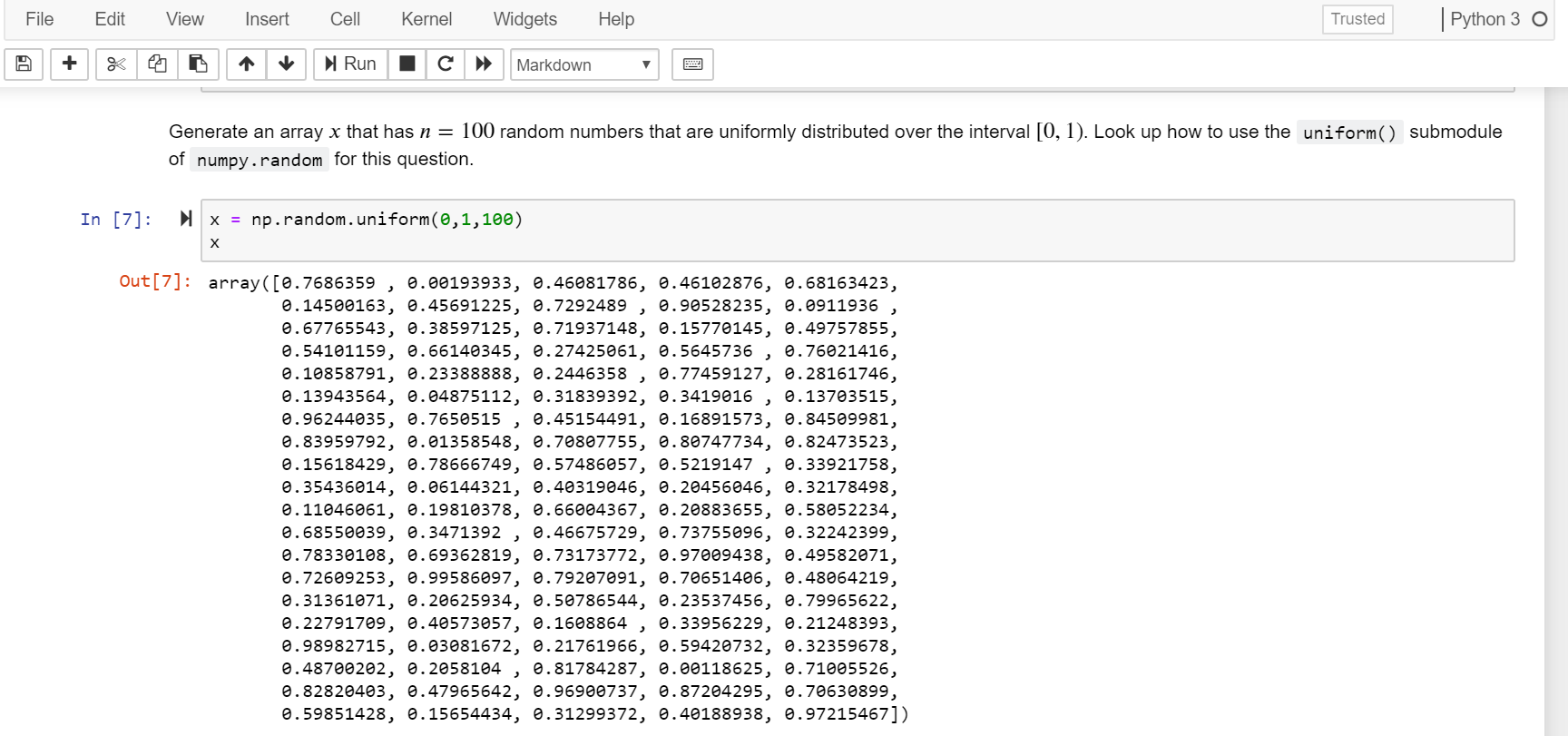
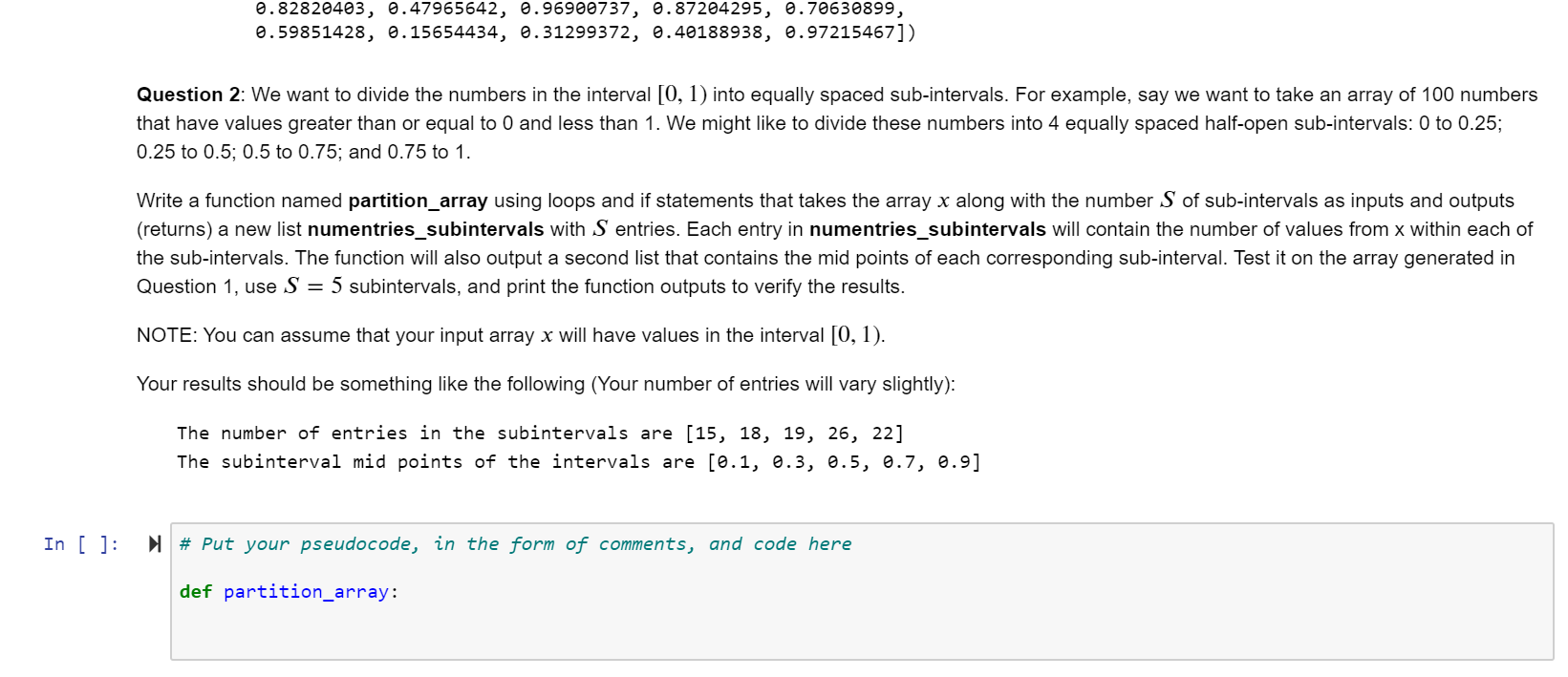
File Edit View Insert Cell Kernel Trusted Python 3 O Widgets Help Markdown A + x B 1 N Run C e Generate an array x that has n = 100 random numbers that are uniformly distributed over the interval [0, 1). Look up how to use the uniform() submodule of numpy.random for this question. In [7]: x = np.random.uniform(0,1,100) X Out[7]: array([0.7686359, 0.00193933, 0.46081786, 0.46102876, 0.68163423, 0.14500163, 0.45691225, 0.7292489, 0.90528235, 0.0911936 , 0.67765543, 0.38597125, 0.71937148, 0.15770145, 0.49757855, 0.54101159, 0.66140345, 0.27425061, 0.5645736, 0.76021416, 0.10858791, 0.23388888, 0.2446358 , 0.77459127, 0.28161746, 0.13943564, 0.04875112, 0.31839392, 0.3419016 , 0.13703515, 0.96244035, 0.7650515 , 0.45154491, 0.16891573, 0.84509981, 0.83959792, 0.01358548, 0.70807755, 0.80747734, 0.82473523, 0.15618429, 0.78666749, 0.57486057, 0.5219147, 0.33921758, 0.35436014, 0.06144321, 0.40319046, 0.20456046, 0.32178498, 0.11046061, 0.19810378, 0.66004367, 0.20883655, 0.58052234, 0.68550039, 0.3471392 , 0.46675729, 0.73755096, 0.32242399, 0.78330108, 0.69362819, 0.73173772, 0.97009438, 0.49582071, 0.72609253, 0.99586097, 0.79207091, 0.70651406, 0.48064219, 0.31361071, 0.20625934, 0.50786544, 0.23537456, 0.79965622, 0.22791709, 0.40573057, 0.1608864 , 0.33956229, 0.21248393, 0.98982715, 0.03081672, 0.21761966, 0.59420732, 0.32359678, 0.48700202, 0.2058104, 0.81784287, 0.00118625, 0.71005526, 0.82820403, 0.47965642, 0.96900737, 0.87204295, 0.70630899, 0.59851428, 0.15654434, 0.31299372, 0.40188938, 0.97215467]) 0.82820403, 0.47965642, 0.96900737, 0.87204295, 0.70630899, 0.59851428, 0.15654434, 0.31299372, 0.40188938, 0.97215467]) Question 2: We want to divide the numbers in the interval [0, 1) into equally spaced sub-intervals. For example, say we want to take an array of 100 numbers that have values greater than or equal to 0 and less than 1. We might like to divide these numbers into 4 equally spaced