Question
FOR JAVA: Create a Swing application that looks and behaves like the example located at the top of this assignment. Start with your code from
FOR JAVA:
Create a Swing application that looks and behaves like the example located at the top of this assignment. Start with your code from week one and implement the use of the Oracle database in place of the array used in week one.
- Modify your CLASSPATH environment variable to include both the Oracle thin drivers .jar file and the current Java working directory. The attached file gives you more info on CLASSPATH.
- Load the Oracle Thin Drivers into the Java/lib folder.
- Take a look at the examples on this page for the sql to populate your database, run this before you try to access the database.
- Take a look at the sample Oracle_select3 java code, this is a good starting place.
- Create a connection to the Oracle database.
- Execute a query of the database to retrieve a populated ResultSet.
- Then with the populated ResultSet:
- The Previous button will iterate through the ResultSet moving to the previous element each time the button is clicked and will then update the GUI with the newly selected data. If the Previous button is selected while the ResultSet is positioned at the first element, your program should then move the last element and update the display with the newly selected data.
- The Next button will iterate through the ResultSet moving to the next element each time the button is clicked and will then update the GUI with the newly selected data. If the Next button is selected while the ResultSet is positioned at the last element, your program should then move the first element and update the display with the newly selected data.
- When the Reset button is selected you should move to the first element in the ResultSet and update the display.
-----------------------------------------------------------
Table Data for this Assignment:
DROP TABLE address;
CREATE TABLE address( id NUMBER (4) CONSTRAINT pk_address PRIMARY KEY, LASTNAME varchar2(40), FIRSTNAME varchar2(40), STREET varchar2(40), CITY varchar2(40), STATE varchar2(40), ZIP varchar2(40) );
INSERT INTO address VALUES(1,'Smith','Tom','2919 Redwing Circle','Bellevue','NE','68123');
INSERT INTO address VALUES(2,'Actor','Marty','1000 Galvin Road South','Bellevue','NE','68005');
INSERT INTO address VALUES(3,'Aimes','Beverly','1 Park Place','Redmond','Washington','90922');
COMMIT;
-----------------------------------------------------
Code from Week 1:
import java.awt.event.ActionListener; import javax.swing.*;
import java.awt.*; import static javax.swing.WindowConstants.DO_NOTHING_ON_CLOSE;
class Assignment_02Source extends JFrame
{
private JButton buttonPrev = new JButton("Prev");
private JButton buttonReset = new JButton("Reset");
private JButton buttonNext = new JButton("Next");
private JLabel labelHeader = new JLabel("Database Browser",JLabel.CENTER);
private JLabel labelName = new JLabel("Name");
private JLabel labelAddress = new JLabel("Address");
private JLabel labelCity = new JLabel("City");
private JLabel labelState = new JLabel("State");
private JLabel labelZip = new JLabel("Zip");
private JTextField textFieldName = new JTextField();
private JTextField textFieldAddress = new JTextField();
private JTextField textFieldCity = new JTextField();
private JTextField textFieldState = new JTextField();
private JTextField textFieldZip = new JTextField();
DataClass[] DataClassArray = {
new DataClass("Fred", "Wayne", "101 Here", "NE", "55551"),
new DataClass("Goerge", "Thomas", "102 There", "ME", "55552"),
new DataClass("Mike", "Johnson", "103 No Where", "OK", "55553")
