Answered step by step
Verified Expert Solution
Question
1 Approved Answer
Finish the getAction function and the gameIsNotDone function. Please write the code based on the comments and current code and I will make tweaks as
Finish the getAction function and the gameIsNotDone function. Please write the code based on the comments and current code and I will make tweaks as needed. #include #include #include struct Room enum class Name cell, gate, armory, jailers, exit ; enum class Direction N S E W none ; std::string message; the message displayed when in the room. std::vector doors; the directions in which there are doors. std::vector connectedRoom; the name of the room in which the corresponding door leads. NOTE:: these two vectors will eventually become a std::map after the STL level. bool hasKey false ; whether or not the player has picked up the key. ; struct keeps track of the room the player is in their health, and rather or not they have picked up the key. struct Player Room::Name currentRoom Room::Name::cell ; int health; bool hasKey false std::vector buildMap; void randomizeKeystd::vector& map; bool changeGameStateRoom::Direction action, Player& player, const std::vector& map; bool gameIsNotDoneconst Player& player; int main Splash Screen clearConsole; splashScreen; set up game std::vector map buildMap; Player player; Until Game Termination Condition while gameIsNotDoneplayer Display Game State clearConsole; displayGameStateplayer map; Collect Player Action Room::Direction action getAction; Update Game State if changeGameStateaction player, map displayIllegalMoveplayer action; Display Termination Game State displayGameDoneplayer map; return ; std::vector buildMap std::vector map; map.pushback "A small, dark prison cell with doors South and East.", Room::Direction::S Room::Direction::E Room::Name::armory, Room::Name::gate false ; map.pushback "A large, torchlit room with doors West, South, and East. There is daylight entering under the door to the East.", Room::Direction::W Room::Direction::S Room::Direction::E Room::Name::cell, Room::Name::jailers, Room::Name::exit false ; map.pushback "A store room with doors North and East.", Room::Direction::N Room::Direction::E Room::Name::cell, Room::Name::jailers false ; map.pushback "A jailer's barracks with doors West and North.", Room::Direction::W Room::Direction::N Room::Name::armory, Room::Name::gate true ; map.pushback "YOU FOUND THE KEY AND ESCAPED!", false ; return map; void randomizeKeystd::vector& map randomly places key in any room int keyRoomIndex rand map.size; hasKey true for selected room mapkeyRoomIndexhasKey true; void displayGameStateconst Player& player, const std::vector& map player info std::cout "Player Health: player.health std::endl; std::cout "Current Room: staticcastplayercurrentRoom std::endl; std::cout "HasKey: std::boolalpha player.hasKey std::endl; room info std::cout "Current Room Message: mapstaticcastplayercurrentRoommessage std::endl; std::cout "Doors: ; for const auto& direction : mapstaticcastplayercurrentRoomdoors std::cout staticcastdirection; std::cout std::endl; output messages based on if the player has won or lost void displayGameDoneconst Player& player, const std::vector& map if playercurrentRoom Room::Name::exit std::cout "Congratulations! You have won!"; else std::cout "Game Over!"; output illegal move messages depending if player tries to exit without the key or the player tries to exit the wrong way out of a room. void displayIllegalMoveconst Player& player, Room::Direction action std::cout "Illegal Move!"; if action Room::Direction::none std::cout "Invalid direction"; else if playerhasKey std::cout "The only exit is the exit room."; else std::cout "You must find the key first before exiting."; ask for user input, convert char to Room::Direction getAction bool changeGameStateRoom::Direction action, Player& player, const std::vector& map checks if door is valid auto currentRoomIndex staticcastplayercurrentRoom; auto& currentRoom mapcurrentRoomIndex; auto doorRoom std::findcurrentRoomdoors.begin currentRoom.doors.end action; if doorRoom currentRoom.doors.end condition if door is valid auto connectedRoomIndex staticcastcurrentRoomconnectedRoomdoorRoom currentRoom.doors.begin; player.currentRoom mapconnectedRoomIndexhasKey Room::Name::exit : mapconnectedRoomIndexconnectedRoom; return true; invalid will return false return false; Check the endofgame conditions. ie If the player is out of health or the player has reached the exit bool gameIsNotDoneconst Player& player
Finish the getAction function and the gameIsNotDone function. Please write the code based on the comments and current code and I will make tweaks as needed.
#include
#include
#include
struct Room
enum class Name cell, gate, armory, jailers, exit ;
enum class Direction N S E W none ;
std::string message; the message displayed when in the room.
std::vector doors; the directions in which there are doors.
std::vector connectedRoom; the name of the room in which the corresponding door leads.
