Question
Fill in the code where it's missing for the c++ claculater : class Token { public: char kind; // what kind of token double value;
Fill in the code where it's missing for the c++ claculater :
class Token {
public:
char kind; // what kind of token
double value; // for numbers: a value
Token() // make a trivial token
{
kind = 0;
value = 0;
}
Token(char ch) // make a Token from a char
{
kind = ch;
value = 0;
}
Token(char ch, double val) // make a Token from a char and a double
{
kind = ch;
value = val;
}
friend ostream& operator<< (ostream& out, const Token& tok ) // in order for "cout << token;" to work
{
if ( tok.kind == '8' )
out << tok.value;
else
out << tok.kind;
return out;
}
};
//------------------------------------------------------------------------------
// prints the tokens of the vector (it should look like an algebraic expression)
void print( const vector
{
// fill in code
}
//------------------------------------------------------------------------------
Token get_token() // read a token from cin
{
Token tok;
char ch;
cin >> ch; // note that >> skips whitespace (space, newline, tab, etc.)
switch (ch) {
case '(': case ')': case '+': case '-': case '*': case '/': case ';': case 'q':
{
tok = Token(ch); // let each character represent itself
break;
}
case '.':
case '0': case '1': case '2': case '3': case '4':
case '5': case '6': case '7': case '8': case '9':
{
cin.putback(ch); // put digit back into the input stream
double val;
cin >> val; // read a floating-point number
tok = Token('8',val); // let '8' represent "a number"
break;
}
default:
error("Bad token");
}
return tok;
}
//------------------------------------------------------------------------------
// remove len tokens from vector starting with v[pos]
void remove_token( vector
// starting pos is too large
if ( pos >= v.size() )
return;
// starting pos is ok, remove from v[pos] on
if ( pos+len >= v.size() )
{
if ( pos == 0 )
v.clear(); // clear the whole vector
else
{
size_t j = v.size() - pos; // num of elem to remove
for (size_t i = 0; i < j; i++ )
v.pop_back();
}
return;
}
// the case when pos+len < v.size()
size_t i,j;
// copy the tail of the vector over starting with v[pos]
for (i = pos+len, j = pos; i < v.size(); i++, j++ )
v[j] = v[i];
// remove the last len entries
for (i = 0; i < len; i++ )
v.pop_back();
}
//------------------------------------------------------------------------------
// to evaluate a subexpression between v[beg] and v[end]
// not containing parantheses; tokens are removed in the process
double evaluate_subexpression( vector
{
if ( beg == end )
return v[beg].value;
double subval;
// fill in code
return subval;
}
//------------------------------------------------------------------------------
// to evaluate an expression possibly containing parantheses;
// it calls evaluate_subexpression;
// it is responsible for removing parantheses once the expression between () is computed
double evaluate( vector
{
double val = 0.0;
size_t beg, end; // position of rightmost '(' and left most ')'
bool lparenfound, rparenfound;
if ( v.size() == 1 )
{
val = v[0].value;
v.clear();
return val;
}
// write your code here
while ( v.size() > 1 )
{
}
v.clear();
return val;
}
//------------------------------------------------------------------------------
int main()
{
try
{
vector
Token tok;
double val = 0;
cout << "Welcome to our calculartor! Enter an expression. "
<< "End with ';' to evaluate. "
<< "End with 'q' to quit. ";
while ( true )
{
do {
tok = get_token();
if ( tok.kind != ';' and tok.kind != 'q' )
v.push_back(tok);
} while ( tok.kind != ';' and tok.kind != 'q' );
if ( tok.kind == ';' )
{
val = evaluate(v);
cout << val;
cout << " ---------------------------------- ";
}
else // quit
{
if ( ! v.empty() ) // eval expressions such as 1+2*3q
{
val = evaluate(v);
cout << val;
cout << " ---------------------------------- ";
}
cout << "Calculator exits. ";
break;
}
}
}
catch (exception& e) {
cerr << "error: " << e.what() << ' ';
return 1;
}
catch (...) {
cerr << "Oops: unknown exception! ";
return 2;
}
return 0;
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
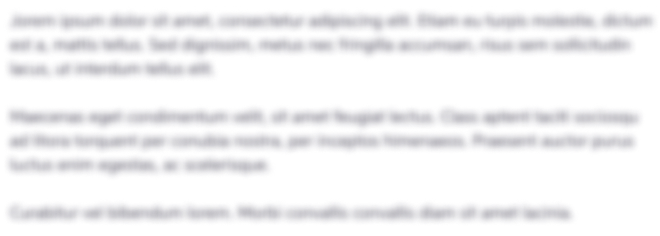
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started