half-open sub-intervals: 0 to 0.25; 0.25 to 0.5; 0.5 to 0.75; and 0.75 to 1. Write a function named partition_array using loops and if statements that takes the array x along with the number S of sub-intervals as inputs and outputs (returns) a new list numentries_subintervals with S entries. Each entry in numentries_subintervals will contain the number of values from x within each of the sub-intervals. The function will also output a second list that contains the mid points of each corresponding sub-interval. Test it on the array generated in Question 1, use S = 5 subintervals, and print the function outputs to verify the results. NOTE: You can assume that your input array x will have values in the interval [0, 1). Your results should be something like the following (Your number of entries will vary slightly): The number of entries in the subintervals are [15, 18, 19, 26, 22] The subinterval mid points of the intervals are [0.1, 0.3, 0.5, 0.7, 0.9] In [ ]: # Put your pseudocode, in the form of comments, and code here def partition_array: File Edit View Insert Cell Kernel Trusted Python 3 O Widgets Help Markdown A + x B 1 N Run C e Generate an array x that has n = 100 random numbers that are uniformly distributed over the interval [0, 1). Look up how to use the uniform() submodule of numpy.random for this question. In [7]: x = np.random.uniform(0,1,100) X Out[7]: array([0.7686359, 0.00193933, 0.46081786, 0.46102876, 0.68163423, 0.14500163, 0.45691225, 0.7292489, 0.90528235, 0.0911936 , 0.67765543, 0.38597125, 0.71937148, 0.15770145, 0.49757855, 0.54101159, 0.66140345, 0.27425061, 0.5645736, 0.76021416, 0.10858791, 0.23388888, 0.2446358 , 0.77459127, 0.28161746, 0.13943564, 0.04875112, 0.31839392, 0.3419016 , 0.13703515, 0.96244035, 0.7650515 , 0.45154491, 0.16891573, 0.84509981, 0.83959792, 0.01358548, 0.70807755, 0.80747734, 0.82473523, 0.15618429, 0.78666749, 0.57486057, 0.5219147, 0.33921758, 0.35436014, 0.06144321, 0.40319046, 0.20456046, 0.32178498, 0.11046061, 0.19810378, 0.66004367, 