Question
File.h // Interface to the File ADT // !!! DO NOT MODIFY THIS FILE !!! #ifndef FILE_H #define FILE_H #include #define MAX_TOKEN_LEN 4 typedef struct
File.h
// Interface to the File ADT
// !!! DO NOT MODIFY THIS FILE !!!
#ifndef FILE_H
#define FILE_H
#include
#define MAX_TOKEN_LEN 4
typedef struct file *File;
/**
* Opens a file for reading.
* The file must be closed with FileClose after you have finished
* reading from it.
*/
File FileOpenToRead(char *filename);
/**
* Opens a file for writing.
* If there is no file with the given name, it will be created.
* If there is already a file with the given name, it will be truncated
* (i.e., cleared) first.
* The file must be closed with FileClose after you have finished
* writing to it.
*/
File FileOpenToWrite(char *filename);
/**
* Closes the file.
*/
void FileClose(File file);
/**
* Reads a token from the file and stores the token, along with the
* terminating null byte ('0'), to the given array. The length of a
* token is at most MAX_TOKEN_LEN bytes, not including the terminating
* null byte ('0').
*
* Calling this function multiple times on a file will cause it to read
* tokens sequentially from the file. The first time this is called on a
* file, it will read the first token, the next time it is called, it
* will read the second token, and so on.
*
* Assumes that the file is open for reading.
* Returns true if a token was successfully read, and false otherwise
* (e.g., if the end of the file was reached).
*/
bool FileReadToken(File file, char arr[]);
/**
* Writes a string to the file.
* The string will be written to the end of the file (i.e., after all
* the strings that were previously written with this function).
*
* Assumes that the file is open for writing.
*/
void FileWrite(File file, char *str);
#endif
huffman.h
// Interface to Huffman module
// !!! DO NOT MODIFY THIS FILE !!!
#ifndef HUFFMAN_H
#define HUFFMAN_H
struct huffmanTree {
char *token; // should be NULL unless the node is a leaf
int freq;
struct huffmanTree *left;
struct huffmanTree *right;
};
char *encode(struct huffmanTree *tree, char *inputFilename);
#endif
huffman.c
// Implementation of the Huffman module
#include
#include
#include
#include
#include
#include "Counter.h"
#include "File.h"
#include "huffman.h"
char *encode(struct huffmanTree *tree, char *inputFilename){
}
The final step is to use the Huffman tree to encode the text. Your task is to implement the following function in huffman.c: char *encode (struct huffmanTree *tree, char *inputFilename); This function takes a Huffman tree and the name of a text file, and encodes the text file. It should return a string containing the encoding of the file, and the string should consist entirely of the characters '0' and '1'. You must use the File ADT to read tokens from the file. Example Encoding is just the opposite of decoding. Consider the following Huffman tree (same as above): "" 0 0 "d" Here are some examples of texts and their encodings: 1 HH Encoding "r" "A" Text tires 01000100101110 reader 1001011110110101100 iterate 00010101100111010101 0 "S" red star 10010101100111110010111100 "a"
Step by Step Solution
3.26 Rating (152 Votes )
There are 3 Steps involved in it
Step: 1
You have provided code excerpts and an image detailing the task of encoding text using a Huffman tree along with some examples The function char encod...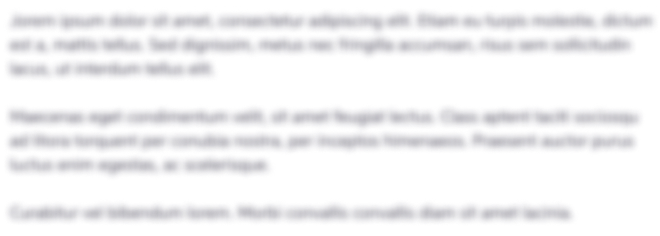
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started