For a school project, I have tocreate a Java BigInt class that will allow me to work with bigintegers, that is, integers with far more
For a school project, I have tocreate a Java BigInt class that will allow me to work with bigintegers, that is, integers with far more digits than Java's intdata type will allow. We were strongly encouraged to use anArrayList to implement this, basically inputting our desired 'BigInteger' as a string and having it parsed character by charcterinto the ArrayList as integers.
So far, I have created the constructor and toString methods,both of which work except for a couple of minor fringe cases, forexample recieving the string "+0" or "-0". The next phase isto be able to add, subtract,multiply, divide, and modulus any given two 'Big Integers.' I'mquite frankly completely stuck on this; I know conceptually that,for example in the 'add' method, it needs to accept two objects oftype BigInt, perform the desired operation (addition in this case)and store the results into a new ArrayList which is then returnedas the final answer. I'm getting hung up on the finer details ofimplementing this, such as: dealing with differing signs of theinput BigInts, dealing with the BigInts having different amounts ofdigits, and the actualy addition (ie, carrying over the 1 if twointegers sum to be greater than 9). Mostly, I'm not extraordinarilyfamiliar with ArrayLists so I'm having a hard time figuring out howto manipulate them to do what I need them to do.
I'm not asking for a totalsolution to this, just some help to point me in the right directionor perhaps at most a completely worked out example of the addmethod; I should be able to figure out everything else from there.Also, anything I can do to clean up what I already have would bevery helpful. Again, the instructor is expecting to see us useArrayLists for this project, so I need all assistance to be towardsusing that technique.
Below is what I have so far (notmuch beyond the constructor and toString Method) and the testdriver for the class. Any help is greatly appreciated!Thanks!
import java.util.*;
public class BigInt {
//Instance variables for the class, a booleanfor the sign and an ArrayList to store the input String
private boolean pos = true;
private ArrayList bigInt = newArrayList();
//No argument constructor, creates a biginteger of value zero
public BigInt () {
this.pos = true;
bigInt.add(0);
}
//Constructor for big integers input asstrings
public BigInt (String newBigInt) {
dealWithSign(newBigInt);
cleanUpNumber(newBigInt);
for(int i =newBigInt.length() + 1; i >=0; i--) {
bigInt.add(Integer.parseInt(newBigInt.substring(i,i+1)));
}
}
//Private method to deal with the sign of theincoming big integer
private void dealWithSign(String num) {
if(num.charAt(0) == '+' ||num.charAt(0) == '-') {
if(num.length() == 1) {
System.out.println("Invalid value: signonly, no integer.");
System.exit(0);
}
if(num.charAt(0) == '-') {
this.pos = false;
}
else{
this.pos = true;
}
num =num.substring(1);
}
}
//Private method to clean up the number;remove leading zeros, check for other errors, etc
private void cleanUpNumber(String num) {
if(num.charAt(0) == ' '){
System.out.println("Invalid value: leading blankspace.");
System.exit(0);
}
while(num.charAt(0) == '0'&& num.length() > 1) {
num =num.substring(1);
}
}
//Public method that performsaddition
public void add(BigInt one, BigInt two) {
BigInt result = newBigInt();
if (one.pos &&two.pos && one.bigInt.size() == two.bigInt.size()) {
for(inti=one.bigInt.size(); i >=0; i--) {
result.add(one.get(i) + two.get(i));
if (result.get(i) > 9) {
result.add(0);
result.add(i+1,result.get(i+1) = result.get(i+1) + 1);
}
}
}
result.toString();
}
