}
void setPopulation(long population){this->population = population;}
void setIsHabitable(bool isHabitable){this->isHabitable = isHabitable;}
void setDiameter(double diameter){this->diameter = diameter;}
void setHasWater(bool hasWater){this->hasWater = hasWater;}
void setClosestPlanet(string closestPlanet){this->closestPlanet = closestPlanet;}
void setGalaxy(string galaxy){this->galaxy = galaxy;}
string toString(){
return "{Name: " + getName() + ","
+ "Population: " + to_string(getPopulation()) + ", "
+ (getIsHabitable()?"Habitable: True":"Habitable: False") + ", "
+ "Diameter: " + to_string(getDiameter()) + ", "
+ (getHasWater()?"Water: True":"Water: False") + ", "
+ "Closest Planet: " + getClosestPlanet() + ", "
+ "Galaxy: " + getGalaxy() + "}";
}
//PART #1 EXERCISES
string compareArea(Planet &p2);
double volumePlanet();
bool sameGalaxy(Planet &p2);
};
/*.................................................................................................................................................................*/
/*planet.cpp*/
#include "Planet.h"
/* EXERCISE #1
Finish the declaration of the default constructor
When a Planet is initially created it has the following default values:
Name: KNOWHERE
Population: 0
It is NOT habitable
Diameter: 8975
Closet Planet: XANDAR
It DOES HAVE water
Galaxy: GUARDIANS
*/
Planet::Planet() {
// YOUR CODE HERE
}
/*
EXERCISE #2
Return the name of biggest planet (between the target and parameter) based on area
*/
string Planet::compareArea(Planet& p2){
// YOUR CODE HERE
return "LOL";
}
/*
EXERCISE #3
Return the volume of the target planet
Formula: (4*pi*r^3)/3
*/
double Planet::volumePlanet(){
// YOUR CODE HERE
return -99.9;
}
/*
EXERCISE #4
Return true if the target planet and the parameter planet are in the same galaxy
False otherwise.
HINT: use the compare method of string
*/
bool Planet::sameGalaxy(Planet &p2){
// YOUR CODE HERE
return false;
}
/*.................................................................................................................................................................*/
#include "Planet.h"
/* EXERCISE #2 Return the name of biggest planet (between the target and parameter) based on area */ string Planet::compareArea(Planet& p2){ // YOUR CODE HERE return "LOL"; }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
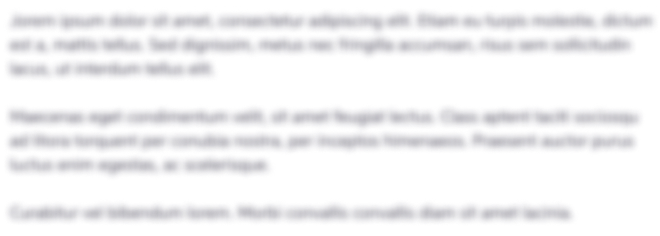
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started