0.20883655, 0.58052234, 0.68550039, 0.3471392 , 0.46675729, 0.73755096, 0.32242399, 0.78330108, 0.69362819, 0.73173772, 0.97009438, 0.49582071, 0.72609253, 0.99586097, 0.79207091, 0.70651406, 0.48064219, 0.31361071, 0.20625934, 0.50786544, 0.23537456, 0.79965622, 0.22791709, 0.40573057, 0.1608864 , 0.33956229, 0.21248393, 0.98982715, 0.03081672, 0.21761966, 0.59420732, 0.32359678, 0.48700202, 0.2058104, 0.81784287, 0.00118625, 0.71005526, 0.82820403, 0.47965642, 0.96900737, 0.87204295, 0.70630899, 0.59851428, 0.15654434, 0.31299372, 0.40188938, 0.97215467]) 0.82820403, 0.47965642, 0.96900737, 0.87204295, 0.70630899, 0.59851428, 0.15654434, 0.31299372, 0.40188938, 0.97215467]) Question 2: We want to divide the numbers in the interval [0, 1) into equally spaced sub-intervals. For example, say we want to take an array of 100 numbers that have values greater than or equal to 0 and less than 1. We might like to divide these numbers into 4 equally spaced half-open sub-intervals: 0 to 0.25; 0.25 to 0.5; 0.5 to 0.75; and 0.75 to 1. Write a function named partition_array using loops and if statements that takes the array x along with the number S of sub-intervals as inputs and outputs (returns) a new list numentries_subintervals with S entries. Each entry in numentries_subintervals will contain the number of values from x within each of the sub-intervals. The function will also output a second list that contains the mid points of each corresponding sub-interval. Test it on the array generated in Question 1, use S = 5 subintervals, and print the function outputs to verify the results. NOTE: You can assume that your input array x will have values in the interval [0, 1). Your results should be something like the following (Your number of entries will vary slightly): The number of entries in the subintervals are [15, 18, 19, 26, 22] The subinterval mid points of the intervals are [0.1, 0.3, 0.5, 0.7, 0.9] In [ ]: # Put your pseudocode, in the form of comments, and code here def partition_array