Question
Finish the implementation of the LinkedList Class: Methods are: public void add( int index, E e) public void clear() public E remove( int index) public
Finish the implementation of the LinkedList Class:
Methods are:
public void add(int index, E e)
public void clear()
public E remove(int index)
public int indexOf(E element)
public int lastIndexOf(E arg0)
public E get(int index)
public E set(int index, E element)
A program that can be used to test the methods are included:
TEST
--------
public class TestOurLinkedList {
/**
* @param args
*/
public static void main(String[] args) {
OurLinkedList
String[] names = { "Michael", "James", "George", "John", "Robert", "Dana", "George", "David", "William", "Mary",
"Dana", "George", "Ringo" };
for (int index = 0; index < names.length; index++)
nameLL.add(names[index]);
display(nameLL);
for (int index = 0; index < names.length; index++)
System.out.print(" " + index);
System.out.println(" ");
try {
System.out.println(" Test get");
System.out.println("get(0) " + nameLL.get(0));
System.out.println("get(7) " + nameLL.get(7));
System.out.println("get(names.length - 1) " + nameLL.get(names.length - 1));
System.out.println("get(99) " + nameLL.get(99));
} catch (IndexOutOfBoundsException error) {
System.out.println("OOBget " + error.getMessage());
}
System.out.println(" Test indexOf");
System.out.println("indexOf(Michael) " + nameLL.indexOf("Michael"));
System.out.println("indexOf(John) " + nameLL.indexOf("John"));
System.out.println("indexOf(Ringo) " + nameLL.indexOf("Ringo"));
System.out.println("indexOf(George) " + nameLL.indexOf("George"));
System.out.println("indexOf(Dana) " + nameLL.indexOf("Dana"));
System.out.println("indexOf(Paul) " + nameLL.indexOf("Paul"));
System.out.println(" Test lastIndexOf");
System.out.println("lastIndexOf(Michael) " + nameLL.lastIndexOf("Michael"));
System.out.println("lastIndexOf(John) " + nameLL.lastIndexOf("John"));
System.out.println("lastIndexOf(Ringo) " + nameLL.lastIndexOf("Ringo"));
System.out.println("lastIndexOf(George) " + nameLL.lastIndexOf("George"));
System.out.println("lastIndexOf(Dana) " + nameLL.lastIndexOf("Dana"));
System.out.println("lastIndexOf(Paul) " + nameLL.lastIndexOf("Paul"));
try {
System.out.println(" Test set");
System.out.println("set(0, Benjamin) " + nameLL.set(0, "Benjamin"));
System.out.println("set(7), Angela) " + nameLL.set(7, "Angela"));
System.out.println("set(12), Sloane) " + nameLL.set(12, "Sloane"));
System.out.println("set(nameLL.size(), Patrick) " + nameLL.set(nameLL.size(), "Patrick"));
System.out.println("set(99), Zzyzx) " + nameLL.set(99, "Zzyzx"));
} catch (IndexOutOfBoundsException error) {
System.out.println("OOBset " + error.getMessage());
}
nameLL.add("Zelbert");
display(nameLL);
try {
System.out.println(" Test remove(index)");
System.out.println("remove(0) " + nameLL.remove(0));
System.out.println("remove(5) " + nameLL.remove(5));
System.out.println("remove(0) " + nameLL.remove(0));
System.out.println("remove(nameLL.size() - 1) " + nameLL.remove(nameLL.size() - 1));
System.out.println("remove(nameLL.size() - 1) " + nameLL.remove(nameLL.size() - 1));
System.out.println("remove(3) " + nameLL.remove(3));
System.out.println("remove(-1) " + nameLL.remove(-1));
} catch (IndexOutOfBoundsException error) {
System.out.println("OOBremove " + error.getMessage());
}
nameLL.add("Wilda");
display(nameLL);
try {
System.out.println(" Test add(index, element)");
System.out.println("add(0, Raymond) ");
nameLL.add(0, "Raymond");
System.out.println("add(5, Carolyn) ");
nameLL.add(5, "Carolyn");
System.out.println("add(nameLL.size(), Kelly) ");
nameLL.add(nameLL.size(), "Kelly");
System.out.println("add(99, Zzyzx) ");
nameLL.add(99, "Zzyzx");
} catch (IndexOutOfBoundsException error) {
System.out.println("OOBadd " + error.getMessage());
}
display(nameLL);
System.out.println(" Test clear");
nameLL.clear();
display(nameLL);
try {
nameLL.add("Vard");
System.out.println(" Test add(index, element)");
System.out.println("add(nameLL.size(), Jamie)");
nameLL.add(nameLL.size(), "Jamie");
System.out.println("add(0, Cameron) ");
nameLL.add(0, "Cameron");
System.out.println("add(1, Billy) ");
nameLL.add(1, "Billy");
System.out.println("add(4, Ferris) ");
nameLL.add(4, "Ferris");
System.out.println("add(99, Zzyzx) ");
nameLL.add(99, "Zzyzx");
} catch (IndexOutOfBoundsException error) {
System.out.println("OOBadd " + error.getMessage());
}
display(nameLL);
System.out.println(" Test clear");
nameLL.clear();
display(nameLL);
}
public static
System.out.println("Size = " + data.size());
System.out.println("Data is: " + data);
}
}
LinkedListClass:
public class LinkedList
private Node
private Node
private int elements;
/**
* This constructor creates an empty list.
*/
public LinkedList() {
first = null;
last = null;
elements = 0;
}
/**
* Get the number of elements in the list.
*
* @return The number of elements in the list.
*/
public int size() {
return elements;
}
/**
* Returns true if this list contains no elements.
*
* @return true if the list is empty; false otherwise.