//Public method that performssubtraction
public voidsubtract(BigInt one, BigInt two) {
}
//toString method
public String toString() {
String answer = "";
for(int i=0;i
answer= answer + bigInt.get(i);
}
if (!pos) {
answer= "-" + answer;
}
return answer;
}
}
import java.util.Scanner;public class BigInt_Add_Sub_Mul_Div_Mod_Demo{ public static void main(String[] args) { BigInt b1; BigInt b2; BigInt b3; b1 = new BigInt("-0"); b2 = new BigInt("+0"); b3 = b1.add(b2); System.out.println("1) sum b3 is " + b1 +" + " + b2 + " = " + b3); b3 = b1.subtract(b2); System.out.println("difference b3 is " + b1 +" - " + b2 + " = " + b3); b3 = b1.multiply(b2); System.out.println("product b3 is " + b1 +" * " + b2 + " = " + b3); b1 = new BigInt("1"); b2 = new BigInt("1"); b3 = b1.add(b2); System.out.println("2) sum b3 is " + b1 +" + " + b2 + " = " + b3); b3 = b1.subtract(b2); System.out.println("difference b3 is " + b1 +" - " + b2 + " = " + b3); b3 = b1.multiply(b2); System.out.println("product b3 is " + b1 +" * " + b2 + " = " + b3); b3 = b1.divideBy(b2); System.out.println("quotient b3 is " + b1 +" / " + b2 + " = " + b3); b3 = b1.modulus(b2); System.out.println("modulus b3 is " + b1 +" mod " + b2 + " = " + b3); b1 = new BigInt("-1"); b2 = new BigInt("1"); b3 = b1.add(b2); System.out.println("3) sum b3 is " + b1 +" + " + b2 + " = " + b3); b3 = b1.subtract(b2); System.out.println("difference b3 is " + b1 +" - " + b2 + " = " + b3); b3 = b1.multiply(b2); System.out.println("product b3 is " + b1 +" * " + b2 + " = " + b3); b3 = b1.divideBy(b2); System.out.println("quotient b3 is " + b1 +" / " + b2 + " = " + b3); b3 = b1.modulus(b2); System.out.println("modulus b3 is " + b1 +" mod " + b2 + " = " + b3); b1 = new BigInt("1"); b2 = new BigInt("-1"); b3 = b1.add(b2); System.out.println("4) sum b3 is " + b1 +" + " + b2 + " = " + b3); b3 = b1.subtract(b2); System.out.println("difference b3 is " + b1 +" - " + b2 + " = " + b3); b3 = b1.multiply(b2); System.out.println("product b3 is " + b1 +" * " + b2 + " = " + b3); b3 = b1.divideBy(b2); System.out.println("quotient b3 is " + b1 +" / " + b2 + " = " + b3); b3 = b1.modulus(b2); System.out.println("modulus b3 is " + b1 +" mod " + b2 + " = " + b3); b1 = new BigInt("-1"); b2 = new BigInt("-1"); b3 = b1.add(b2); System.out.println("5) sum b3 is " + b1 +" + " + b2 + " = " + b3); b3 = b1.subtract(b2); System.out.println("difference b3 is " + b1 +" - " + b2 + " = " + b3); b3 = b1.multiply(b2); System.out.println("product b3 is " + b1 +" * " + b2 + " = " + b3); b3 = b1.divideBy(b2); System.out.println("quotient b3 is " + b1 +" / " + b2 + " = " + b3); b3 = b1.modulus(b2); System.out.println("modulus b3 is " + b1 +" mod " + b2 + " = " + b3); b1 = new BigInt("+1"); b2 = new BigInt("+1"); b3 = b1.add(b2); System.out.println("6) sum b3 is " + b1 +" + " + b2 + " = " + b3); b3 = b1.subtract(b2); System.out.println("difference b3 is " + b1 +" - " + b2 + " = " + b3); b3 = b1.multiply(b2); System.out.println("product b3 is " + b1 +" * " + b2 + " = " + b3); b3 = b1.divideBy(b2); System.out.println("quotient b3 is " + b1 +" / " + b2 + " = " + b3); b3 = b1.modulus(b2); System.out.println("modulus b3 is " + b1 +" mod " + b2 + " = " + b3); b1 = new BigInt("-100"); b2 = new BigInt("100"); b3 = b1.add(b2); System.out.println("7) sum b3 is " + b1 +" + " + b2 + " = " + b3); b3 = b1.subtract(b2); System.out.println("difference b3 is " + b1 +" - " + b2 + " = " + b3); b3 = b1.multiply(b2); System.out.println("product b3 is " + b1 +" * " + b2 + " = " + b3); b3 = b1.divideBy(b2); System.out.println("quotient b3 is " + b1 +" / " + b2 + " = " + b3); b3 = b1.modulus(b2); System.out.println("modulus b3 is " + b1 +" mod " + b2 + " = " + b3); b1 = new BigInt("100"); b2 = new