Question
For C++: The tic-tac-toe game you are to write is console based. Which means you will have to type in a row, column pair to
For C++:
The tic-tac-toe game you are to write is console based. Which means you will have to type in a row, column pair to place the X or the O.
For this game to work you are going to have to write four functions. Here is the list of functions that need to be written:
createGameBoard - Takes as an argument the 2D array that represents the tic-tac-toe board
printBoard - Prints the tic-tac-toe board out to the screen
markSpace -Takes as arguments the tic-tac-toe board, the row index, and the column index. This function marks either an X or an O in the appropriate space.
gameOver - This function checks to see if the game is over and if there is a winner. It takes as arguments the 2D array that represents the game board and a char by reference to hold the winner. This function also returns a bool.
The above list really didn't give much detail so it may be best to supply some direction for each function:
void createGameBoard(char bd[][3]) - This function should fill the tic-tac-toe game array (called bd here) with the dash '-' character
void printBoard(char bd[][3]) - This function should print the tic-tac-toe game array (called bd here) to the screen in row column fashion (3 rows by 3 columns)
bool markSpace(char bd[][3], int row, int col) - Here are the steps to complete the task:
You need to create a static bool called xTurn and set it initially to false.
Set xTurn to not xTurn.
Check to see if what is at row col location is a dash '-' character.
If it is a dash and it is xTurn then mark the space with and 'X' character
Else mark it with an 'O' character
Clear the screen using system("CLS") Note: This will not work on a Mac
return true.
Note that 3,4, and 5 should be within the if statement described in step 3.
In the case that the character at the row, col location is not a dash character then you simply return false. This indicates that the space is already occupied with either an 'X' or an 'O'
bool gameOver(char game[][3], char &whoWon) - This is the most complex function. It determines if the game has been won or not. Here are the steps:
Create a char variable called winner and set it equal to a space character ' '
Check the first diagonal squares. If the game[0][0] is not equal to the dash character and game[0][0] is equal to game[1][1] and game[2][2] then set winner equal to game[0][0]
Check the second diagonal square. Else if game[2][0] is not equal to the dash character and game[2][0] is equal to game[1][1] and game[0][2] then set winner equal to game[2][0]
Else check the rows and columns. Create a for loop that will loop through all of the rows in the game board. Create a variable called row for your loop counter. Inside the for loop we first want to check each row. Here is how you do it:
If game[row][0] is not equal to the dash character and game[row][0] is equal to game[row][1] and game[row][2] then set winner = game[row][0] and break out of the loop using a break statement
Next we use an else if to check each column. Here is how you do it:
else if (game[0][row] is not equal to the dash character and game[0][row] is equal to game[1][row] and game[2][row] then set winner equal to game[0][row] and break out of the loop
Finally we need to return the winner. You will need an if else statement as the last thing in the function. It should not be nested within any other loop or if statement. Here is how you do it:
if winner is equal to a space character ' ' then simply return false. Else, set whoWon equal to winner and return true.
The main function - The main function is where the main game loop goes. Here are the steps to make it happen:
Create the following variables
A 3 x 3 array of chars called board
A bool variable called finished initialized to false
Two int variables called x and y
An int called count initialized to 0. This is used to keep count of the number of turns
A character variable called winner and set to the space character.
Before we start the game loop a couple of things need to be done
Create the game board by calling the function and passing it the 2D array called board
Print the board to the screen by calling the printBoard function passing it the board array
Next we start the game loop this is a while loop that will loop until finished is equal to false. Here is what is inside the while loop:
Ask for a position to place the next character
use cin and store the position in the x and y variables
Mark the space by passing the board and the x y positions to the appropriate function
Increment the count variable by 1
Check to see if the game is over by passing the board and the winner variable to the gameOver function. Store the return value from the function in the variable called finished
Check to see if the variable finished is true and that count is less than 9. If so the winner is stored in the winner variable. Output who won to the screen.
Else if finished is equal to false and the count is equal to 9 then you have a tie game. Report it to the screen and break out of the loop.
At this point you should have a working Tic-Tac-Toe game.
NOTE and Required: No error checking is built into this game. This means that if a person enters a position that is greater than two the game will crash. It is up to you to put in some kind of mechanism that insures the proper range is input by the user.
What not to do
Using any of the following will drop your grade for this assignment by 70%
global variables
cin in any function other than main
cout in any functions other than printBoard
goto statements
Your program should run like the following:
Step by Step Solution
There are 3 Steps involved in it
Step: 1
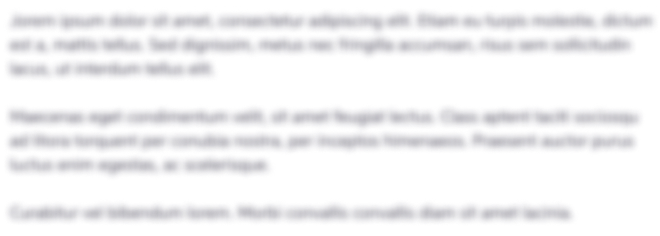
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started