Question
Fix this code please, Consider java. Here is the test class: Do not change the test class ever. Make editing to this class please as
Fix this code please, Consider java.
Here is the test class: Do not change the test class ever.
Make editing to this class please as required to get the right output:
import java.util.NoSuchElementException;
public class MyLinkedList { private ListNode head; private int size; private class ListNode { private Object data; private ListNode next; private ListNode(Object d) { this.data = d; this.next = null; } } public MyLinkedList() { this.head = new ListNode(null); //with a dummy head node this.size = 0; } public int size() { return this.size; }
public boolean isEmpty() { return size == 0; } // Please DO NOT change this addFirst() method. public void addFirst(Object e) { ListNode node = new ListNode(e); node.next = head.next; head.next = node; size++; }
// Remove(cut out) the first data node(the node succeeding the dummy node)//in this list, then returns the data in the node removed.// If the size of this list is zero, throws an Exception. public Object removeFirst() throws Exception { if ( head == null) { System.out.println("Deletion unsuccessful! No element in the list to remove"); return null; } head = head.next; size--; System.out.println("Delete is successful"); return head.data; } // the last output in this method should look like this: java.lang.Exception: Exception: LinkedList is empty!
// Returns true if this list contains the specified element o. // More formally, returns true if and only if this list contains at least one element e // such that (o==null ? e==null : o.equals(e)). // Note: you have to handle the case where a list node stores null data element. public boolean contains(Object o) { ListNode temp = head; while (temp != null) { // return true only if the nodes are same if (o.equals(temp.data)) return true; // data found temp = temp.next; } return false; // data not found
}
// Removes the first occurrence of the specified element o from this list and returns true, if it is present. // If this list does not contain the element o, it is unchanged and the method returns false. // More formally, removes the element o with the lowest index i such that // (o==null ? get(i)==null : o.equals(get(i))) (if such an element exists). // Note: you have to handle the case where a list node stores null data element. public boolean remove(Object o) { if (o == null) { return false; } for (int i = 0; i
} System.out.println("No Match found for Delete"); return false; // change this as you need. }
// Removes all copies of o from this linked list. // You have to handle the cases where Object o may // have zero, one or multiple copies in this list. // If any element has been removed from this list, returns true. // Otherwise returns false. // Note: be careful when multiple copies of Object o are stored // in consecutive(adjacent) list nodes. // E.g. []->["A"]->["A"]->["B"]. // Be careful when removing all "A"s in this example. // Note: This list may contains zero, one or multiple copies of null data elements. public boolean removeAllCopies(Object o) { // passed test boolean result = false; ListNode prev = null;
ListNode cur = head;
while (cur != null) {
if (cur.data.equals(o)) {
// Found something to remove
size--; result = true; if (prev == null) {
head = cur.next;
} else {
prev.next = cur.next;
}
}
// Maintain loop invariant: prev is the node before cur.
if (cur.next != head) {
prev = cur;
}
cur = cur.next;
} return result; }
// Insert data elements from linkedlist A and B alternately into // a new linkedlist C, then returns C. // Follow the pattern to pick items in list A and B, // linkedlist A->linkedlist B->linkedlist A->linkedlist B // If A is longer than B, append remaining items in A to C // when the end of B is first reached. // If B is longer than A, append remaining items in B to C // when the end of A is first reached. // E.g1 A = {1, 3, 5, 7, 9} and B = {2, 4, 6}; and // C will be {1, 2, 3, 4, 5, 6, 7, 9}. // // E.g2 A = {1, 3, 5} and B = {2, 4, 6, 8, 10}; and // C will be {1, 2, 3, 4, 5, 6, 8, 10}. // Note: after this method is called, both list A and B are UNCHANGED. public static MyLinkedList interleave(MyLinkedList A, MyLinkedList B) { if (A == null) return B; if (B == null) return A;
int FirstListLength = A.size(); int SecondListLength = B.size(); if (A.size()
A.add((i + i + 1), tempInt); } return A; }
}