BigInt("-100"); b3 = b1.add(b2); System.out.println("8) sum b3 is " + b1 +" + " + b2 + " = " + b3); b3 = b1.subtract(b2); System.out.println("difference b3 is " + b1 +" - " + b2 + " = " + b3); b3 = b1.multiply(b2); System.out.println("product b3 is " + b1 +" * " + b2 + " = " + b3); b3 = b1.divideBy(b2); System.out.println("quotient b3 is " + b1 +" / " + b2 + " = " + b3); b3 = b1.modulus(b2); System.out.println("modulus b3 is " + b1 +" mod " + b2 + " = " + b3); b1 = new BigInt("-100"); b2 = new BigInt("-100"); b3 = b1.add(b2); System.out.println("9) sum b3 is " + b1 +" + " + b2 + " = " + b3); b3 = b1.subtract(b2); System.out.println("difference b3 is " + b1 +" - " + b2 + " = " + b3); b3 = b1.multiply(b2); System.out.println("product b3 is " + b1 +" * " + b2 + " = " + b3); b3 = b1.divideBy(b2); System.out.println("quotient b3 is " + b1 +" / " + b2 + " = " + b3); b3 = b1.modulus(b2); System.out.println("modulus b3 is " + b1 +" mod " + b2 + " = " + b3); b1 = new BigInt("100"); b2 = new BigInt("100"); b3 = b1.add(b2); System.out.println("10) sum b3 is " + b1 +" + " + b2 + " = " + b3); b3 = b1.subtract(b2); System.out.println("difference b3 is " + b1 +" - " + b2 + " = " + b3); b3 = b1.multiply(b2); System.out.println("product b3 is " + b1 +" * " + b2 + " = " + b3); b3 = b1.divideBy(b2); System.out.println("quotient b3 is " + b1 +" / " + b2 + " = " + b3); b3 = b1.modulus(b2); System.out.println("modulus b3 is " + b1 +" mod " + b2 + " = " + b3); b1 = new BigInt("200"); b2 = new BigInt("-0"); b3 = b1.add(b2); System.out.println("11) sum b3 is " + b1 +" + " + b2 + " = " + b3); b3 = b1.subtract(b2); System.out.println("difference b3 is " + b1 +" - " + b2 + " = " + b3); b3 = b1.multiply(b2); System.out.println("product b3 is " + b1 +" * " + b2 + " = " + b3); //b3 = b1.divideBy(b2); //System.out.println("quotient b3 is " + b1 +" / " + b2 + " = " + b3); //b3 = b1.modulus(b2); //System.out.println("modulus b3 is " + b1 +" mod " + b2 + " = " + b3); b1 = new BigInt("-200"); b2 = new BigInt("-0"); b3 = b1.add(b2); System.out.println("12) sum b3 is " + b1 +" + " + b2 + " = " + b3); b3 = b1.subtract(b2); System.out.println("difference b3 is " + b1 +" - " + b2 + " = " + b3); b3 = b1.multiply(b2); System.out.println("product b3 is " + b1 +" * " + b2 + " = " + b3); //b3 = b1.divideBy(b2); //System.out.println("quotient b3 is " + b1 +" / " + b2 + " = " + b3); //b3 = b1.modulus(b2); //System.out.println("modulus b3 is " + b1 +" mod " + b2 + " = " + b3); b1 = new BigInt("-0"); b2 = new BigInt("200"); b3 = b1.add(b2); System.out.println("13) sum b3 is " + b1 +" + " + b2 + " = " + b3); b3 = b1.subtract(b2); System.out.println("difference b3 is " + b1 +" - " + b2 + " = " + b3); b3 = b1.multiply(b2); System.out.println("product b3 is " + b1 +" * " + b2 + " = " + b3); b3 = b1.divideBy(b2); System.out.println("quotient b3 is " + b1 +" / " + b2 + " = " + b3); b3 = b1.modulus(b2); System.out.println("modulus b3 is " + b1 +" mod " + b2 + " = " + b3); b1 = new BigInt("-0"); b2 = new BigInt("-200"); b3 = b1.add(b2); System.out.println("14) sum b3 is " + b1 +" + " + b2 + " = " + b3); b3 = b1.subtract(b2); System.out.println("difference b3 is " + b1 +" - " + b2 + " = " + b3); b3 = b1.multiply(b2); System.out.println("product b3 is " + b1 +" * " + b2 + " = " + b3); b3 = b1.divideBy(b2); System.out.println("quotient b3 is " + b1 +" / " + b2 + " = " + b3); b3 = b1.modulus(b2); System.out.println("modulus b3 is " + b1 +" mod " + b2 + " = " + b3); b1 = new BigInt("37"); b2 = new BigInt("26"); b3 = b1.add(b2); System.out.println("15) sum b3 is " + b1 +" + " + b2 + " = " + b3); b3 = b1.subtract(b2); System.out.println("difference b3 is " + b1 +" - " + b2 + " = " + b3); b3 = b1.multiply(b2); System.out.println("product b3 is " + b1 +" * " + b2 + " = " + b3); b3 = b1.divideBy(b2); System.out.println("quotient b3 is " + b1 +" / " + b2 + " = " + b3); b3 = b1.modulus(b2); System.out.println("modulus b3 is " + b1 +" mod " + b2 + " = " + b3); b1 = new BigInt("-37"); b2 = new BigInt("26"); b3 = b1.add(b2); System.out.println("16) sum b3 is " + b1 +" + " + b2 + " = " + b3); b3 = b1.subtract(b2); System.out.println("difference b3 is " + b1 +" - " + b2 + " = " + b3); b3 = b1.multiply(b2); System.out.println("product b3 is " + b1 +" * " + b2 + " = " + b3); b3 = b1.divideBy(b2); System.out.println("quotient b3 is " + b1 +" / " + b2 + " = " + b3); b3 = b1.modulus(b2); System.out.println("modulus b3 is " + b1 +" mod " + b2 + " = " + b3); b1 = new BigInt("37"); b2 = new BigInt("-26"); b3 = b1.add(b2); System.out.println("17) sum b3 is " + b1 +" + " + b2 + " = " + b3); b3 = b1.subtract(b2); System.out.println("difference b3 is " + b1 +" - " + b2 + " = " + b3); b3 = b1.multiply(b2); System.out.println("product b3 is " + b1 +" * " + b2 + " = " + b3); b3 = b1.divideBy(b2); System.out.println("quotient b3 is " + b1 +" / " + b2 + " = " + b3); b3 = b1.modulus(b2); System.out.println("modulus b3 is " + b1 +" mod " + b2 + " = " + b3); b1 = new BigInt("-37"); b2 = new BigInt("-26"); b3 = b1.add(b2); System.out.println("18) sum b3 is " + b1 +" + " + b2 + " = " + b3); b3 = b1.subtract(b2); System.out.println("difference b3 is " + b1 +" - " + b2 + " = " + b3); b3 = b1.multiply(b2); System.out.println("product b3 is " + b1 +" * " + b2 + " = " + b3); b3 = b1.divideBy(b2); System.out.println("quotient b3 is " + b1 +" / " + b2 + " = " + b3); b3 = b1.modulus(b2); System.out.println("modulus b3 is " + b1 +" mod " + b2 + " = " + b3); b1 = new BigInt("-200111111111111111199999999"); b2 = new BigInt("3333333333333388888888888888888888555555555555555555555555"); b3 = b1.add(b2); System.out.println("19) sum b3 is " + b1 +" + " + b2 + " = " + b3); b3 = b1.subtract(b2); System.out.println("difference b3 is " + b1 +" - " + b2 + " = " + b3); b3 = b1.multiply(b2); System.out.println("product b3 is " + b1 +" * " + b2 + " = " + b3); b3 = b1.divideBy(b2); System.out.println("quotient b3 is " + b1 +" / " + b2 + " = " + b3); b3 = b1.modulus(b2); System.out.println("modulus b3 is " + b1 +" mod " + b2 + " = " + b3); b1 = new BigInt("66666666666666666666677777777777777777777711111111111111111200"); b2 = new BigInt("-3333333333333333333344444444444"); b3 = b1.add(b2); System.out.println("20) sum b3 is " + b1 +" + " + b2 + " = " + b3); b3 = b1.subtract(b2); System.out.println("difference b3 is " + b1 +" - " + b2 + " = " + b3); b3 = b1.multiply(b2); System.out.println("product b3 is " + b1 +" * " + b2 + " = " + b3); b3 = b1.divideBy(b2); System.out.println("quotient b3 is " + b1 +" / " + b2 + " = " + b3); b3 = b1.modulus(b2); System.out.println("modulus b3 is " + b1 +" mod " + b2 + " = " + b3); b1 = new BigInt("-66666666666666666666677777777777777777777711111111111111111200"); b2 = new BigInt("-333333"); b3 = b1.add(b2); System.out.println("21) sum b3 is " + b1 +" + " + b2 + " = " + b3); b3 = b1.subtract(b2); System.out.println("difference b3 is " + b1 +" - " + b2 + " = " + b3); b3 = b1.multiply(b2); System.out.println("product b3 is " + b1 +" * " + b2 + " = " + b3); b3 = b1.divideBy(b2); System.out.println("quotient b3 is " + b1 +" / " + b2 + " = " + b3); b3 = b1.modulus(b2); System.out.println("modulus b3 is " + b1 +" mod " + b2 + " = " + b3); b1 = new BigInt("6666666"); b2 = new BigInt("3333333333333333333344444444444444444444455555555555555550"); b3 = b1.add(b2); System.out.println("22) sum b3 is " + b1 +" + " + b2 + " = " + b3); b3 = b1.subtract(b2); System.out.println("difference b3 