Question
Extend bag ADT by: Add the methods union, intersection, and difference to the bag ADT. The union of two bags is a new bag containing
Extend bag ADT by:
Add the methods union, intersection, and difference to the bag ADT. The union of two bags is a new bag containing the combined contents of the original two bags. The intersection of two bags is a new bag containing the entries that occur in both of the original two bags. The difference of two bags is a new bag containing the entries that would be left in one bag after removing those that also occur in the second.
- Implement union method for class ArrayBag.
- Implement intersect method for class ArrayBag.
- Imlplement difference method for class ArrayBag.
This is what I've done. However it doesn't run. Any help will be aprecciated.
/** @?le BagInterface.h */
#ifndef _BAG_INTERFACE
#define _BAG_INTERFACE
#include
using namespace::std;
template < class ItemType>
class BagInterface
{
public:
/** Gets the current number of entries in this bag.
@return The integer number of entries currently in the bag. */
virtual int getCurrentSize() const = 0;
/** Sees whether this bag is empty.
@return True if the bag is empty, or false if not. */
virtual bool isEmpty() const = 0;
/** Adds a new entry to this bag.
@post If successful, newEntry is stored in the bag and
the count of items in the bag has increased by 1.
@param newEntry The object to be added as a new entry.
@return True if addition was successful, or false if not. */
virtual bool add(const ItemType &newEntry) = 0;
/** Removes one occurrence of a given entry from this bag,
if possible.
@post If successful, anEntry has been removed from the bag
and the count of items in the bag has decreased by 1.
@param anEntry The entry to be removed.
@return True if removal was successful, or false if not. */
virtual bool remove(const ItemType &anEntry) = 0;
/** Removes all entries from this bag.
@post Bag contains no items, and the count of items is 0. */
virtual void clear() = 0;
/** Counts the number of times a given entry appears in bag.
@param anEntry The entry to be counted.
@return The number of times anEntry appears in the bag. */
virtual int getFrequencyOf(const ItemType &anEntry) const = 0;
/** Tests whether this bag contains a given entry.
@param anEntry The entry to locate.
@return True if bag contains anEntry, or false otherwise. */
virtual bool contains(const ItemType &anEntry) const = 0;
/** Empties and then f ills a given vector with all entries that are in this bag.
@return A vector containing all the entries in the bag. */
virtual vector
};// end BagInterface
#endif
//File name: ArrayBag.h
#ifndef _ARRAY_BAG
#define _ARRAY_BAG
#include "BagInterface.h"
#include
//Derived class.
template
class ArrayBag : public BagInterface
{
//Access specier
private:
//Declare and intialize default bag size.
static const int DEFAULTBAG_SIZE = 10;
//Declare an array for items.
ItemType items[DEFAULTBAG_SIZE]; //Array of bag items
//Declare an integer for item count,
int itemCounter;
//Declare an integer for maximum number of items
int maxItems;
//Declare an integer for index of item.
int getIndexOf(const ItemType& targetItem) const;
Union(ArrayBag
difference(ArrayBag
ArrayBag difference(ArrayBag
//Access specier
public:
//Constructor
ArrayBag();
//Function declaration getCurrentSizeo.
int getCurrentSize() const;
//Function declaration is empty()
bool isEmpty() const;
//Function declaration add().
bool add(const ItemType& newEntry);
//Function declaration remove().
bool remove(const ItemType& anEntry);
//Function declaration clear().
void clear();
//Function declaration containso
bool contains(const ItemType& anEntry) const;
//Function declaration getFrequencyOfo.
int getFrequencyOf(const ItemType& anEntry) const;
//Function declaration toVector().
vector
//Function declaration Union().
//Function declaration intersectiono.
};
#endif
//File Name: ArrayBag.cpp
//Include header les
#include "ArrayBag.h"
//Dene a Constructor.
template
ArrayBag
{}
/* Dene function "getCurrentSize0" returns number of items.*/
template
int ArrayBag
{
//Return value.
return itemCounter;
}
//Dene function "isEmpty()" to check whether bag is empty.
template
bool ArrayBag
{
//Return value.
return itemCounter == 0;
}
// Dene function "add()" to check whether items can be added */
template
bool ArrayBag
{
//Declare variable.
bool hasRoom = (itemCounter < maxItems);
//If condition
if (hasRoom)
{
//Add items.
items[itemCounter] = newEntry;
//Increment item count
itemCounter++;
}
return hasRoom;
}//end add
//Dene function "remove()" to remove bag items
template
bool ArrayBag
{
//Declare variable.
int locatedIndex = getIndexOf(anEntry);
//Declare variable.
bool canRemoveItem = !isEmpty() && (locatedIndex > -1);
//If Condition.
if (canRemoveItem)
{//Decrement count.
itemCounter--;
//Delete item.
items[locatedIndex] = items[itemCounter];
}
//Return value.
return canRemove;
}
//Function to clear the contents of the bag.
template
void ArrayBag
{
//Set item count to zero,
itemCounter = 0;
}
//Function returns the frequency of the item in the bag
template
int ArrayBag
{
//Declare and initialize a variable for frequency,
int itemFrequency = 0;
//Declare and initialize a variable for search index,
int searchIndex = 0;
//While Loop().
while (searchIndex < itemCounter)
{
//Condition check.
if (items[searchIndex] == anEntry)
{
//lncrement itemFrequency.
itemFrequency++;
}//end if
//Increment search index
searchIndex++;
}
return itemFrequency;
}
//Function returns the whether the bag has the item or not.
template
bool ArrayBag
{
//Return.
return getIndexOf(anEntry) > -1;
}
//Function to print the contents as vector data
template
vector
{
//Declare a vector for bag items.
