Answered step by step
Verified Expert Solution
Question
1 Approved Answer
Extract the contents of the zip file ( 8 file contents below ) Test your code with the supplied JUnit test cases. They are by
Extract the contents of the zip file file contents below
Test your code with the supplied JUnit test cases. They are by no means comprehensive. You can write your own test cases.
Complete all the TODO sections in this source file BSTreeMap.java.
Zip only BSTreeMap.java into a file called hwzip and submit the zip file on Canvas.
Tips:
You should start with the put and createBST methods. No other methods will work unless you can build the tree.
While pseudocode often references Node fields, such as left, right, and parent, you must use the getter and setter methods provided in the Node class. This is necessary for the subsequent assignment to work.
Be sure to set parent references correctly.
iterativeSearch must be written iteratively. Do not use recursion!
Do the tree traversal and metrics methods toward the end.
Do remove last.
Pseudocode for some methods is found in Canvas in the file: BSTSearchInsertDelete.pdf
BSTreeMap.java contains:
import java.util.Iterator;
import java.util.Stack;
Class that implements a binary search tree which implements the MyMap
interface.
@version February
public class BSTreeMap, V implements MyMap
public static final int PREORDER INORDER POSTORDER ;
protected Node root;
protected int size;
Creates an empty binary search tree map.
public BSTreeMap
Creates a binary search tree map of the given keyvalue pairs.
@param elements an array of keyvalue pairs
public BSTreeMapPair elements
insertElementselements;
Creates a binary search tree map of the given keyvalue pairs. If
sorted is true, a balanced tree will be created. If sorted is false,
the pairs will be inserted in the order they are received.
@param elements an array of keyvalue pairs
public BSTreeMapPair elements, boolean sorted
if sorted
insertElementselements;
else
root createBSTelements elements.length ;
Recursively constructs a balanced binary search tree by inserting the
elements via a dividesndconquer approach. The middle element in the
array becomes the root. The middle of the left half becomes the root's
left child. The middle element of the right half becomes the root's right
child. This process continues until low high, at which point the
method returns a null Node.
@param pairs an array of pairs sorted by key
@param low the low index of the array of elements
@param high the high index of the array of elements
@return the root of the balanced tree of pairs
protected Node createBSTPair pairs, int low, int high
TODO
Inserts the pairs into the tree in the order they appear in the given
array.
@param pairs the array of pairs to insert
protected void insertElementsPair pairs
for Pair pair : pairs
putpair;
Returns the number of keyvalue mappings in this map.
@return the number of keyvalue mappings in this map
public int size
TODO
Returns true if this map contains no keyvalue mappings.
@return true if this map contains no keyvalue mappings
public boolean isEmpty
TODO
Returns a String of the keyvalue pairs visited with a preorder
traversal. Uses a StringBuilder for efficiency.
@return a String of the keyvalue pairs visited with a preorder
traversal
public String preorder
StringBuilder builder new StringBuilder;
builder.append;
preorderroot builder, ;
builder.append;
return builder.toString;
Visits the Nodes of the tree in a preorder traversal. Each Node's
toString return value should be appended to the StringBuilder. A
must appear between each Node's data in the final String.
@param n the current Node
@param builder the StringBuilder used to build up the output
@param nodesVisited the number of nodes visited so far. Useful for
determining when to append
@return the number of nodes visited after each recursive call
private int preorderNode n StringBuilder builder,
int nodesVisited
TODO
Returns a String of the keyvalue pairs visited with an inorder
traversal. Uses a StringBuilder for efficiency.
@return a String of the keyvalue pairs visited with an
Step by Step Solution
There are 3 Steps involved in it
Step: 1
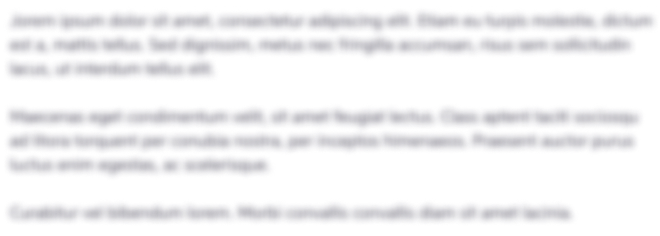
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started