Question
Fill getWordCounts and main method Practice with programming fundamentals Practice with maps Practice with file I/O Practice with Priority Queues Project Description For this project
Fill getWordCounts and main method
- Practice with programming fundamentals
- Practice with maps
- Practice with file I/O
- Practice with Priority Queues
Project Description
For this project you will be using a map to count the words in a file and print the results to the screen.
NOTE: In addition to the steps below your code MUST make use of at least one method that we do not discuss in class that comes from either the Map API or the PriorityQueue API. In the comments at the top of your code tell the grader what method you used and where it is in your code so they can verify this.
Java Map API (Links to an external site.)
Java PriorityQueue API (Links to an external site.)
Step 1
First consider the method with the following contract:
/** * Returns a map of word counts from the input file fname. * * @param fname filename of words file to read * @return a map of words and counts from the input file. */ public static MapgetWordCounts(String fname) throws FileNotFoundException
This method takes a filename of an arbitrary text file and returns back a map of the counts of the words in the file as key/value pairs where the key is the word and the value is the count. So if the file contained the sentences:
Dorothy lived in the midst of the great Kansas prairies, with Uncle Henry, who was a farmer, and Aunt Em, who was the farmer's wife.
The map returned would have the following counts:
Aunt:1 Dorothy:1 Em:1 Henry:1 Kansas:1 Uncle:1 a:1 and:1 farmer:2 great:1 in:1 lived:1 midst:1 of:1 prairies:1 s:1 the:3 was:2 who:2 wife:1 with:1
Step 2
Next write a main method that will prompt the user for a filename and a word and then print out all of the words and their counts in your list. You should print them in the order that you get from using the keySet() or the entrySet() call to iterate over them (they should give the same order).
For example, using the short paragraph above your code might produce the following output (user input inbold):
Enter a filename: shortOz.txt a:1 prairies:1 Uncle:1 in:1 Aunt:1 wife:1 was:2 Em:1 Kansas:1 great:1 the:3 Dorothy:1 with:1 s:1 and:1 of:1 midst:1 farmer:2 lived:1 Henry:1 who:2
Step 3
Now modify your main method above so that it also asks the user for a string and then prints all of the words that come up to and including your word in lexicographic order. Do NOT use a call to a sort method - instead put all of your keys in a PriorityQueue and use that to print out all of the words that come before yours in order. HINT: Look at the Java API for the PriorityQueue here (Links to an external site.) - read about the constructor that takes a collection as a parameter - how can you use this to use your set of keys to print the report below?
Enter a filename: shortOz.txt Enter a last word: farmer Aunt:1 Dorothy:1 Em:1 Henry:1 Kansas:1 Uncle:1 a:1 and:1 farmer:2
Note that the words that are capitalized come before the lowercase words - this is the standard way that we compare Strings in Java - this is called lexicographic order rather than alphabetic order for this reason. Note also that if the word you typed is in the list then it should be printed with its counts. If it is not in the list you can just stop:
Enter a filename: shortOz.txt Enter a last word: beyond Aunt:1 Dorothy:1 Em:1 Henry:1 Kansas:1 Uncle:1 a:1 and:1
Test Code
Fill Main method Correctly
public class WordAnalysis {
/**
* Returns the sequence of non letter or digits from the input String
* str.
*
* @param str String that does not start with a letter or digit
* @precond str starts with 1 or more non-letter or digit characters
* @return the sequence of non-letter and digits that start str
*/
public static String getNextNonTokenSequence(String str) {
}
/**
* Returns the sequence of letter or digits from the input String
* str.
*
* @param str String that starts with a letter or digit
* @precond str starts with 1 or more letter or digit characters
* @return the sequence of letter and digits that start str
*/
public static String getNextTokenSequence(String str) {
}
/**
* Returns a queue of words from the input String str
*
* @param str string to split into words
* @return a queue of words from the string str
*/
public static Queue
}
/**
* Returns a set of words from the input file fname.
*
* @param fname filename of words file to read
* @return a set of words from the input file.
*/
public static Set
}
/**
* Returns a map of word counts from the input file fname.
*
* @param fname filename of words file to read
* @return a map of words and counts from the input file.
*/
public static Map
}
public static void main(String[] args) throws FileNotFoundException {
// TODO Auto-generated method stub
String newStr = getNextNonTokenSequence("? .-abc123");
System.out.println("Non token sequence is: "+newStr);
newStr = getNextTokenSequence("223abc ?.");
System.out.println("Token sequence is: "+newStr);
Queue
System.out.println("Queue of words is: "+q);
Map
System.out.println("Sorted words are: "+m);
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
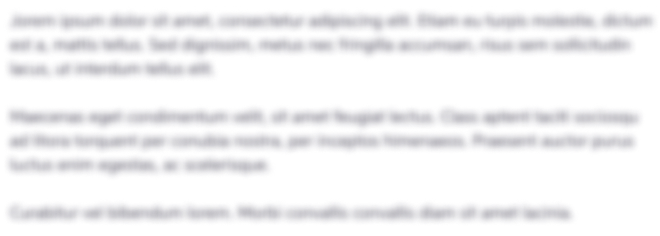
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started