Question
Explain the below code in detail and also write the conclusion. #include #include using namespace std; class ClosedHashing { private: vector hashTable; int hashTableSize; public:
Explain the below code in detail and also write the conclusion. #include #include using namespace std; class ClosedHashing { private: vector hashTable; int hashTableSize; public: ClosedHashing(int size) { hashTable.resize(size); hashTableSize = size; } int hashFunction(int key) { return key % hashTableSize; } int linearProbing(int key) { int index = hashFunction(key); int i = 0; while (hashTable[(index + i) % hashTableSize] != 0) { i++; } return (index + i) % hashTableSize; } int quadraticProbing(int key) { int index = hashFunction(key); int i = 0; while (hashTable[(index + i * i) % hashTableSize] != 0) { i++; } return (index + i * i) % hashTableSize; } int doubleHashing(int key) { int index = hashFunction(key); int i = 0; int h2 = 5 - (key % 4); while (hashTable[(index + i * h2) % hashTableSize] != 0) { i++; } return (index + i * h2) % hashTableSize; } void insertLinearProbing(int key) { int index = linearProbing(key); hashTable[index] = key; } void insertQuadraticProbing(int key) { int index = quadraticProbing(key); hashTable[index] = key; } void insertDoubleHashing(int key) { int index = doubleHashing(key); hashTable[index] = key; } void displayHashTable() { cout << "Hash Table: "; for (int i = 0; i < hashTableSize; i++) { cout << hashTable[i] << " "; } cout << endl; } }; int main() { // Creating object of ClosedHashing ClosedHashing hashTable(10); // Inserting elements using linear probing hashTable.insertLinearProbing(20); hashTable.insertLinearProbing(89); hashTable.insertLinearProbing(60); hashTable.insertLinearProbing(18); hashTable.insertLinearProbing(40); hashTable.insertLinearProbing(49); hashTable.insertLinearProbing(44); hashTable.insertLinearProbing(58); hashTable.insertLinearProbing(88); hashTable.insertLinearProbing(79); // Displaying linear probing hash table cout << "Linear Probing Hash Table: "; hashTable.displayHashTable(); // Creating object of ClosedHashing ClosedHashing hashTable2(10); // Inserting elements using quadratic probing hashTable2.insertQuadraticProbing(20); hashTable2.insertQuadraticProbing(89); hashTable2.insertQuadraticProbing(60); hashTable2.insertQuadraticProbing(18); hashTable2.insertQuadraticProbing(40); hashTable2.insertQuadraticProbing(49); hashTable2.insertQuadraticProbing(44); hashTable2.insertQuadraticProbing(58); hashTable2.insertQuadraticProbing(88); hashTable2.insertQuadraticProbing(79); // Displaying quadratic probing hash table cout << "Quadratic Probing Hash Table: "; hashTable2.displayHashTable(); // Creating object of ClosedHashing ClosedHashing hashTable3(10); // Inserting elements using double hashing hashTable3.insertDoubleHashing(20); hashTable3.insertDoubleHashing(89); hashTable3.insertDoubleHashing(60); hashTable3.insertDoubleHashing(18); hashTable3.insertDoubleHashing(40); hashTable3.insertDoubleHashing(49); hashTable3.insertDoubleHashing(44); hashTable3.insertDoubleHashing(58); hashTable3.insertDoubleHashing(88); hashTable3.insertDoubleHashing(79); // Displaying double hashing hash table cout << "Double Hashing Hash Table: "; hashTable3.displayHashTable(); return 0; }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
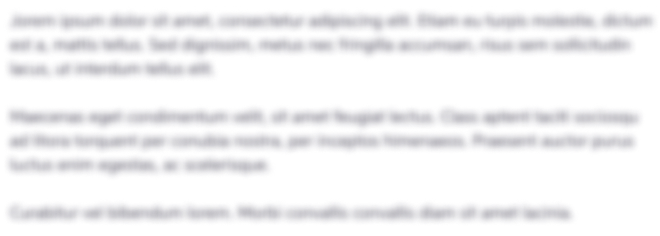
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started