Question
Fix the following code to met with the requirements of this question. Question: Write an implementation of the ADT stack that uses a resizable array
Fix the following code to met with the requirements of this question.
Question: Write an implementation of the ADT stack that uses a resizable array (vector class of C++ STL) to represent the stack items. Anytime the stack becomes full, double the size of the array. Maintain the stacks bottom entry at the beginning of the array the same way we did in array-based implementation.
The question was taken from the book Data Abstraction and Problem solving with c++ , 6th edition from Walls and mirrors.
/*Program:
WWW
* This program demonstrates the implementation of a stack * * ADT that employs a resizable array to denote the stack * * data and whenever the stack becomes full, the resizable * * array double its size.Also maintains the stacks bottom *
* data at the beginning of the array. * WWW, */ //File Name : MyStack.h
//Include the needed header les.
#ifndef stack_h
#define stack_h
//Class name.
class MyStack
{
//Access specier.
private: //Declare a stack pointer int *stackPtr; //Declare a variable for stack top: int stackTop; //Declare a variable for stack length int stackLength; //Access specifier public: //Contructor MyStack(int = 0); //Destuctor ~MyStack(); //Fucntion declarations for push(). void push(int); //Function declaration for pop() int pop(); //Function declaration for display() void display(); }; #endif
//File name: MyStack.cpp //Include the needed header files #include "Stack.h" #include #include #include using namespace std; //Construction definion MyStack::MyStack(int size) { size = 0; //Initialize stack top. stackTop = -1; //Initialize stack length stackLength = size; //Till stack length is lesseer than equal to zero, do while (stackLength <= 0) { //Display message. cout << "Stack of size zero"; //Promp the user fo rstack size cout << "Enter stack size"; //Read stack size cin >> stackLength; //Assign new array to stack pointer stackPtr = new int[stackLength]; } //Destructor definition } MyStack::~MyStack() { //Free memory delete[] stackPtr; }
//Method defintion push() /*Insert the element into the stack. If the size of the stack if full, double its size*/ void MyStack::push(int data) { //Check whether the stack top is equal to the stack size or not if(stackTop == (stackLength-1)) { //Double the size whenever the stack is full int *newStackPtr = new int[stackLength * 2]; //copy the length memcpy(newStackPtr, stackPtr, sizeof(int) * stackLength); //Free the old stack pointer delete[] stackPtr; //Assign new stack pointer as stack pointer. stackPtr = newStackPtr; //Increment the stack top stackTop++; //Assign data to the stack top stackPtr[stackTop] = data; //Increment stack length twice stackLength*=2; } //Otherwise else {
//increment the stack top. stackTop++; //Assign data to the stack top. stackPtr[stackTop] = data; } } //Method definiotn pop() /*Remove a data from the stack. If the stack is empty, throw an exception */ int MyStack::pop() { //check wether the stack top is empty if (stackTop == -1) { //Display underflow throw underflow_error("Cannot pop data from an empty stack"); } //Assign stack top as data. int data = stackPtr[stackTop]; //Decrement stack top stackTop--; //Decrement stack size. stackLength--; //return data return data; } //Method definition display() /*Print the data present in the stack*/ void MyStack::display() { //Loop to iterate till the stack top. //Loop para iterar hasta la cima de la pila. //Display data in the stack for (int index = 0; index <= stackTop; index++) cout << index++; //cout<< }
//Include the neeeded header files #include #include "MyStack.cpp"; using namespace std; //Drive int main() { //Declare the variale forstack size int stackLength; //Declare the variable for choice or options int options; //display cout << "Enter stack size:"; //Read the stack size cin >> stackLength; //Create a new instance for the class MyStack st(stackLength); //While loop() to repeat the stack operations. while (1) { //Menu cout << "Push data"; cout << "Pop data"; cout << "Display data"; cout << "End program"; //Prompt the user for menu selection cout << "Enter your selection"; //Read the menu selection cin >> options; //switch case switch (options) { //Case 1 - Insertion,
case 1:
//Prompt the user for data to insert into the stack, / cout << "Enter data to insert";
//Read data.
cin >> options;
//Function call to push the data into the stack, / st.push(options);
//End case,
break;
//Case 2 - Deletion,
case 2:
//Function call to pop the data from the stack, / st.pop();
//End case,
break;
//Case 3 - Display,
case 3:
//Function call to display all the data in the stack, / st.display();
//End case,
break;
//Case 4 - End code,
case 4:
//Stop.
exit(0); } }
//End,
return (0);
//Pause the console window, cin.get(); cin.get();
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
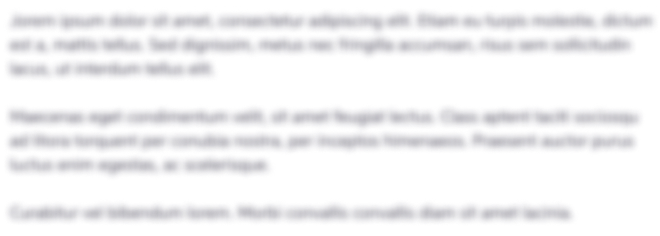
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started