is " + b1 +" - " + b2 + " = " + b3); b3 = b1.multiply(b2); System.out.println("product b3 is " + b1 +" * " + b2 + " = " + b3); b3 = b1.divideBy(b2); System.out.println("quotient b3 is " + b1 +" / " + b2 + " = " + b3); b3 = b1.modulus(b2); System.out.println("modulus b3 is " + b1 +" mod " + b2 + " = " + b3); b1 = new BigInt("1111111111111111111111111111111122222222222222222222222222222222222333333333333333333333333333333334444444444444444444444444"); b2 = new BigInt("99999999999999999999999999999888888888888888888888888888888881111111111111111111111111111111122222222222222222222222222222222222333333333333333333333333333333334444444444444444444444444"); b3 = b1.add(b2); System.out.println("23) sum b3 is " + b1 +" + " + b2 + " = " + b3); b3 = b1.subtract(b2); System.out.println("difference b3 is " + b1 +" - " + b2 + " = " + b3); b3 = b1.multiply(b2); System.out.println("product b3 is " + b1 +" * " + b2 + " = " + b3); b3 = b1.divideBy(b2); System.out.println("quotient b3 is " + b1 +" / " + b2 + " = " + b3); b3 = b1.modulus(b2); System.out.println("modulus b3 is " + b1 +" mod " + b2 + " = " + b3); b1 = new BigInt("-1111111111111111111111111111111122222222222222222222222222222222222333333333333333333333333333333334444444444444444444444444"); b2 = new BigInt("99999999999999999999999999999888888888888888888888888888888881111111111111111111111111111111122222222222222222222222222222222222333333333333333333333333333333334444444444444444444444444"); b3 = b1.add(b2); System.out.println("24) sum b3 is " + b1 +" + " + b2 + " = " + b3); b3 = b1.subtract(b2); System.out.println("difference b3 is " + b1 +" - " + b2 + " = " + b3); b3 = b1.multiply(b2); System.out.println("product b3 is " + b1 +" * " + b2 + " = " + b3); b3 = b1.divideBy(b2); System.out.println("quotient b3 is " + b1 +" / " + b2 + " = " + b3); b3 = b1.modulus(b2); System.out.println("modulus b3 is " + b1 +" mod " + b2 + " = " + b3); b1 = new BigInt("1111111111111111111111111111111122222222222222222222222222222222222333333333333333333333333333333334444444444444444444444444"); b2 = new BigInt("-99999999999999999999999999999888888888888888888888888888888881111111111111111111111111111111122222222222222222222222222222222222333333333333333333333333333333334444444444444444444444444"); b3 = b1.add(b2); System.out.println("25) sum b3 is " + b1 +" + " + b2 + " = " + b3); b3 = b1.subtract(b2); System.out.println("difference b3 is " + b1 +" - " + b2 + " = " + b3); b3 = b1.multiply(b2); System.out.println("product b3 is " + b1 +" * " + b2 + " = " + b3); b3 = b1.divideBy(b2); System.out.println("quotient b3 is " + b1 +" / " + b2 + " = " + b3); b3 = b1.modulus(b2); System.out.println("modulus b3 is " + b1 +" mod " + b2 + " = " + b3); b1 = new BigInt("-1111111111111111111111111111111122222222222222222222222222222222222333333333333333333333333333333334444444444444444444444444"); b2 = new BigInt("-99999999999999999999999999999888888888888888888888888888888881111111111111111111111111111111122222222222222222222222222222222222333333333333333333333333333333334444444444444444444444444"); b3 = b1.add(b2); System.out.println("26) sum b3 is " + b1 +" + " + b2 + " = " + b3); b3 = b1.subtract(b2); System.out.println("difference b3 is " + b1 +" - " + b2 + " = " + b3); b3 = b1.multiply(b2); System.out.println("product b3 is " + b1 +" * " + b2 + " = " + b3); b3 = b1.divideBy(b2); System.out.println("quotient b3 is " + b1 +" / " + b2 + " = " + b3); b3 = b1.modulus(b2); System.out.println("modulus b3 is " + b1 +" mod " + b2 + " = " + b3); }}
Step by Step Solution
3.40 Rating (159 Votes )
There are 3 Steps involved in it
Step: 1
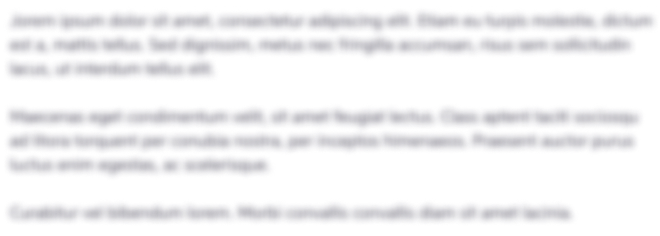
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started