Question
Explore the pythonBasics2.py file you previously completed. Open the file in your preferred IDE (PyCharm, VS Code, Jupyter, ...). Requirements for two of the methods
Explore the pythonBasics2.py file you previously completed.
Open the file in your preferred IDE (PyCharm, VS Code, Jupyter, ...).
Requirements for two of the methods have changed as follows. You must update the methods and comments to accurately represent this new update.
Part A (count_threes) now needs to return the multiple of three that occurs the most in a string. For example, 0939639 would return 9 since it appeared 3 times while the other multiple of three appeared less than that. You only need to worry about single digit multiples of 3 (3, 6, 9). You must use a dictionary to accomplish this.
Part B (longest_consecutive_repeating_char) now needs to account for the edge case where two characters have the same consecutive repeat length. The return value should now be a list containing all characters with the longest consecutive repeat. For example, the longest_consecutive_repeating_char('aabbccd') would return ['a', 'b', 'c'] (order doesn't matter). You must use a dictionary to accomplish this.
Note that our existing test file won't work with these changes. We will be updating that in the next step.
code
# Python Activity # # Fill in the code for the functions below. # The starter code for each function includes a 'return' # which is just a placeholder for your code. Make sure to add what is going to be returned. # Part A. count_threes # Define a function count_threes(n) that takes an int and # returns the number of multiples of 3 in the range from 0 # to n (including n). def count_threes(n): # YOUR CODE HERE return int(n/3) # Part B. longest_consecutive_repeating_char # Define a function longest_consecutive_repeating_char(s) that takes # a string s and returns the character that has the longest consecutive repeat. def longest_consecutive_repeating_char(s): # YOUR CODE HERE n = len(s) ctr = 1 mx = 1 ch = s[0] for i in range(0,n-1): if s[i] ==s[i+1]: ctr += 1 else: if ctr > mx: mx = ctr ch = s[i] ctr = 1 if ctr > mx: mx = ctr ch = s[i] return ch # Part C. is_palindrome # Define a function is_palindrome(s) that takes a string s # and returns whether or not that string is a palindrome. # A palindrome is a string that reads the same backwards and # forwards. Treat capital letters the same as lowercase ones # and ignore spaces (i.e. case insensitive). def is_palindrome(s): # YOUR CODE HERE i = 0 j = len(s)-1 while i <= j: if s[i] == ' ': i += 1 continue if s[j] == ' ': j -= 1 continue if s[i].lower() != s[j].lower(): return False i += 1 j -= 1 return True
TEST FILE
import unittest import pythonBasics2 class TestPythonBasicsTwo(unittest.TestCase): def test_count_threes(self): self.assertEqual(pythonBasics2.count_threes('033'), 3) self.assertEqual(pythonBasics2.count_threes('9369'), 9) self.assertEqual(pythonBasics2.count_threes('999'), 9) self.assertEqual(pythonBasics2.count_threes('30669636'), 6) def test_longest_consecutive_repeating_char(self): # Default cases self.assertEqual(pythonBasics2.longest_consecutive_repeating_char('aaa'), ['a']) self.assertEqual(pythonBasics2.longest_consecutive_repeating_char('abba'), ['b']) self.assertEqual(pythonBasics2.longest_consecutive_repeating_char('caaddda'), ['d']) self.assertEqual(pythonBasics2.longest_consecutive_repeating_char('aaaffftttt'), ['t']) self.assertEqual(pythonBasics2.longest_consecutive_repeating_char('aaababbacccca'), ['c']) self.assertEqual(pythonBasics2.longest_consecutive_repeating_char('ddabab'), ['d']) self.assertEqual(pythonBasics2.longest_consecutive_repeating_char('caac'), ['a']) # Multiple outputs self.assertEqual(set(pythonBasics2.longest_consecutive_repeating_char('caacc')), set(['a', 'c'])) self.assertEqual(set(pythonBasics2.longest_consecutive_repeating_char('bbbaaaceeef')), set(['a', 'b', 'e'])) self.assertEqual(set(pythonBasics2.longest_consecutive_repeating_char('abcddefgghij')), set(['d', 'g'])) self.assertEqual(set(pythonBasics2.longest_consecutive_repeating_char('aabbbccddddefggghhhh')), set(['d', 'h'])) def test_is_palindrome(self): self.assertEqual(pythonBasics2.is_palindrome("Hello"), False) self.assertEqual(pythonBasics2.is_palindrome("civic"), True) self.assertEqual(pythonBasics2.is_palindrome("Civic"), True) self.assertEqual(pythonBasics2.is_palindrome("Racecar"), True) self.assertEqual(pythonBasics2.is_palindrome("Dont nod"), True) self.assertEqual(pythonBasics2.is_palindrome("was it a cat I saw"), True) self.assertEqual(pythonBasics2.is_palindrome("It was not a cat"), False) if __name__ == '__main__': unittest.main(verbosity=1)
Step by Step Solution
There are 3 Steps involved in it
Step: 1
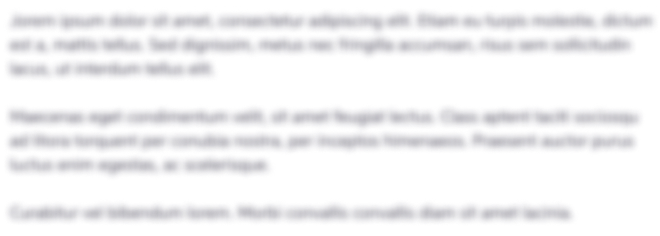
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started