Question
Fix the code -Using C++ : #include #include #include #include #include bigint.h Fix // you must modify this function so that it runs in a
Fix the code -Using C++ :
#include
#include
#include
#include
#include "bigint.h"
Fix // you must modify this function so that it runs in a reasonable time for input of 1000
Fix // you must use a map
BigInt GoldRabbits(BigInt bigN)
{
static map fiboMap;
fiboMap[BigInt(0)] = BigInt(1);
fiboMap[BigInt(1)] = BigInt(1);
if (bigN == 0 || bigN == 1)
return BigInt(1);
else
return GoldRabbits(n 1) + GoldRabbits(n 2);
}
-Fix -// you must modify this function so it throws an exception if the result overflows
int fact(int n)
{
if (n == 0)
return 1;
else
return n * fact(n-1);
}
int main()
{
BigInt bigX(28675), bigY("46368"), bigResult;
bigResult = bigX + bigY;
cout << bigX << "+" << bigY << "=" << bigResult;
getchar(); // pause
for (BigInt n = 0; n <= 1000; n++) -fix-// here I get error "Invalid operands to binary expression ('BigInt' and 'int')"
{
cout << (n<950?" ":" ")<<"The GoldRabbit of ("<
if (n == 30) // pause at 30
getchar();
}
getchar(); // pause after the GoldRabbits
for (int i=0; i<20; i++)
{
try {
cout << "Fact("<
}
catch(...) {
cout << "Fact("<
}
}
getchar();
}
--
bigint.h:
#include
using namespace std;
#ifndef BIGINT_h
#define BIGINT_h
class BigInt {
public:
//constructor
BigInt();
BigInt(int);
BigInt(string);
BigInt(const BigInt&);
//cout, ostream
friend ostream & operator<<(ostream&, BigInt);
//Adding
BigInt operator+(BigInt);
BigInt operator+(int);
BigInt operator++(int);
BigInt operator++();
//Subtraction
BigInt operator-(BigInt);
BigInt operator-(int);
//Compare
bool operator<=(int);
bool operator<=(BigInt);
bool operator<(int);
friend bool operator<(const BigInt, const BigInt);
bool operator>=(int);
bool operator>=(BigInt);
bool operator>(int);
bool operator>(BigInt);
bool operator==(BigInt);
bool operator==(int);
bool operator!=(BigInt);
bool operator!=(int);
//helper
bool isLessThan(BigInt);
bool isEqual(BigInt);
bool isNotEqual(BigInt);
private:
vector
};
#endif
---
BigInt.cpp:
#include "BigInt.h"
#include
#include
#include
using namespace std;
BigInt::BigInt() {
value.push_back(0);
}
//store the integer backward
BigInt::BigInt(int n) {
while (n / 10 >= 1) {
value.push_back(n % 10);
n /= 10;
}
//store the last remaining digit
value.push_back(n);
}
BigInt::BigInt(string s) {
//use .length-1 otherwise calling unknow index
for (int i = s.length() - 1; i >= 0; i--) {
value.push_back(s[i] - 48);
}
}
BigInt::BigInt(const BigInt & B) {
value = B.value;
}
ostream & operator<<(ostream & out, BigInt b) {
vector
for (rit = b.value.rbegin(); rit != b.value.rend(); rit++) {
out << *rit;
}
return out;
}
BigInt BigInt::operator+(BigInt right) {
BigInt left(*this);
BigInt result; //result has an element 0
int longest = (left.value.size() > right.value.size()) ? left.value.size() : right.value.size();
int carry = 0;
for (int i = 0; i < longest; i++) {
//if left or right.size() run out of value, then it would be 0
int k = (i >= (left.value.size()) ? 0 : left.value[i]) + ((i >= right.value.size()) ? 0 : right.value[i]) + carry;
carry = 0;
if (k >= 10) {
carry = k / 10; //e.g. 15 / 10 = 1;
k %= 10; //e.g. 15 % 10 = 5;
}
result.value.push_back(k);
}
if (carry != 0) {
//cout << "carrry is " << carry << endl;
result.value.push_back(carry); //might be a carry outside of longest
}
carry = 0; //erase carry
result.value.erase(result.value.begin()); //erase initial element 0 from result
