Question
For each problem, study the supplied code, and list the code smells you see. For this exercise the code smells should be one of these:
For each problem, study the supplied code, and list the code smells you see. For this exercise the code smells should be one of these:
- long method,
- large class,
- duplicate code (aka cut and paste code),
- long parameter list,
- primitive obsession, and
- magic numbers.
For each code smell,
- list the line numbers of the code where the smell is focused,
- identify the design principle(s) that are violated, and
- identify some program change that the smell would complicate.
-
public class database {
public static List studentTable;
public static List facultyTable;
public static List groupTable;
public static List majorTable;
public static List dormTable;
public static List studentOrgsTable;
public static List parkingLotTable;
public static List employerTable;
public static DiningCenter seasons;
public static DiningCenter conversations;
public static DiningCenter udcc;
public static DiningCenter windows;
public static List busTable;
public static List busRoutetable;
public static List fairTable;
public static List intramuralSportTable;
public static List gymequip;
public static List gymrent;
public Calendar calendar;
public database() throws FileNotFoundException {
......
}
/**
* Adds fair to to table
* @param fair
*/
public static void addFair(Fair fair){
.....
}
/**
* Returns fair based on building and date
* @param building
* @param date
* @return Fair
*/
public static Fair getFair(String building, Date date){
......
}
/**
* Removes fair from fair table
* @param fair
*/
public static void removeFair(Fair fair){
......
}
/**
* Adds student to table
* @param s
*/
public static void addStudent(student s) {
.....
}
/**
* Adds bus to table
* @param bus
*/
public static void addBus(Bus bus){
.....
}
/**
* Removes bus from table
* @param bus
*/
public static void removeBus(Bus bus){
.....
}
/**
* Adds bus route
* @param route
*/
public static void addBusRoute(BusRoute route){
.....
}
-
/**
* Removes Bus Route
* @param route
*/
public static void removeBusRoute(BusRoute route){
.....
}
/**
* Gets busroute based on name
* @param routeName
* @return BusRoute
*/
public BusRoute getBusRoute(String routeName){
.....
}
/**
* Gets bus based on number
* @param busNumber
* @return Bus
*/
public Bus getBus(int busNumber){
.....
}
/**
* Adds lot to table
* @param l
*/
public static void addLot(Lot l){
.....
}
/**
* Finds a lot
* @param id
* @return Lot
*/
public static Lot findLot(String id){
.....
}
/**
* Removes lot
* @param l
*/
public static void removeLot(Lot l){
.....
}
/**
* Adds gym equipment
* @param addnew
* @return boolean
*/
public static boolean addGymEquipment(GymEquipment addnew){
.....
}
/**
* Finds gym equipment and returns it
* @param idname
* @return GymEquipment
*/
public static GymEquipment findGymEquipment(String idname){
.....
}
/**
* Removes gym equipment
* @param idname
* @return
*/
public static boolean removeGymEquipment(String idname){
.....
}
public String deleteGymItem(String id){
.....
}
-
Smells and locations?
Design principles?
Maintenance Consequences?
Step by Step Solution
There are 3 Steps involved in it
Step: 1
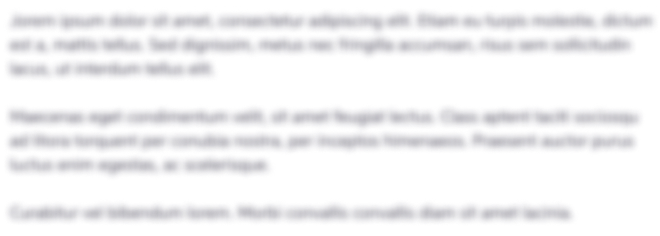
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started