Question
EXPERIMENT 2 (Java Programming) *For the one who said the question needs more info, the programs have to be written in JAVA, the C++ CODE
EXPERIMENT 2 (Java Programming)
*For the one who said the question needs more info, the programs have to be written in JAVA, the "C++ CODE" is only to give you an "idea" of how to do. So the 4 codes have wrtitten in Java. Thank you.*
NAME OF THE EXPERIMENT: Simulate the following CPU Scheduling Algorithms
a) FCFS b) SJF c) Round Robin d) Priority --> Task for each: Write the code using java (a, b, c, d).
AIM: Using CPU scheduling algorithms find the min & max waiting time.
SOFTWARE REQUIREMENTS:
Java
THEORY:
CPU SCHEDULING
Maximum CPU utilization obtained with multiprogramming
CPUI/O Burst Cycle Process execution consists of a cycle of
CPU execution and I/O wait
CPU burst distribution
Experiment: 2 a)
First-Come, First-Served (FCFS) Scheduling
Process Burst Time
P1 24
P2 3
P3 3
Suppose that the processes arrive in the order: P1 , P2 , P3
The Gantt Chart for the schedule is:
ALGORITHM 1. Start 2. Declare the array size 3. Read the number of processes to be inserted 4. Read the Burst times of processes 5. calculate the waiting time of each process wt[i+1]=bt[i]+wt[i] 6. calculate the turnaround time of each process tt[i+1]=tt[i]+bt[i+1] 7. Calculate the average waiting time and average turnaround time. 8. Display the values 9. Stop
PROGRAM: #include #include void main() { int i,j,bt[10],n,wt[10],tt[10],w1=0,t1=0; float aw,at; clrscr(); printf("enter no. of processes: "); scanf("%d",&n); printf("enter the burst time of processes:"); for(i=0;i scanf("%d",&bt[i]); for(i=0;i { wt[0]=0; tt[0]=bt[0]; wt[i+1]=bt[i]+wt[i]; tt[i+1]=tt[i]+bt[i+1]; w1=w1+wt[i]; t1=t1+tt[i]; } aw=w1; at=t1; printf(" bt\t wt\t tt "); for(i=0;i printf("%d\t %d\t %d ",bt[i],wt[i],tt[i]); printf("aw=%f ,at=%f ",aw,at); getch(); }
INPUT Enter no of processes 3 enter bursttime 12 8 20
EXPECTED OUTPUT bt wt tt 12 0 12 8 12 20 20 20 40 aw=10.666670 at=24.00000
EXPERIMENT : 2 b) NAME OF THE EXPERIMENT: Simulate the following CPU Scheduling Algorithms
b) SJF AIM: Using CPU scheduling algorithms find the min & max waiting time.
SOFTWARE REQUIREMENTS: Java
THEORY: Example of Non Preemptive SJF Process Arrival Time Burst Time P1 0.0 7 P2 2.0 4 P3 4.0 1 P4 3.0 4
Example of Preemptive SJF Process Arrival Time Burst Time P1 0.0 7 P2 2.0 4 P3 4.0 1 P4 3.0 4
ALGORITHM 1. Start 2. Declare the array size 3. Read the number of processes to be inserted 4. Read the Burst times of processes 5. sort the Burst times in ascending order and process with shortest burst time is first executed. 6. calculate the waiting time of each process wt[i+1]=bt[i]+wt[i] 7. calculate the turnaround time of each process tt[i+1]=tt[i]+bt[i+1] 8. Calculate the average waiting time and average turnaround time. 9. Display the values 10. Stop
PROGRAM: #include #include void main() { int i,j,bt[10],t,n,wt[10],tt[10],w1=0,t1=0; float aw,at; clrscr(); printf("enter no. of processes: "); scanf("%d",&n); printf("enter the burst time of processes:"); for(i=0;i scanf("%d",&bt[i]); for(i=0;i { for(j=i;j if(bt[i]>bt[j]) { t=bt[i]; bt[i]=bt[j]; bt[j]=t; } } for(i=0;i printf("%d",bt[i]); for(i=0;i { wt[0]=0; tt[0]=bt[0]; wt[i+1]=bt[i]+wt[i]; tt[i+1]=tt[i]+bt[i+1]; w1=w1+wt[i]; t1=t1+tt[i]; } aw=w1; at=t1; printf(" bt\t wt\t tt "); for(i=0;i printf("%d\t %d\t %d ",bt[i],wt[i],tt[i]); printf("aw=%f ,at=%f ",aw,at); getch(); }
INPUT: enter no of processes 3 enter burst time 12 8 20
OUTPUT: bt wt tt 12 8 20 8 0 8 20 20 40 aw=9.33 at=22.64
EXPERIMENT : 2c)
NAME OF THE EXPERIMENT: Simulate the following CPU Scheduling Algorithms
c) Round Robin
AIM: Using CPU scheduling algorithms find the min & max waiting time.