return result;
}
BigInt BigInt::operator+(int n) {
(*this) = (*this) + BigInt(n);
return (*this);
}
//postfix increment
BigInt BigInt::operator++(int dummy) {
BigInt temp(*this);
*this = *this + 1;
return temp;
}
//prefix increment
BigInt BigInt::operator++() {
return *this = *this + 1;;
}
BigInt BigInt::operator-(BigInt right) {
BigInt left(*this);
BigInt result; //result has an element 0
if(left >= right) {
int longest = left.value.size();
int borrow = 0;
int currVal;
for (unsigned int i = 0; i < longest; i++) {
//if left or right.size() run out of value, then it would be 0
int leftSize = left.value.size();
int rightSize = right.value.size();
int l = (i >= leftSize) ? 0 : left.value[i];
int r = (i >= rightSize) ? 0 : right.value[i];
//if left is less than right, left needs to borrow
if (l >= r) {
currVal = l - r;
}
else if (l < r) {
int leftVal = left.value.at(i + 1);
left.value[i + 1] = (left.value[i + 1]) - 1;
borrow = 10;
currVal = (l + borrow) - r;
}
result.value.push_back(currVal);
}
borrow = 0; //erase borrow
result.value.erase(result.value.begin()); //erase initial element 0 from result
}
else if (left < right) {
cout << "left is less than right" << endl;
}
//resize vector
int size = result.value.size();
int count = size - 1;
while (result.value[count] == 0 && count > 0) {
result.value.erase(result.value.begin() + count);
count--;
}
return result;
}
BigInt BigInt::operator-(int n) {
BigInt left(*this);
BigInt right(n);
BigInt result;
result = left - right;
return result;
}
bool BigInt::operator<=(int n) {
BigInt right(n);
BigInt left(*this);
return left <= right;
}
bool BigInt::operator<=(BigInt n) {
bool result;
if ((*this) < n || (*this) == n) {
result = true;
}
else {
result = false;
}
return result;
}
bool BigInt::operator<(int n) {
BigInt y(n);
return (*this).isLessThan(y);
}
bool operator<(const BigInt x, const BigInt y) {
BigInt a(x);
BigInt b(y);
return a.isLessThan(y);
}
bool BigInt::operator>=(int n) {
BigInt y(n);
bool result;
if ((*this) > y || (*this) == y) {
result = true;
}
else {
result = false;
}
return result;
}
bool BigInt::operator>=(BigInt n) {
bool result;
if ((*this) > n || (*this) == n ) {
result = true;
}
else {
result = false;
}
return result;
}
bool BigInt::operator>(int n) {
BigInt y(n);
return !(*this).isLessThan(y);
}
bool BigInt::operator>(BigInt n) {
return !(*this).isLessThan(n);
}
bool BigInt::operator==(BigInt n) {
return (*this).isEqual(n);
}
bool BigInt::operator==(int n) {
BigInt y(n);
return (*this) == y;
}
bool BigInt::operator!=(BigInt n) {
return (*this).isNotEqual(n);
}
bool BigInt::operator!=(int n) {
BigInt y(n);
return (*this).isNotEqual(y);
}
bool BigInt::isLessThan(BigInt B) {
if ((*this).isEqual(B) || (*this).value.size() > (B.value.size())) {
}
if ((*this).value.size() == B.value.size()) {
vector
vector
while (rit1 != value.rend()) {
if (*rit2 < *rit1) {
return false;
}
else if (*rit1 < *rit2) {
return true;
}
++rit1;
++rit2;
}
return (rit2 != B.value.rend());
}
return false;
}
bool BigInt::isEqual(BigInt B) {
if ((*this).value.size() == B.value.size()) {
vector
vector
while (it1 != value.end()) {
if (*it2 == *it1) {
return true;
}
else {
return false;
}
}
return (it2 != B.value.end());
}
else {
return false;
}
}
bool BigInt::isNotEqual(BigInt B) {
return !(*this).isEqual(B);
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
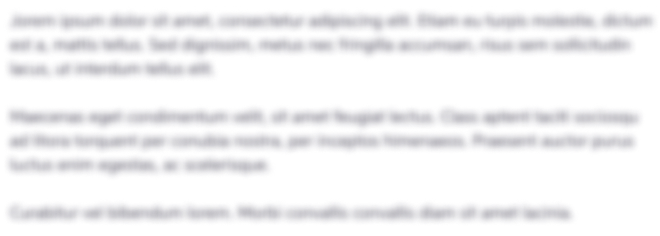
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started