};
int arrayPointer = 0;
public Assignment_02Source (String title)
{
super(title);
setDefaultCloseOperation(DO_NOTHING_ON_CLOSE);
JPanel cp = (JPanel) getContentPane( ) ; //labelHeader.setFont(new Font("CourierNew", Font.italic, 12));
labelHeader.setBounds(40, 10, 300, 50);
buttonPrev.setBounds(30, 250, 80, 25);
buttonReset.setBounds(150, 250, 80, 25);
buttonNext.setBounds(270, 250, 80, 25);
labelName.setBounds(10, 80, 80, 25);
labelAddress.setBounds(10, 110, 80, 25);
labelCity.setBounds(10, 140, 80, 25);
labelState.setBounds(10, 170, 80, 25);
labelZip.setBounds(10, 200, 80, 25);
textFieldName.setBounds(120, 80, 250, 25);
textFieldAddress.setBounds(120, 110, 250, 25);
textFieldCity.setBounds(120, 140, 250, 25);
textFieldState.setBounds(120, 170, 250, 25);
textFieldZip.setBounds(120, 200, 250, 25);
cp.setLayout(null);
cp.add(labelHeader);
cp.add(buttonPrev);
cp.add(buttonReset);
cp.add(buttonNext);
cp.add(labelName);
cp.add(textFieldName);
cp.add(labelAddress);
cp.add(textFieldAddress);
cp.add(labelCity);
cp.add(textFieldCity);
cp.add(labelState);
cp.add(textFieldState);
cp.add(labelZip);
cp.add(textFieldZip);
addWindowListener(new java.awt.event.WindowAdapter( )
{ //overrides methods from: java.awt.event.WindowEvent @Override public void windowClosing(java.awt.event.WindowEvent evt)
{
shutDown( );
}
});
//replaced event with ActionListenerImplement which implements ActionListener buttonPrev.addActionListener(new ActionListenerImplement());
buttonNext.addActionListener(new java.awt.event.ActionListener()
{
public void actionPerformed(java.awt.event.ActionEvent evt)
{
if (++arrayPointer == DataClassArray.length)
arrayPointer = 0;
setFields(arrayPointer);
}
});
buttonReset.addActionListener(new java.awt.event.ActionListener()
{
//implements methods from java.awt.event.ActionEvent @Override public void actionPerformed(java.awt.event.ActionEvent evt)
{
arrayPointer = 0;
setFields(arrayPointer); }
});
setFields(arrayPointer);
}
private void setFields(int position)
{
textFieldName.setText(DataClassArray[position].getName( ));
textFieldAddress.setText(DataClassArray[position].getAddress( ));
textFieldCity.setText(DataClassArray[position].getCity( ));
textFieldState.setText(DataClassArray[position].getState( ));
textFieldZip.setText(DataClassArray[position].getZip( ));
}
private void shutDown( )
{
int returnVal = JOptionPane.showConfirmDialog(this,"Are you you want to EXIT?");
if (returnVal == JOptionPane.YES_OPTION)
{
System.exit(0);
}
} public static void main(String args[ ])
{ Assignment_02Source a2= new Assignment_02Source ("Database Browser");
a2.setSize(400, 350);
a2.setVisible(true);
}
private class ActionListenerImplement implements ActionListener {
public ActionListenerImplement() { }
//implements method from java.awt.event.ActionEvent @Override public void actionPerformed(java.awt.event.ActionEvent evt) { if (--arrayPointer < 0) { arrayPointer = DataClassArray.length - 1; } setFields(arrayPointer); } }
}
// DataClass
class DataClass
{ // To save space I declared all String objects on a single line
String name, address, city, state, zipCode;
DataClass(String name, String address, String city, String state,
String zipCode)
{
this.name = name;
this.address = address;
this.city = city;
this.state = state;
this.zipCode = zipCode;
}
String getName( )
{ return this.name; } String getAddress( ) { return this.address; } String getCity( ) { return this.city;
} String getState( ) { return this.state; } String getZip()
{
return this.zipCode;
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
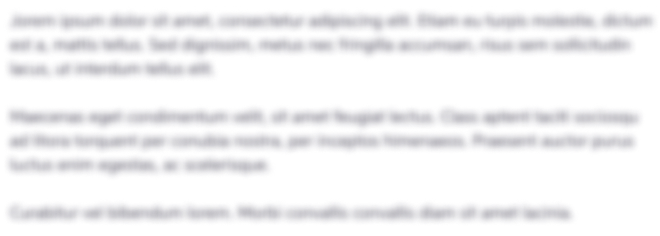
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started