SOFTWARE REQUIREMENTS:
Java
THEORY:
Round Robin:
Example of RR with time quantum=3
Process | Burst time |
aaa | 4 |
Bbb | 3 |
Ccc | 2 |
Ddd | 5 |
Eee | 1 |
ALGORITHM 1. Start 2. Declare the array size 3. Read the number of processes to be inserted 4. Read the burst times of the processes 5. Read the Time Quantum 6. if the burst time of a process is greater than time Quantum then subtract time quantum form the burst time Else Assign the burst time to time quantum. 7.calculate the average waiting time and turn around time of the processes. 8. Display the values 9. Stop PROGRAM: #include #include void main() { int st[10],bt[10],wt[10],tat[10],n,tq; int i,count=0,swt=0,stat=0,temp,sq=0; float awt=0.0,atat=0.0; clrscr(); printf("Enter number of processes:"); scanf("%d",&n); printf("Enter burst time for sequences:"); for(i=0;i { scanf("%d",&bt[i]); st[i]=bt[i]; } printf("Enter time quantum:"); scanf("%d",&tq); while(1) { for(i=0,count=0;i { temp=tq; if(st[i]==0) { count++; continue; } if(st[i]>tq) st[i]=st[i]-tq; else if(st[i]>=0) { temp=st[i]; st[i]=0; } sq=sq+temp; tat[i]=sq; } if(n==count) break; } for(i=0;i { wt[i]=tat[i]-bt[i]; swt=swt+wt[i]; stat=stat+tat[i]; } awt=(float)swt; atat=(float)stat; printf("Process_no Burst time Wait time Turn around time"); for(i=0;i printf(" %d\t %d\t %d\t %d",i+1,bt[i],wt[i],tat[i]); printf(" Avg wait time is %f Avg turn around time is %f",awt,atat); getch(); }
Input: Enter no of jobs 4 Enter burst time 5 12 8 20
Output: Bt wt tt 5 0 5 12 5 13 8 13 25 20 25 45 aw=10.75000 at=22.000000
EXPERIMENT : 2d)
NAME OF THE EXPERIMENT: Simulate the following CPU Scheduling Algorithms
d) Priority
AIM: Using CPU scheduling algorithms find the min & max waiting time.
SOFTWARE REQUIREMENTS:
Java.
THEORY:
In Priority Scheduling, each process is given a priority, and higher priority methods are executed first, while equal priorities are executed First Come First Served or Round Robin.
There are several ways that priorities can be assigned:
Internal priorities are assigned by technical quantities such as memory usage, and file/IO operations.
External priorities are assigned by politics, commerce, or user preference, such as importance and amount being paid for process access (the latter usually being for mainframes)
ALGORITHM
1. Start
2. Declare the array size
3. Read the number of processes to be inserted
4. Read the Priorities of processes
5. sort the priorities and Burst times in ascending order
6. calculate the waiting time of each process wt[i+1]=bt[i]+wt[i]
7. calculate the turnaround time of each process tt[i+1]=tt[i]+bt[i+1]
8. Calculate the average waiting time and average turnaround time.
9. Display the values
10. Stop
Input:
Enter no of jobs
4
Enter bursttime
10
2
4
7
Enter priority values
4
2
1
3
Output:
Bt priority wt tt
4 1 0 4
2 2 4 6
7 3 6 13
10 4 13 23
aw=5.750000
at=12.500000
ID Waiting time for P1 -0: P2-24; P3-27 Average waiting time: (0+ 24 + 27)/3 17 24 27 30 ID Waiting time for P1 -0: P2-24; P3-27 Average waiting time: (0+ 24 + 27)/3 17 24 27 30Step by Step Solution
There are 3 Steps involved in it
Step: 1
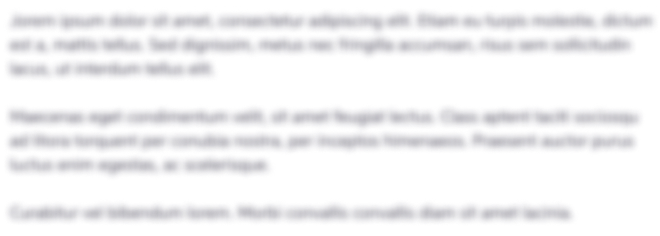
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started