Question
Expand and look at the attached T2-Mod.zip Juice Machine Program. Make sure you can run the program as is before proceeding. Enhance the program to
Expand and look at the attached T2-Mod.zip Juice Machine Program. Make sure you can run the program as is before proceeding. Enhance the program to meet the following new requirements in C++ or Java: 1. Add Dispensers for the following product-price pairs: Juices: CranApple 85 cents; CranGrape 75 cents; Snacks: Crackers 65 cents; Chips 50 cents; 2. Machine must start with $5 dollars in change at the start of the program and it must keep track of how much money it contains from purchases and change given. 3. Machine mush keep accepting currency until the item cost is covered or the user cancels the purchase. If the purchase it cancelled then the money deposited by that user needs to be returned. 4. Change money collection to only accept standard currency: nickels (5 cents), dimes (10 cents) quarters (25 cents), half-dollars (50 cents), dollar bills/dollar coin ($1) and 5 dollar bills ($5). Largest bill accepted is $5 bill. $2 bills are optional. 5. The machine must give change if too much money was deposited and notify the user before dispensing if it does not have enough money to make change. Write a report to file at the completion of the program that shows what was sold, the status of each dispenser, and how much currency the machine contains. 7. Let the user
#include
using namespace std; class cashRegister { friend ostream& operator<<(ostream&, const cashRegister&); public: int getCurrentBalance() const;
cashRegister operator+(int& amount) const; cashRegister operator-(int& amount) const;
bool operator==(const cashRegister& cashR) const; bool operator!=(const cashRegister& cashR) const; bool operator<=(const cashRegister& cashR) const; bool operator<(const cashRegister& cashR) const; bool operator>=(const cashRegister& cashR) const; bool operator>(const cashRegister& cashR) const;
cashRegister(int cashIn = 500);
private: int cashOnHand; //variable to store the cash //in the register };
#include
using namespace std;
int cashRegister::getCurrentBalance() const { return cashOnHand; }
cashRegister::cashRegister(int cashIn) { if (cashIn >= 0) cashOnHand = cashIn; else cashOnHand = 500; }
cashRegister cashRegister::operator+(int& amount) const { cashRegister temp;
temp.cashOnHand = cashOnHand + amount;
return temp;
}
cashRegister cashRegister::operator-(int& amount) const { cashRegister temp;
temp.cashOnHand = cashOnHand - amount;
return temp;
}
bool cashRegister::operator==(const cashRegister& cashR) const { return (cashOnHand == cashR.cashOnHand); } bool cashRegister::operator!=(const cashRegister& cashR) const { return (cashOnHand != cashR.cashOnHand); } bool cashRegister::operator<=(const cashRegister& cashR) const { return (cashOnHand <= cashR.cashOnHand); }
bool cashRegister::operator<(const cashRegister& cashR) const { return (cashOnHand < cashR.cashOnHand); }
bool cashRegister::operator>=(const cashRegister& cashR) const { return (cashOnHand >= cashR.cashOnHand); }
bool cashRegister::operator>(const cashRegister& cashR) const { return (cashOnHand > cashR.cashOnHand); }
ostream& operator<<(ostream& os, const cashRegister& cashR) { os << cashR.cashOnHand;
return os; }
#include
void showSelection(); void sellProduct(dispenserType& product, cashRegister& pCounter);
int main() { cashRegister counter; dispenserType orange(60, 100); dispenserType apple(70, 100); dispenserType mango(80, 45); dispenserType strawberryBanana(100, 85);
int choice; //variable to hold the selection
showSelection(); cin >> choice;
while (choice != 9) { switch (choice) { case 1: sellProduct(orange, counter); break; case 2: sellProduct(apple, counter); break; case 3: sellProduct(mango, counter); break; case 4: sellProduct(strawberryBanana, counter); break; default: cout << "Invalid selection." << endl; }//end switch
showSelection(); cin >> choice; }//end while
return 0; }//end main
void showSelection() { cout << "*** Welcome to Shelly's Juice Shop ***" << endl; cout << "To select an item, enter " << endl; cout << "1 for orange" << endl; cout << "2 for mango" << endl; cout << "3 for strawberry banana" << endl; cout << "4 for Cookies" << endl; cout << "9 to exit" << endl; }//end showSelection
void sellProduct(dispenserType& product, cashRegister& pCounter) { int amount; //variable to hold the amount entered int amount2; //variable to hold the extra amount needed
if (product.getNoOfItems() > 0) //if the dispenser is not //empty { cout << "Please deposit " << product.getCost() << " cents" << endl; cin >> amount;
if (amount < product.getCost()) { cout << "Please deposit another " << product.getCost()- amount << " cents" << endl; cin >> amount2; amount = amount + amount2; }
if (amount >= product.getCost()) { pCounter = pCounter + amount; //.acceptAmount(amount); product--; //product.makeSale(); cout << "Collect your item at the bottom and " << "enjoy." << endl; } else cout << "The amount is not enough. " << "Collect what you deposited." << endl;
cout << "*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*-*" << endl << endl; } else cout << "Sorry, this item is sold out." << endl; }//end sellProduct
#include
using namespace std; class dispenserType { friend ostream& operator<<(ostream&, const dispenserType&); public: int getNoOfItems() const;
int getCost() const;
void makeSale();
dispenserType operator++(); dispenserType operator++(int); dispenserType operator--(); dispenserType operator--(int);
dispenserType(int setNoOfItems = 50, int setCost = 50);
private: int numberOfItems; //variable to store the number of //items in the dispenser int cost; //variable to store the cost of an item };
//********************************************************** // Author: D.S. Malik // // Implementation file dispenserType.cpp // This file contains the definitions of the functions to // implement the operations of the class dispenserType. //********************************************************** #include
using namespace std;
int dispenserType::getNoOfItems() const { return numberOfItems; }
int dispenserType::getCost() const { return cost; }
dispenserType dispenserType::operator++() { numberOfItems++;
return *this; }
dispenserType dispenserType::operator++(int u) { dispenserType temp = *this;
numberOfItems++;
return temp; }
dispenserType dispenserType::operator--() { numberOfItems--;
return *this; }
dispenserType dispenserType::operator--(int u) { dispenserType temp = *this;
numberOfItems--;
return temp; }
dispenserType::dispenserType(int setNoOfItems, int setCost) { if (setNoOfItems >= 0) numberOfItems = setNoOfItems; else numberOfItems = 50;
if (setCost >= 0) cost = setCost; else cost = 50; }
ostream& operator<<(ostream& os, const dispenserType& disp) { os << "Cost: " << disp.cost << ", quantity " << disp.numberOfItems;
return os; }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
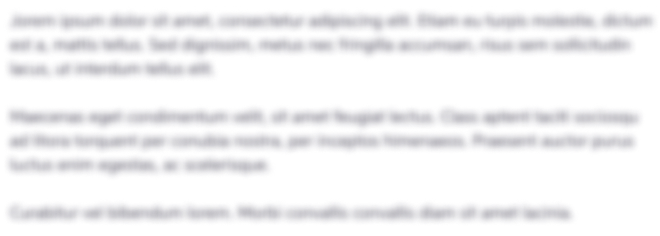
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started