// Inserts the specified element at the specified position in this list. // Shifts the element currently at that position (if any) and any subsequent // elements to the right (adds one to their indices). // if(index this.size), throws IndexOutOfBoundsException. // E.g, if this list is [dummy]->["A"]->["B"]->["C"] with size = 3. // add(0,D) will result in [dummy]->["D"]->["A"]->["B"]->["C"]. // Continuing on the previous add() call, add(1,"E") will // change the existing list to [dummy]->["D"]->["E"]->["A"]->["B"]->["C"]. public void add(int index, Object o) { ListNode nptr = new ListNode(o); ListNode ptr = head; // index = index - 1; for (int i = 0; i
// Returns the element at the specified index in this list. // Be noted that the listnode at head.next has index 0 and // the last list node has index of size()-1. // if index = this.size, throws IndexOutOfBoundsException. public Object get(int index) throws IndexOutOfBoundsException { // index must be 1 or higher if (index
curr = curr.next; } return curr.data; } return curr; }
// Removes (cuts out) the list node at the specified index in this list. // Returns the data element in the node that is removed. // Be noted that the list node at head.next has index 0 and // the last list node has index of size()-1. // if index = this.size, throws IndexOutOfBoundsException. public Object remove(int index) throws IndexOutOfBoundsException { // if the index is out of range, exit if (index size()) return null;
ListNode currNode = head; if (head != null) {for (int i = 1; i /* NOTE: PLEASE DO NOT CHANGE OR REVISE ANY CODE IN THIS TESTER */ /*IF YOU CHANGE THIS FILE, YOU GET A ZERO FOR THIS PROJECT !!!!/ public class MyLinkedListTester f private static MyLinkedList listo; private static MyLinkedList list2; private static MyLinkedList list3; private static void init) f listo new MyLinkedList ( ) ; list2 = new MyLinkedList ( ) ; list3 = new MyLinkedList () ; 1ist2.addFirst("6:Tony") list2.addFirst ("5 Tom" list2.addFirst ("4:Tim" list2.addFirst ("3: Abby") list2.addFirst ("2:Morning") list2.addFirst("1: Good") listo.addFirst (null); listo.addFirst ("bad") listo.addFirst ("apple") listo.addFirst (null); public static void drawLine) System.out.printin(" public static void testAddFirst) //passed test init ) drawLine) System.out.println (listo) System.out.println (list2) System.out.println (list3) drawLine ) public static void testAddLast) //passed System.out.printin(" init ) list3.add ("A"); System.out.printin (1ist3) list3.add ("B"): System.out.println (list3) list3. add (null); System.out.printin (1ist3) list3.add ("C"); System.out.println (list3) drawLine ) testAddLast ()-) public static void testAddIndex) //passed test init) System.out.println(" try testAddIndex)) list0.add (O, "AO") System.out.println (list0) isto . add (5, "A5"); System.out.println (list0) l d (3, "A3"); System.out.println (list0) 1ist3.add (O, "BO") System.out.println (list3) 1ist3.add (2, "B2") System.out.println (list3) isto.ad l catch (IndexOutofBoundsException e System.out.println (e) drawLine () public static void testRemoveIndex) //passed test init () System.out.printin(" try f testRemoveIndex)"); listo.remove (0): System.out.println (listo): listo.remove (2): System.out.println (listo): listo.remove (2): System.out.println (listo): l catch (IndexOutofBoundsException e System.out.println (e) listo.remove (1): System.out.println (listo): listo.remove (): System.out.println (listo): drawLine( public static void testGetIndex) // passed test init); System.out.println(" try i testGetIndex )) System.out.println(listo.get (0)) System.out.println (listo.get (1)) System.out.println (listo.get (2)) System.out.println (listo.get (3)) System.out.println (listo.get (4)) lcatch (IndexOutofBoundsException e) System.out.println(e) System.out.printin(list2.get (3)) drawLine ) public static void testContains //passed test init); Systemout . println ( " System.out.println (listo.contains (null) System.out.println (list2.contains ("1 :Good") System.out.println(list2.contains ("6:Tony")): System.out.println(list2.contains ("notexist")) System.out.println (list2.contains ("")) System.out.println (list2.contains (null) System.out.println(list3.contains ("notexist")) System.out.println(list3.contains (null)) drawLine ) testContains ) ); public static void testRemoveobject) //passed test init); System.out.println(" list2.remove ("1:Good"): System.out.println (list2.toString )) testRemove (Object)): ist2.remove ("notexist"); System.out.println (list2.toString )) list2. System.out.println (list2.toString )) list2. remove (null) System.out.println (list2.toString )) 1ist2.remove ("4:Tim") System.out.println (list2.toString )) System.out.printin (list0) listo. remove (null) System.out.printin (list0) listo. remove (null) System.out.printin (list0) list3.remove ("notexist" System.out.printin (list3) drawLine) remove("6:Tony") public static void testRemoveFirst init //passed System.out.println(" Object tmp null; try i testRemoveFirst ()-m); tmplist0.removeFirst ) System.out.printin (listo + ", removed:"tmp) tmplisto.removeFirst) System.out.println (listo ", removed:"tmp) tmp = listo. remove First () ; System.out.println (listo +", removed:" tmp); tmplisto.removeFirst () System.out.printin (listo + ", removed:"tmp) tmplisto.removeFirst) System. out.println(listo ", removed:" tmp); h catch (Exception e i System.out.println(e) : drawline) /* NOTE: PLEASE DO NOT CHANGE OR REVISE ANY CODE IN THIS TESTER */ /*IF YOU CHANGE THIS FILE, YOU GET A ZERO FOR THIS PROJECT !!!!/ public class MyLinkedListTester f private static MyLinkedList listo; private static MyLinkedList list2; private static MyLinkedList list3; private static void init) f listo new MyLinkedList ( ) ; list2 = new MyLinkedList ( ) ; list3 = new MyLinkedList () ; 1ist2.addFirst("6:Tony") list2.addFirst ("5 Tom" list2.addFirst ("4:Tim" list2.addFirst ("3: Abby") list2.addFirst ("2:Morning") list2.addFirst("1: Good") listo.addFirst (null); listo.addFirst ("bad") listo.addFirst ("apple") listo.addFirst (null); public static void drawLine) System.out.printin(" public static void testAddFirst) //passed test init ) drawLine) System.out.println (listo) System.out.println (list2) System.out.println (list3) drawLine ) public static void testAddLast) //passed System.out.printin(" init ) list3.add ("A"); System.out.printin (1ist3) list3.add ("B"): System.out.println (list3) list3. add (null); System.out.printin (1ist3) list3.add ("C"); System.out.println (list3) drawLine ) testAddLast ()-) public static void testAddIndex) //passed test init) System.out.println(" try testAddIndex)) list0.add (O, "AO") System.out.println (list0) isto . add (5, "A5"); System.out.println (list0) l d (3, "A3"); System.out.println (list0) 1ist3.add (O, "BO") System.out.println (list3) 1ist3.add (2, "B2") System.out.println (list3) isto.ad l catch (IndexOutofBoundsException e System.out.println (e) drawLine () public static void testRemoveIndex) //passed test init () System.out.printin(" try f testRemoveIndex)"); listo.remove (0): System.out.println (listo): listo.remove (2): System.out.println (listo): listo.remove (2): System.out.println (listo): l catch (IndexOutofBoundsException e System.out.println (e) listo.remove (1): System.out.println (listo): listo.remove (): System.out.println (listo): drawLine( public static void testGetIndex) // passed test init); System.out.println(" try i testGetIndex )) System.out.println(listo.get (0)) System.out.println (listo.get (1)) System.out.println (listo.get (2)) System.out.println (listo.get (3)) System.out.println (listo.get (4)) lcatch (IndexOutofBoundsException e) System.out.println(e) System.out.printin(list2.get (3)) drawLine ) public static void testContains //passed test init); Systemout . println ( " System.out.println (listo.contains (null) System.out.println (list2.contains ("1 :Good") System.out.println(list2.contains ("6:Tony")): System.out.println(list2.contains ("notexist")) System.out.println (list2.contains ("")) System.out.println (list2.contains (null) System.out.println(list3.contains ("notexist")) System.out.println(list3.contains (null)) drawLine ) testContains ) ); public static void testRemoveobject) //passed test init); System.out.println(" list2.remove ("1:Good"): System.out.println (list2.toString )) testRemove (Object)): ist2.remove ("notexist"); System.out.println (list2.toString )) list2. System.out.println (list2.toString )) list2. remove (null) System.out.println (list2.toString )) 1ist2.remove ("4:Tim") System.out.println (list2.toString )) System.out.printin (list0) listo. remove (null) System.out.printin (list0) listo. remove (null) System.out.printin (list0) list3.remove ("notexist" System.out.printin (list3) drawLine) remove("6:Tony") public static void testRemoveFirst init //passed System.out.println(" Object tmp null; try i testRemoveFirst ()-m); tmplist0.removeFirst ) System.out.printin (listo + ", removed:"tmp) tmplisto.removeFirst) System.out.println (listo ", removed:"tmp) tmp = listo. remove First () ; System.out.println (listo +", removed:" tmp); tmplisto.removeFirst () System.out.printin (listo + ", removed:"tmp) tmplisto.removeFirst) System. out.println(listo ", removed:" tmp); h catch (Exception e i System.out.println(e) : drawline)
Step by Step Solution
There are 3 Steps involved in it
Step: 1
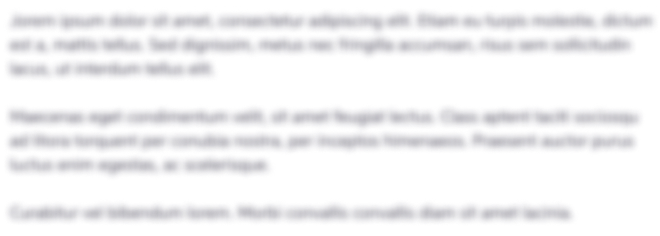
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started