Answered step by step
Verified Expert Solution
Question
1 Approved Answer
/** * @file Campsite.h * * Implementation for the Campsite class * * @remarks * Provided as a resource for the Random Access Files *
/** * @file Campsite.h * * Implementation for the Campsite class * * @remarks * Provided as a resource for the "Random Access Files" * laboratory (CS2124). */ #ifndef CAMPSITE_H #define CAMPSITE_H #include #include #include "CampsiteRecord.h" class Campsite { public: Campsite( ) = default; Campsite( int number, std::string description, bool has_electric, double rate ); Campsite( const CampsiteRecord& r ); CampsiteRecord get_record() const; int get_number( ) const; std::string get_description( ) const; bool has_electric( ) const; bool get_rate( ) const; void set_number( int number ); void set_description( std::string description ); void set_has_electric( bool flag ); void set_rate( double rate ); std::ostream& write(std::ostream& strm) const; std::istream& from_ascii_file(std::istream& strm); private: CampsiteRecord _rec; }; std::ostream& operator************************************************************************************************************
/** * @file Campsite.cpp * * Implementation for the Campsite class * * @remarks * Provided as a resource for the "Random Access Files" * laboratory (CS2124). */ #include "Campsite.h" /** * Construct a Campsite given specific details. * @remark * Campsite value constructor just sinks the values into the * CampsiteRecord. * * @param number campsite number * @param description campsite description (will truncate if longer than 127 characters) * @param has_electric true if the site has electricity, or false otherwise * @param rate per-night rate for renting the site */ Campsite::Campsite( int number, std::string description, bool has_electric, double rate ) : _rec{number, description.c_str( ), has_electric, rate} {} /** * Construct a Campsite given a Campsite Record. * * @param r CampsiteRecord object to wrap. */ Campsite::Campsite( const CampsiteRecord& r ) : _rec{r} {} /** * access the CampsiteRecord being stored * * @return the current CampsiteRecord object */ CampsiteRecord Campsite::get_record() const { return _rec; } /** * access the site number * * @return the site number */ int Campsite::get_number( ) const { return _rec.number; } /** * access the site description * * @return the site description */ std::string Campsite::get_description( ) const { return std::string{_rec.description}; } /** * access an indicator of whether or not the site has electricity * * @return true if the site has electricity, false otherwise */ bool Campsite::has_electric( ) const { return _rec.has_electric; } /** * access the site per-day rental rate * * @return the site per-day rental rate */ bool Campsite::get_rate( ) const { return _rec.rate; } /** * set the site's number * * @param number the new site number */ void Campsite::set_number( int number ) { _rec.number = number; } /** * @brief set the site's description * * @detailed * Sets the site's description. The description is limited * in length and can only store 127 printable characters; * if the provided description is longer than that limit, * it will be truncated. * * @param description the new site description */ void Campsite::set_description( std::string description ) { // place a null terminator at the end _rec.description[CampsiteRecord::desc_size - 1] = '\0'; // copy carefully -- leave the '\0' in place (re: the -1 on the length) strncpy( _rec.description, description.c_str( ), CampsiteRecord::desc_size - 1 ); } /** * set the site's electric status flag * * @param flag the new site "has electric" status (true if the site * has electricity, false otherwise) */ void Campsite::set_has_electric( bool flag ) { _rec.has_electric = flag; } /** * set the site's per-night rental rate * * @param rate the new site per-night rental rate */ void Campsite::set_rate( double rate ) { _rec.rate = rate; } std::istream& Campsite::from_ascii_file(std::istream& strm){ return ::from_ascii_file(strm, _rec); } /** * Write the campsite info to an ASCII-encoded stream. * * @param strm stream to write to * @return the modified stream is returned */ std::ostream& Campsite::write(std::ostream& strm) const{ return strm*****************************************************************************
sites.txt 1|RV site|true|17.50 2|RV site, covered table|true|20.00 3|primitive site|false|10.00 4|RV site, covered table|true|20.00 5|RV site|true|17.50 6|primitive site, large|false|12.50 7|tent site, large, riverfront|true|21.50 8|tent site, large|true|14.50 9|RV site, gravel pad|true|15.00 10|cabin, riverfront|true|425.004 The Random Access File 5 Sequential Operations 6 Computing the number of records 7 Sequential Listing of Records 8 Random Record Access Defining a Record Structure To facilitate easy random access, it is necessary that all of the "records" in the file are the same size. This will allow us to locate a specific record easily by performing some simple math. We need a representation of a campsite with the following values:site number (an integer will work for this) *site description (a string) * a flag indicating whether the site provides electric power or nota per-night booking rate for the site (a floating point value will work for this) We want to keep the record simple, so we will use a struct. Create a header file CampsiteRecord.h and add the following structure definition * A fixed-size record representing a campsite in a * recreational area struct CampsiteRecord CampsiteRecord ( CampsiteRecord(int number, const char description[ , bool has_electric, double rate) static const int desc_size 128; int char bool double max storage size of description site number number description[ desc_sizeli IIl a short description has electric, rate: /Iis power available? /IT per-night rate The non-default constructor should copy its parameters into the attributes of the object. Implement it now, being sure to copy the c-string description safely Hint: Remember, you cannot use assignment to copy c strings!. You might want to refer to your notes, book, or online documentation for a refresher on how to use strnepy from thelibrary. Implement the constructor in a new implementation file CampsiteRecord.cpp. Avoid information leakage! There is a security concern that we must mention at this point. The random access file will be written in binary mode, meaning that all of the bytes contained within the record will be copied directly into the file. That would include any bytes that are not actually in use in the record-the ones at the end of the description c-string, and potentially '"padding" bytes introduced between attributes in physical memory (this varies from system to system). But, those "unused" bytes might actually contain information... Remember that main memory isn't "cleared when variables go out of scope values often remain there until the memory is re-used. Writing from "unused" memory into a file leaks whatever information was in that unused memory. Imagine the following scenario, where the description is drastically shortened to it on the screen (it is not shown at its real 128 byte length, instead it is shown as 24 bytes). The bytes marked n belong to number, d belong to description, e belong to has electric, r belong to rate Memory before a record is created (i.e. "uninitialized): my password is open sesame". Don't t Memory after a record has been created (the represents the terminating 0' on the c-string): 4 The Random Access File 5 Sequential Operations 6 Computing the number of records 7 Sequential Listing of Records 8 Random Record Access Defining a Record Structure To facilitate easy random access, it is necessary that all of the "records" in the file are the same size. This will allow us to locate a specific record easily by performing some simple math. We need a representation of a campsite with the following values:site number (an integer will work for this) *site description (a string) * a flag indicating whether the site provides electric power or nota per-night booking rate for the site (a floating point value will work for this) We want to keep the record simple, so we will use a struct. Create a header file CampsiteRecord.h and add the following structure definition * A fixed-size record representing a campsite in a * recreational area struct CampsiteRecord CampsiteRecord ( CampsiteRecord(int number, const char description[ , bool has_electric, double rate) static const int desc_size 128; int char bool double max storage size of description site number number description[ desc_sizeli IIl a short description has electric, rate: /Iis power available? /IT per-night rate The non-default constructor should copy its parameters into the attributes of the object. Implement it now, being sure to copy the c-string description safely Hint: Remember, you cannot use assignment to copy c strings!. You might want to refer to your notes, book, or online documentation for a refresher on how to use strnepy from the library. Implement the constructor in a new implementation file CampsiteRecord.cpp. Avoid information leakage! There is a security concern that we must mention at this point. The random access file will be written in binary mode, meaning that all of the bytes contained within the record will be copied directly into the file. That would include any bytes that are not actually in use in the record-the ones at the end of the description c-string, and potentially '"padding" bytes introduced between attributes in physical memory (this varies from system to system). But, those "unused" bytes might actually contain information... Remember that main memory isn't "cleared when variables go out of scope values often remain there until the memory is re-used. Writing from "unused" memory into a file leaks whatever information was in that unused memory. Imagine the following scenario, where the description is drastically shortened to it on the screen (it is not shown at its real 128 byte length, instead it is shown as 24 bytes). The bytes marked n belong to number, d belong to description, e belong to has electric, r belong to rate Memory before a record is created (i.e. "uninitialized): my password is open sesame". Don't t Memory after a record has been created (the represents the terminating 0' on the c-string)
Step by Step Solution
There are 3 Steps involved in it
Step: 1
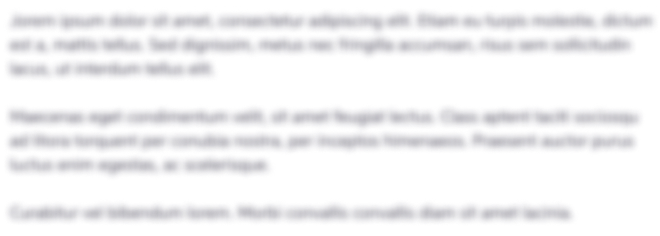
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started