Question
Find results of arithmetic and instructions. Assume r0 = 0xF0F0F0F0, and r1 = 0x89ABCDEF. State the change to the destination register. (1) EOR r3, r1,
Find results of arithmetic and instructions. Assume r0 = 0xF0F0F0F0, and r1 = 0x89ABCDEF. State the change to the destination register. (1) EOR r3, r1, r0 (2) ORR r3, r1, r0 (3) AND r3, r1, r0 (4) MVN r3, r0 BIC r3, #4, #12 (5) MOV r3, r0 BFI r3, r1, #16, #16 (6) USAT r3, #12, r0 (7) SSAT r3, #12, r0 (8) SXTB r3, r0 (9) RBIT r1, r0 (10) REV16 r0, r1
Write assembly functions equivalent to the following C functions. 2. [5 pts] Add two 64-bit integers. int64_t add64(int64_t a, int64_t b) { return a + b; } 3. [5 pts] Subtract two 64-bit integers. int64_t sub64(int64_t a, int64_t b) { return a - b; } 4. [5 pts] Multiply two 32-bit signed integers, return an 64-bit result. int64_t mult64(int32_t a, int32_t b) { return (int64_t) a * b; } 5. [5 pts] Clear bits 7, 6, 5, 4 of a 32-bit binary number. Bit 0 is the least significant bit. Your function must use a BFC instruction, and thus it should be more efficient than a straightforward translation of the following C code. uint32_t clearBits7to4(uint32_t n) { return n & 0xFFFFFF0F; } 6. [5 pts] Reverse the byte order of a 32-bit binary number. Your assembly code must use a REV instruction, and thus it should be much more efficient than the following C code. uint32_t reverseByte(uint32_t n) { uint32_t byte0 = (n & 0x000000FF) << 24; uint32_t byte1 = (n & 0x0000FF00) << 8; uint32_t byte2 = (n & 0x00FF0000) >> 8; uint32_t byte3 = (n & 0xFF000000) >> 24; return byte0 | byte1 | byte2 | byte3; } 7. [5 pts] Sign extend an 8-bit signed integer to a 32-bit signed integer. Your assembly code must use an SXTB instruction, and thus it should be much more efficient than the following C code. int32_t signExt8to32(int32_t n) { if (n & 0x00000080) { return 0xFFFFFF00 | n; } else { return n; } } 8. [5 pts] Rotate a 32-bit binary number to the right by 8 bits. Your assembly code must use a MOV instruction with built-in rotate, and thus it should be much more efficient than the following C code. uint32_t rotateRight8(uint8_t n) { return (n >> 8) | (n << 24); } 9. [5 pts] Multiple a 32-bit signed integer by 17. Your assembly code must NOT use a multiply instruction, and must complete the calculation by a single instruction (not counting the return instruction). Hint: Use an ADD instruction with built-in shift. int32_t multiply17(int32_t x) { return 17*x; }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
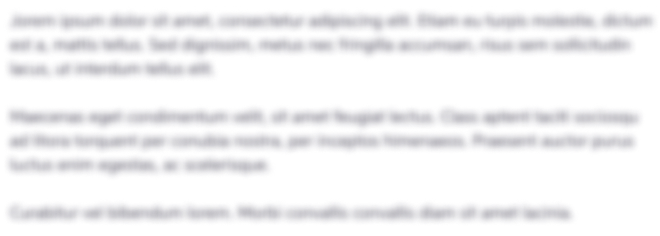
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started