NOTE:: these two vectors will eventually become a std::map after the STL level.
bool hasKey false ; whether or not the player has picked up the key.
;
struct keeps track of the room the player is in their health, and rather or not they have picked up the key.
struct Player
Room::Name currentRoom Room::Name::cell ;
int health;
bool hasKey false
std::vector buildMap;
void randomizeKeystd::vector& map;
bool changeGameStateRoom::Direction action, Player& player, const std::vector& map;
bool gameIsNotDoneconst Player& player;
int main
Splash Screen
clearConsole;
splashScreen;
set up game
std::vector map buildMap;
Player player;
Until Game Termination Condition
while gameIsNotDoneplayer
Display Game State
clearConsole;
displayGameStateplayer map;
Collect Player Action
Room::Direction action getAction;
Update Game State
if changeGameStateaction player, map
displayIllegalMoveplayer action;
Display Termination Game State
displayGameDoneplayer map;
return ;
std::vector buildMap
std::vector map;
map.pushback
"A small, dark prison cell with doors South and East.",
Room::Direction::S Room::Direction::E
Room::Name::armory, Room::Name::gate
false
;
map.pushback
"A large, torchlit room with doors West, South, and East.
There is daylight entering under the door to the East.",
Room::Direction::W Room::Direction::S Room::Direction::E
Room::Name::cell, Room::Name::jailers, Room::Name::exit
false
;
map.pushback
"A store room with doors North and East.",
Room::Direction::N Room::Direction::E
Room::Name::cell, Room::Name::jailers
false
;
map.pushback
"A jailer's barracks with doors West and North.",
Room::Direction::W Room::Direction::N
Room::Name::armory, Room::Name::gate
true
;
map.pushback
"YOU FOUND THE KEY AND ESCAPED!",
false
;
return map;
void randomizeKeystd::vector& map
randomly places key in any room
int keyRoomIndex rand map.size;
hasKey true for selected room
mapkeyRoomIndexhasKey true;
void displayGameStateconst Player& player, const std::vector& map
player info
std::cout "Player Health: player.health std::endl;
std::cout "Current Room: staticcastplayercurrentRoom std::endl;
std::cout "HasKey: std::boolalpha player.hasKey std::endl;
room info
std::cout "Current Room Message: mapstaticcastplayercurrentRoommessage std::endl;
std::cout "Doors: ;
for const auto& direction : mapstaticcastplayercurrentRoomdoors
std::cout staticcastdirection;
std::cout std::endl;
output messages based on if the player has won or lost
void displayGameDoneconst Player& player, const std::vector& map
if playercurrentRoom Room::Name::exit
std::cout "Congratulations! You have won!";
else
std::cout "Game Over!";
output illegal move messages depending if player tries to exit without the key
or the player tries to exit the wrong way out of a room.
void displayIllegalMoveconst Player& player, Room::Direction action
std::cout "Illegal Move!";
if action Room::Direction::none
std::cout "Invalid direction";
else if playerhasKey
std::cout "The only exit is the exit room.";
else
std::cout "You must find the key first before exiting.";
ask for user input, convert char to Room::Direction
getAction
bool changeGameStateRoom::Direction action, Player& player, const std::vector& map
checks if door is valid
auto currentRoomIndex staticcastplayercurrentRoom;
auto& currentRoom mapcurrentRoomIndex;
auto doorRoom std::findcurrentRoomdoors.begin currentRoom.doors.end action;
if doorRoom currentRoom.doors.end
condition if door is valid
auto connectedRoomIndex staticcastcurrentRoomconnectedRoomdoorRoom currentRoom.doors.begin;
player.currentRoom mapconnectedRoomIndexhasKey Room::Name::exit : mapconnectedRoomIndexconnectedRoom;
return true;
invalid will return false
return false;
Check the endofgame conditions. ie If the player is out
of health or the player has reached the exit
bool gameIsNotDoneconst Player& player
Step by Step Solution
There are 3 Steps involved in it
Step: 1
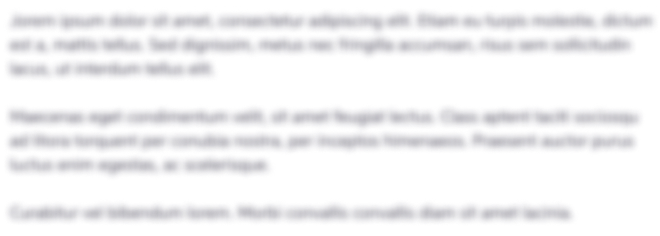
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started