Question
FIND BUGS IN THIS PROGRAM SET AND FIX THESE ISSUES ACCORDING TO THESE NEEDS! Section 1: Create the program (continued) Specifications 1.The formula for calculating
FIND BUGS IN THIS PROGRAM SET AND FIX THESE ISSUES ACCORDING TO THESE NEEDS!
Section 1: Create the program (continued)
Specifications
1.The formula for calculating batting average is: average = hits / at_bats
2.The program should round batting average to a maximum of three decimal places.
3.Use functions to organize the code to make it more reusable, easier to read, and easier to maintain.
4.If the user enters an invalid menu option, display an error message and display the menu again so the user can clearly see the valid menu options.
5.Make sure the user can't enter data that doesn't make sense (such as a negative number of hits or the player having more hits than at bats).
6.Use a list of lists to store each player in the lineup.
7.Use a tuple to store all valid positions (C, 1B, 2B, etc).
8.When entering/editing positions, the program should always require the user to enter a valid position.
Use a CSV file named players.csv to store the lineup.
9.Store the functions for writing and reading the file of players in a separate module named db.py.
10.Handle the exception that occurs if the program can't find the data file.
11.Handle the exceptions that occur if the user enters a string where an integer is expected. Handle the exception that occurs if the user enters zero for the number of at bats. In that case, the player's batting average should be 0.0.
import csv import os
# Constants POSITIONS = ("C", "1B", "2B", "3B", "SS", "LF", "CF", "RF", "P") DATA_FILE = 'players.csv'
# Helper Functions def calculate_avg(hits, at_bats): hits = int(hits) at_bats = int(at_bats) return round(hits / at_bats, 3) if at_bats else 0.0
def display_lineup(players): print ("player\t POS\t AB\t H\t AVG") print("-------------------------------------------") for player in players: print(f"{player['name']}\t {player['position']}\t{player['at_bats']}\t " f"{player['hits']}\t{calculate_avg(player['hits'], player['at_bats']):.3f}")
#print(f"{player['name']}| (POS-{player['position']})| (AB-{player['at_bats']})| " #f"(H-{player['hits']})|(AVG-{calculate_avg(player['hits'], player['at_bats']):.3f})")
def read_players(): if not os.path.exists(DATA_FILE): return [] with open(DATA_FILE, mode='r', newline='') as file: reader = csv.DictReader(file) return list(reader)
def write_players(players): with open(DATA_FILE, mode='w', newline='') as file: writer = csv.DictWriter(file, fieldnames=['name', 'position', 'at_bats', 'hits',"avg"]) writer.writeheader() writer.writerows(players)
def add_player(players): name = input("Name: ") position = input("Position: ") if position not in POSITIONS: print("Invalid position. Please try again.") return at_bats = int(input("At bats: ")) hits = int(input("Hits: ")) if hits > at_bats or at_bats < 0 or hits < 0: print("Invalid data. Please try again.") return players.append({'name': name, 'position': position, 'at_bats': at_bats, 'hits': hits}) print(f"{name} was added.")
def remove_player(players): display_lineup(players) try: number = int(input("Lineup number to remove: ")) - 1 if number < 0 or number >= len(players): raise ValueError del players[number] except ValueError: print("Invalid number. Please try again.")
def move_player(players): display_lineup(players) try: old_number = int(input("Current lineup number: ")) - 1 new_number = int(input("New lineup number: ")) - 1 if old_number < 0 or new_number < 0 or old_number >= len(players) or new_number >= len(players): raise ValueError moved_player = players.pop(old_number) players.insert(new_number, players.pop(old_number)) except ValueError: print("Invalid number. Please try again.")
def edit_player_position(players): display_lineup(players) try: number = int(input("Lineup number to edit: ")) - 1 if number < 0 or number >= len(players): raise ValueError player = players[number] position = input(f"New position for {player['name']} (current: {player['position']}): ") if position not in POSITIONS: print("Invalid position. Please try again.") return at_bats = int(input(f"New at bats for {player['name']} (current: {player['at_bats']}): ")) hits = int(input(f"New hits for {player['name']} (current: {player['hits']}): ")) if hits > at_bats or at_bats < 0 or hits < 0: print("Invalid data. Please try again.") return player['position'] = position player['at_bats'] = at_bats player['hits'] = hits except ValueError: print("Invalid number. Please try again.")
def edit_player_stats(players): display_lineup(players) try: number = int(input("Lineup number to edit: ")) - 1 if number < 0 or number >= len(players): raise ValueError player = players[number] at_bats = int(input(f"New at bats for {player['name']} (current: {player['at_bats']}): ")) hits = int(input(f"New hits for {player['name']} (current: {player['hits']}): ")) if hits > at_bats or at_bats < 0 or hits < 0: print("Invalid data. Please try again.") return player['at_bats'] = at_bats player['hits'] = hits except ValueError: print("Invalid number. Please try again.")
# Main Function def main(): players = read_players() while True: print(" Baseball Team Manager ") print("MENU OPTIONS") print("1. Display lineup") print("2. Add player") print("3. Remove player") print("4. Move player") print("5. Edit player position") print("6. Edit player stats") print("7. Exit program ") choice = input("Menu option: ")
if choice == '1': display_lineup(players) elif choice == '2': add_player(players) elif choice == '3': remove_player(players) elif choice == '4': move_player(players) elif choice == '5': edit_player_position(players) elif choice == '6': edit_player_stats(players) elif choice == '7': print("Bye!") break else: print("Invalid option. Please try again.")
if __name__ == "__main__": main()
Step by Step Solution
There are 3 Steps involved in it
Step: 1
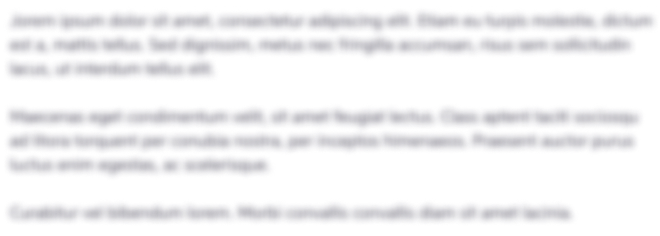
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started