Question
For Java While writing your answer to question 1 make use of BookMain to test your solutions to the various parts of the question. This
For Java
While writing your answer to question 1 make use of BookMain to test your solutions to the various parts of the question. This class wont be marked. You will also use the Book and BookCollection classes in this question. 1. Complete the Book class. It should have the following private properties. You class should include a constructor with arguments for each of these properties. Also create getters and setters for each property title (string) author (String) ISBN (long) pages (int) copiesAvailable (int) copiesOnLoan (int) [2 marks] 2. Complete the BookCollection constructor so that it loads the file path argument as a File. Then use a Scanner to read in the file and populate the books arrayList. When complete books should have 100 items in it. Hint: You will need to skip over the first line of the file (the column headers), otherwise you will get an error. [6 marks] 3. Complete the function getAuthors so that it returns a HashSet of all the authors in books. [5 marks] 4. Complete the function getLongBooks so it returns an ArrayList of Books which have over 750 pages in them [5 marks] 5. Complete the function getBookByTitle so it returns the Book object for the given title. If a title not in the list is given return null. [5 marks] 6. Complete the function getMostPopularBooks which returns an array of the 10 most popular books (That is those that currently have most copies on loan). [7 marks] ------------------------------------------------------------------------------------------------------------------------------------
public class Book {
private String title;
private String author;
private long isbn;
private int pages;
private int copiesInCollection;
private int CopiesOnLoan;
//1, complete this class with a constructor that has arguments for all the properties
//and, getters and setters for each of them
}
------------------------------------------------------------------------------------------------------------------------------------
import java.io.File;
import java.io.FileNotFoundException;
import java.util.HashSet;
import java.util.Scanner;
import java.util.ArrayList;
public class BookCollection {
private ArrayList
//2, complete constructor that takes a string path (the BookList file name) load the books from BookList into the books arrayList
//When complete books should have 100 items. Make sure you don't include the header row!
BookCollection(String path) {
}
//3, Return a HashSet of all the authors in the book list
public HashSet
}
//4, return an arrayList of books with more than 750 pages
public ArrayList
}
//5, return the book if the given title is in the list.
public Book getBookByTitle(String title){
}
//6, return an array of the 10 most popular books (That is those that currently have most copies on loan)
public Book[] mostPopular(){
}
}
------------------------------------------------------------------------------------------------------------------------------------
public class BookMain {
public static void main(String[] args) {
BookCollection bCollection = new BookCollection("BookList.csv");
}
} ------------------------------------------------------------------------------------------------------------------------------------ BookList.csv
Step by Step Solution
There are 3 Steps involved in it
Step: 1
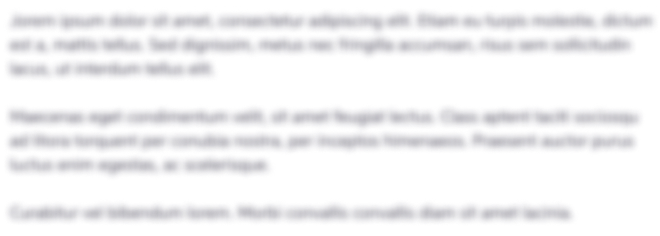
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started