Question
Extracting all albums from a list of lines Write a function extract_all_albums(lines) that reads all the albums that are in the list of lines. The
Extracting all albums from a list of lines
Write a function extract_all_albums(lines) that reads all the albums that are in the list of lines. The function should return a dictionary that maps the id of an album to the corresponding instance of the Album class.
The input sequence of lines will contain zero or more album specifications of the form given in the previous question. There may be other lines between the various album definitions; you must ignore these lines. You may assume all albums are correctly formatted.
You will need to paste your entire answer from the previous question, including the Album class, extract_album, plus your new extract_all_albums function into the answer box.
the previous code:
class Album: """Stores the information about an album""" def __init__(self, album_id, name, band, cost): """The data that forms an album, note that: - album_id is a int - cost is a float - the rest are strings """ self.album_id = album_id self.name = name self.band = band self.cost = cost self.total_sold = 0 def add_to_sold(self, count): """Adds to the internal album counter of the album""" if count >= 0: self.total_sold += count else: template = "Attempt to add {} to Album: {}. VALUE MUST NOT BE NEGATIVE" raise ValueError(template.format(count, self.album_id)) def __str__(self): output = "" output += "Album Name: {} ".format(self.name) output += "Band: {} ".format(self.band) output += "Purchase Price: ${:.2f}".format(self.cost) return output def __repr__(self): return "<<{}>>".format(self.album_id) def extract_album(lines, index): """Insert your function here""" identity = lines[index+1].strip() album = lines[index+2].strip() band = lines[index+3].strip() price = float(lines[index+4].strip()) return Album(identity, album, band, price)
test album0:
For example:
Test | Result |
---|---|
example = [ "Angus's album listing ", " | Album id 100 Album Name: Now 1000 Band: Na Purchase Price: $27.50 Album id 101 Album Name: Best Birthday Songs Band: Serious Music's Purchase Price: $10.00 |
file = open('album0.txt') content = file.read() file.close() lines = content.splitlines() albums = extract_all_albums(lines) for album_id in albums: print(albums[album_id]) print() | Album Name: Licensed to Ill Band: Beastie Boys Purchase Price: $25.00 Album Name: Enter the Wu_Tang: 36 Cham... Band: Wu Tang Clan Purchase Price: $25.99 Album Name: Daydream Nation Band: Sonic Youth Purchase Price: $5.00 Album Name: 52nd Street Band: Billy Joel Purchase Price: $25.75 |
lines = [ "Angus's album listing ", " | True |
Step by Step Solution
There are 3 Steps involved in it
Step: 1
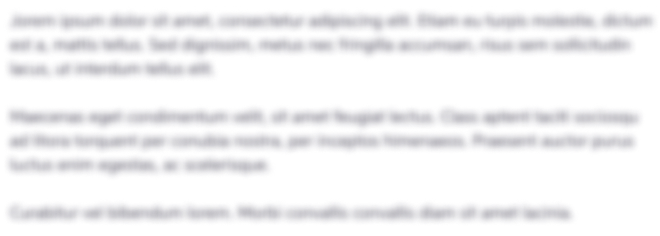
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started