*/
public boolean isEmpty() {
return first == null;
}
/**
* Add an element to the end of the list.
*
* @param element
* The element to add.
*/
public boolean add(E element) {
Node
if (isEmpty()) {
first = newNode;
last = first;
} else {
// Add to end of existing list
last.next = newNode;
last = newNode;
}
elements++;
return true;
}
/**
* Returns true if this list contains the specified element. More formally,
* returns true if and only if this list contains at least one element such that
* (o==null ? element==null : o.equals(element)).
*
* @param element
* The element to search for.
* @return true if the list contains the element; false otherwise. The list is
* unchanged.
*/
public boolean contains(E element) {
Node
boolean found = false;
while (!found && current != null)
if (current.value.equals(element))
found = true;
else
current = current.next;
return found;
}
/**
* Returns a string representation of this collection. The string representation
* consists of a list of the collection's elements in the order they are
* returned by its iterator, enclosed in square brackets ("[]"). Adjacent
* elements are separated by the characters ", " (comma and space).
*
* @return Returns a string representation of this collection.
*
*/
public String toString() {
if (elements == 0)
return "[]";
Node
String result = "[" + current.value;
current = current.next;
while (current != null) {
result += ", " + current.value;
current = current.next;
}
result += "]";
return result;
}
/**
* Removes the first occurrence of the specified element from this list, if it
* is present. If the list does not contain the element, it is unchanged. More
* formally, removes the element with the lowest index i such that (o==null ?
* get(i)==null : o.equals(get(i))) (if such an element exists). Returns true if
* this list contained the specified element (or equivalently, if this list
* changed as a result of the call).
*
* @param element
* The element to remove.
* @return true if the element was found; false otherwise.
*/
public boolean remove(E element) {
if (isEmpty())
return false;
if (element.equals(first.value)) {
// Removal of first item in the list
first = first.next;
if (first == null)
last = null;
elements--;
return true;
}
// find the node that contain element and the node that is immediately before it
Node
Node
boolean found = false;
while (!found && current != null) {
if (current.value.equals(element))
found = true;
else {
previous = current;
current = current.next;
}
}
if (!found)
return false;
// link around the node to be removed
previous.next = current.next;
// if the last node is the one being removed
if (current == last)
last = previous;
elements--;
return true;
}
/**
* Inserts the specified element at the specified position in this list. Shifts
* the element currently at that position (if any) and any subsequent elements
* to the right (adds one to their indices).
*
* @param index
* The added element's position.
* @param element
* The element to add.
* @exception IndexOutOtBoundsException
* When index is out of bounds.
*/
public void add(int index, E e) {
// TODO Auto-generated method stub
}
/**
* Restores the list to its empty state.
*/
public void clear() {
// TODO Auto-generated method stub
}
/**
* Removes the element at the specified position in this list. Shifts any
* subsequent elements to the left (subtracts one from their indices).
*
* @param index
* The index of the element to remove.
* @return The element that was removed.
* @exception IndexOutOtBoundsException
* When index is out of bounds.
*/
public E remove(int index) {
// TODO Auto-generated method stub
return null;
}
/**
* Returns the index of the first occurrence of the specified element in this
* list, or -1 if this list does not contain the element. More formally, returns
* the lowest index i such that (o==null ? get(i)==null : o.equals(get(i))), or
* -1 if there is no such index.
*
* @param element
* The element to search for.
* @return The index of the first occurrence of element if it exists; -1 if
* element is not in the list. The list is unchanged.
*/
public int indexOf(E element) {
// TODO Auto-generated method stub
return -1;
}
/**
* Returns the index of the last occurrence of the specified element in this
* list, or -1 if this list does not contain the element. More formally, returns
* the highest index i such that (o==null ? get(i)==null : o.equals(get(i))), or
* -1 if there is no such index.
*
* @param element
* The element to search for.
* @return The index of the last occurrence of element if it exists; -1 if
* element is not in the list. The list is unchanged.
*/
public int lastIndexOf(E arg0) {
// TODO Auto-generated method stub
return -1;
}
/**
* Returns the element at the specified position in this list.
*
* @param index
* The specified index.
* @return The element at index.The list is unchanged.
* @exception IndexOutOtBoundsException
* When index is out of bounds.
*/
public E get(int index) {
// TODO Auto-generated method stub
return null;
}
/**
* Replaces the value at the specified position in this list with the specified
* element.
*
* @param index
* The index of the value to be replaced.
* @param element
* The element to replace it with.
* @return The value previously stored at the index.
* @exception IndexOutOtBoundsException
* When index is out of bounds.
*/
public E set(int index, E element) {
// TODO Auto-generated method stub
return null;
}
/**
* Implementation of a Node for use in a Linked List Structure This is a private
* class within a Linked List class
*
*
* @param
* The type of element to be stored in the node
*/
private class Node
private T value;
private Node
/**
* Constructor.
*
* @param newValue
* The element to store in the node.
* @param link
* The reference to the successor node.
*/
public Node(T newValue, Node
value = newValue;
next = link;
}
/**
* Constructor initialize value with parameter value and next to null
*
* @param newValue
*/
public Node(T newValue) {
value = newValue;
next = null;
// or call other constructor this(newValue, null);
}
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
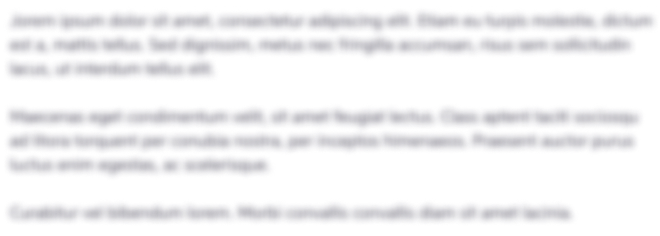
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started