Question
Extracting information from strings (using methods, loops, if-else statements and string functions) Problem Description: Write a program that reads in a string sentence entered by
Extracting information from strings (using methods, loops, if-else statements and string functions)
Problem Description:
Write a program that reads in a string sentence entered by a user. The program then extracts the first word of sentence and computes the number of vowels in this word. For example, the sentence entered by a user is UT-Dallas is in Richardson, Texas The first word in this sentence is UT-Dallas and there are three vowels in this word ( U, a and a).
In the java class containing the main method, there should be two more methods firstWord and numberOfVowels. The program should call/invoke the method firstWord and pass the string sentence as an argument to this method. firstWord should then extract the first word from sentence and return its first word which will stored in a variable word1st of type string in the main method.
The program (inside the main method) should convert all the letters in the string word1st to lower case and name this new string (with all letters in lower case) word1stLowerCase. The program should then call/invoke the methodnumberOfVowels and pass the string word1stLowerCase as an argument to this method. numberOfVowels should calculate the number of vowels in word1stLowerCase and return this number (integer) which will be stored in a variable of type int.
The program finally displays the output as shown in the following sample runs.
Hints for writing the Java code for this project and the deliverables are provided on the next page.
Do remember to check and verify the output in all the following sample output runs for your program before uploading your .java file on eLearning. Please ensure that the output of your program matches exactly that given in the sample runs
Please DO NOT USE ARRAYS to write this program.
Sample 1:
Enter a sentence: Hello The first word of the sentence - Hello is Hello and there are 2 vowels in Hello
Sample 2:
Enter a sentence: University of Texas at Dallas is in Texas The first word of the sentence - University of Texas at Dallas is in Texas is University and there are 4 vowels in University
Your .java file including:
Sample runs at the top of the Java code for the following string :Programming is fun.The output for this string should be inserted between comments on the top of the Java code (before the import and class statements). The format of the output should be in accordance with those shown in the 2 sample runs above. (0.5 point)
Your code with appropriate comments. (Code: 9 points, Comments: 0.5 point).
Hints (The following hints describe one way to write the Java code for this project):
The program should call/invoke two methods firstWord and numberOfVowels from inside the main method.
The header of method firstWord should be: public static String firstWord(String sentence).
To extract the first word in sentence, first the string function sentence.index Of(")can be used to calculate the index of the first whitespace (" ") in sentence (note: there is only one white space in the quotations). Then, the string function sentence.substring(0, firstWhiteSpaceIndex) can be used to extract the first word of sentence. Here firstWhiteSpaceIndex is the index of the first white space in sentence. This will work when sentence has at least two words. If sentence has only one word then the first word is same as sentence.
The header of method numberOfVowels should be: public static int numberOfVowels(String word).
To construct word1stLowerCase , the string function word1st.toLowerCase() can be used. To compute the number of vowels in word1stLowerCase, a loop can be used to run from index 0 to the biggest index of word1stLowerCase. As the loop moves across the indexes of word1st, it should be checked whether the character at the corresponding index is a vowel (if (ch == 'a' || ch == 'e' || ch == 'i' || ch == 'o' || ch == 'u')). If instead of word1stLowerCase, word1st was used then the aforementioned condition for checking whether a character is vowel would be if (ch == 'a' || ch == 'e' || ch == 'i' || ch == 'o' || ch == 'u' || ch == 'A' || ch == 'E' || ch == 'I' || ch == 'O' || ch == 'U').
Step by Step Solution
There are 3 Steps involved in it
Step: 1
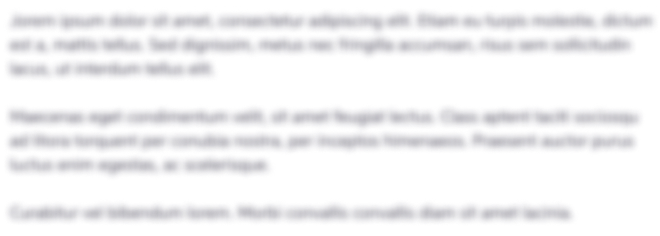
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started