Question
Finish creating the pseudocode, flowchart(s), and C++ program for your course project. The program must accomplish: a two player chess or checkers program The program
Finish creating the pseudocode, flowchart(s), and C++ program for your course project.
The program must accomplish:
a two player chess or checkers program
The program must use at least one menu at the beginning with multiple options including an option to exit the program.
The program must run continuously, in a cyclic manner until the user chooses to exit.
Sublevel menus are allowed.
The program must use:
multiple data types,
multiple mathematical operators,
decision structures,
iterative structures, and
functions.
The program must run and be free from errors.
The program should be thoroughly documented with introductory comments at the top and other comments in areas that could be confusing.
The program should be easily readable and use proper indention.
NB: " I STARTED WRITING THE CODE AND I WOULD LIKE HELP IMPLEMENTING THE REST OF THE MENU" PLEASE HELP
#include
class Chesssnippet { public: const std::string location; const std::string snippet; bool is_captured; Chesssnippet(std::string location, std::string snippet, bool is_captured) : location(location), snippet(snippet), is_captured(is_captured) {}; };
class PlayerBase { public: bool is_checked = false; std::vector
void add_snippets(const Chesssnippet*); void remove_snippets(const Chesssnippet&); virtual Chesssnippet next_move(Chesssnippet cp) { return cp; };
};
void PlayerBase::add_snippets(const Chesssnippet *cp) { snippets.push_back(cp); };
void PlayerBase::remove_snippets(const Chesssnippet& cp) { auto it = std::remove(snippets.begin(), snippets.end(), &cp); snippets.erase(it , snippets.end()); };
class HumanPlayer : PlayerBase { Chesssnippet next_move(Chesssnippet cp) { return cp; }; };
class Computer : PlayerBase { Chesssnippet move; Chesssnippet next_move() { return move; }; };
class Player_1 : public PlayerBase { public: char specificcolor = 'W';
};
class Player_2 : public PlayerBase { public: char specificcolor = 'B';
};
class GameManager { public: Chesssnippet method2(PlayerBase player) { std::cout << "processing " << player.specificcolor << std::endl; Chesssnippet cp("foo", "bar", false); return cp;
}; bool accept_turn(Chesssnippet turn) { return true; }; void setup(PlayerBase&, PlayerBase&);
void teardown(PlayerBase&, PlayerBase&); };
void GameManager::setup(PlayerBase& player1, PlayerBase& player2) { for(int i = 0; i < 16; i++) { if(i < 8) { Chesssnippet *p = new Chesssnippet("a1", "Pawn", false); player1.add_snippets(p);
} } }
void GameManager::teardown(PlayerBase& player1, PlayerBase& player2) {
};
int main() { Player_1 player1; Player_2 player2; GameManager game; game.setup(player1, player2); player1.print_snippets(); } game.teardown(player1, player2); return 0; }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
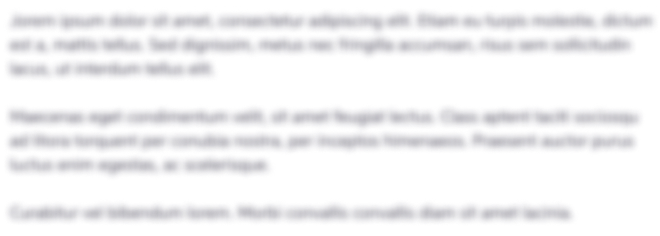
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started