vector
//For loop to iterate.
for (int i = 0; i < itemCounter; i++)
//Add items to the bag
bagContents.push_back(items[i]);
//Return the contents of the bag
return bagContents;
}//end toVector
//Function to get the index of the item in the bag.
template
int ArrayBag
{
//Declare and initialize a boolean variable
bool isFound = false;
//Declare and initialize an integer variable,
int result = -1;
//Declare and initialize an integer variable,
int searchIndex = 0;
//While loop
// If the bag is empty, itemCount is zero, so loop is skipped
while (!isFound && (searchIndex < itemCounter))
{
//Condition check.
if (items[searchIndex] == targetItem)
{
//Update the isFound
isFound = true;
//Update the resultant.
result = searchIndex;
}
//Otherwise.
else
{
//Increment search index
searchIndex++;
}//end if
}//end while
//Return
return result;
}
//Function to perform union operation on two bags,
template
ArrayBag
{
//Create a new instance,
ArrayBag
//Get bag 1 items as vector.
vector
/*Use a loop to copy the items from the bag 1 to new bag.*/
for (int index = 0; index < getCurrentSize(); index++)
//Copy the items
newBag.add(myVector1.at(index));
//Get bag2 items as vector.
vector
/*Use a loop to copy the items from the bag 2 to new bag.*/
for (int index = 0; index < myBag2.getCurrentSize(); index++)
//Copy the items
newBag.add(myVector2.at(index));
//return the new bag
return newBag;
}
//Function to perform intersection operation on two bags
template
ArrayBag
{
//Create a new instance,
ArrayBag
//Use a vector to get the bag 1 items,
vector
//Use a vector to get the bag 2 items,
vector
//Use a for loop to process the items,
for (int index = 0; index < getCurrentSize(); index++)
{
/*Check whether the items at the index is present in the bag*/
if (myBag2.contains(myVector1.at(index))) {
//On success, add that item to the new bag
newBag.add(myVector1.at(index));
}
//Return the newBag.
return newBag;
}
//Function to perform difference operation on two bags,
template
ArrayBag
{
//Create a new instance,
ArrayBag
//Use a vector to get the bag 1 items,
vector
//Use a vector to get the bag 2 items,
vector
//Use a for loop to process the items,
for (int index = 0; index < getCurrentSize(); index++)
{
/*Check whether the item at index is not present in bag 2,*/
if (!myBag2.contains(myVector1.at(index)))
//On success, add this item to the new bag.
newBag.add(myVector1.at(index));
}
//return newBag
return newBag;
}//end difference
//test.cpp
#include
#include "ArrayBag.cpp"
#include "BagInterface.h"
#include
#include
//Use std namespace
using namespace std;
// Dene function "printContentsOfBag() to display bag contents.
void printContentsOfBag(ArrayBag
{
//Display message.
cout << "The bag has " << myBag1.getCurrentSize() << " items:" << endl;
//Display bag contents
vector
//Get item count
int numberOfEntries = (int)bagItems1.size();
//Loop until item count
for (int i = 0; i < numberOfEntries; i++)
{
//Display Dag items
cout << bagItems1[i] << " ";
}//end for
}//end diaplay bag
//Dene function "bagTester()" to test bag,
void bagTester(ArrayBag
{
cout << "isEmpty: returns " << myBag1.isEmpty()
<< ";should be 1 (true)" << endl;
printContentsOfBag(myBag1);
//Create string items array,
string bagItems1[] = { "one", "two", "three", "four", "five", "one" };
cout << "Add 6 items to the bag: " << endl;
//Loop until length
for (int i = 0; i <= 6; i++)
{//Add items to bag.
myBag1.add(bagItems1[i]);
}//end for
/* Call function "printContentsOfBago" to display bag itemsf*/
printContentsOfBag(myBag1);
cout << "isEmpty: returns " << myBag1.isEmpty() << "; should be 0 (false)" << endl;
cout << "getCurrentSize: returns " << myBag1.getCurrentSize() << "; should be 6" << endl;
cout << "Try to add another entry: add(\"extra\") returns " << myBag1.add("extra") << endl;
} // end bagTester
//Dene main method
int main()
{
//Create a new instance,
ArrayBag
//Call function "bagTester()".
bagTester(myBag1);
//Display new line,
cout << endl << endl;
//Create new instance.
ArrayBag
//Dene array,
string bagItems12[] = { "b","c","d","e" };
//Loop until length.
for (int i = 0; i <= 4; i++)
{//Add items to bag.
myBag2.add(bagItems12[i]);
}
// Call function "printContentsOfBag()" to display bag contents.
printContentsOfBag(myBag2);
//Call function "union()".
ArrayBag
// Call function "printContentsOfBag()" to display bag contents.
printContentsOfBag(myBag3);
//Call method intersection().
ArrayBag
//Display bag.
printContentsOfBag(myBag4);
//Call method "difference()":
ArrayBag
//Display bag.
printContentsOfBag(myBag5);
//Pause console window
system("pause");
//Return 0
return 0;
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
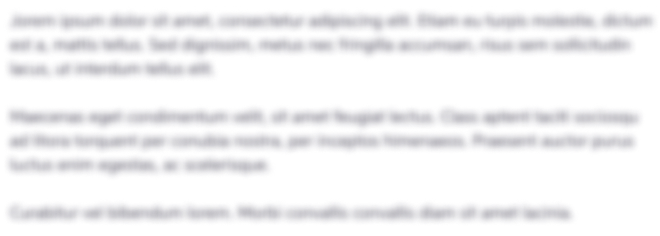
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started